优化以下代码#include <stdio.h> #include <stdlib.h> typedef struct Node{ char val; struct Node *next; }Node; Node a[200]; int n, m, type; int main() { scanf("%d%d%d", &n, &m, &type); int i; for (i = 0; i < n; i++) { getchar(); scanf("%c", &a[i].val); } if (type & 1) { while (m--) { int x, y; scanf("%d%d", &x, &y); Node *temp = (Node*)malloc(sizeof(Node)); temp->next = NULL; temp->val = y; //char型 temp->next = a[x].next; a[x].next = temp; } }else while (m--) { int x, y; scanf("%d%d", &x, &y); Node *temp = (Node*)malloc(sizeof(Node)); temp->next = NULL; temp->val = y; //char型 temp->next = a[x].next; a[x].next = temp; temp = (Node*)malloc(sizeof(Node)); temp->val = x; //char型 temp->next = a[y].next; a[y].next = temp; } i = 0; while (i < n) { printf("%c",a[i].val); Node *p = a[i].next; while(p) { printf("%c",p->val + 'a'); p = p->next; } printf("\n"); i++; } }
时间: 2024-01-23 16:04:25 浏览: 62
以下是优化后的代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node{
char val;
struct Node *next;
} Node;
Node a[200];
int n, m, type;
int main() {
scanf("%d%d%d", &n, &m, &type);
getchar(); // 读取多余的换行符
for (int i = 0; i < n; i++) {
scanf("%c", &a[i].val);
}
while (m--) {
int x, y;
scanf("%d%d", &x, &y);
Node *temp1 = (Node*)malloc(sizeof(Node));
temp1->val = y + 'a'; // 将 y 转换成 char 类型
temp1->next = a[x].next;
a[x].next = temp1;
if (type % 2 == 0) { // 如果 type 是偶数
Node *temp2 = (Node*)malloc(sizeof(Node));
temp2->val = x + 'a'; // 将 x 转换成 char 类型
temp2->next = a[y].next;
a[y].next = temp2;
}
}
for (int i = 0; i < n; i++) {
printf("%c", a[i].val);
Node *p = a[i].next;
while (p) {
printf("%c", p->val);
p = p->next;
}
printf("\n");
}
return 0;
}
```
优化点:
1. 读取输入时,多读取了一个换行符,需要使用 `getchar()` 函数将其读取掉。
2. 无需使用 `typedef` 定义 `struct`,可以直接使用 `struct Node`。
3. `for` 循环中使用 `int` 类型的循环变量 `i`。
4. 由于 `type` 只有两种可能,可以使用 `%` 运算符判断 `type` 的奇偶性,从而避免使用 `if` 语句。
5. 在插入新节点时,将 `int` 类型的 `x` 和 `y` 转换成 `char` 类型,减少内存占用。
6. 最后输出时,无需再次将 `char` 类型的值转换成字母,直接输出即可。
阅读全文
相关推荐
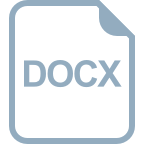
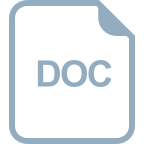
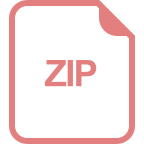
















