写一段代码基于AT90USB1286的bootloader功能实现重启程序时读取代码并写入app区
时间: 2024-02-16 20:03:12 浏览: 18
对于AT90USB1286的bootloader功能,通常使用avr-libc库提供的bootloader模板进行开发。下面是一个基于模板的重启程序时读取代码并写入app区的示例代码:
```c
#include <avr/io.h>
#include <avr/boot.h>
#include <avr/interrupt.h>
#define APP_SECTION_START 0x1800
#define APP_SECTION_SIZE (32 * 1024)
#define APP_PAGE_SIZE SPM_PAGESIZE
void app_start(void) __attribute__ ((noreturn)) {
void (*start)(void) = (void *) APP_SECTION_START;
start();
}
int main(void) {
// Initialize UART and other peripherals as needed
// ...
// Check if the application section is valid
uint8_t *app_ptr = (uint8_t *) APP_SECTION_START;
if (*app_ptr == 0xff) {
// Application section is blank, stay in bootloader
while (1) {
// Wait for commands from the host
// ...
}
}
// If the reset was caused by a watchdog timeout, clear the watchdog flag
if (MCUSR & (1 << WDRF)) {
MCUSR &= ~(1 << WDRF);
}
// Jump to the application section
app_start();
}
void erase_app(void) {
// Erase the entire application section
for (uint32_t addr = APP_SECTION_START; addr < APP_SECTION_START + APP_SECTION_SIZE; addr += APP_PAGE_SIZE) {
boot_page_erase(addr);
boot_spm_busy_wait();
}
}
void write_app(uint8_t *data, uint32_t len) {
uint32_t addr = APP_SECTION_START;
uint32_t end_addr = APP_SECTION_START + APP_SECTION_SIZE;
uint8_t *ptr = data;
while (addr < end_addr && ptr < data + len) {
// Write one page at a time
boot_page_erase(addr);
boot_spm_busy_wait();
for (uint16_t i = 0; i < APP_PAGE_SIZE && ptr < data + len; i += 2) {
uint16_t word = *ptr++;
word |= (*ptr++) << 8;
boot_page_fill(addr + i, word);
}
boot_page_write(addr);
boot_spm_busy_wait();
addr += APP_PAGE_SIZE;
}
}
void restart_app(void) {
// Disable interrupts
cli();
// Clear the reset vector
boot_page_erase(0);
boot_spm_busy_wait();
// Set the reset vector to the start of the application section
boot_page_fill(0, APP_SECTION_START >> 1);
boot_page_write(0);
boot_spm_busy_wait();
// Enable interrupts
sei();
// Jump to the reset vector
asm("jmp 0");
}
void handle_command(uint8_t cmd, uint8_t *data, uint16_t len) {
switch (cmd) {
case 'E': // Erase application section
erase_app();
// Send acknowledgement
// ...
break;
case 'W': // Write data to application section
write_app(data, len);
// Send acknowledgement
// ...
break;
case 'R': // Restart application
restart_app();
break;
// Handle other commands as needed
// ...
}
}
void receive_command(void) {
uint8_t cmd;
uint16_t len;
uint8_t data[APP_PAGE_SIZE];
// Receive command from UART
// ...
handle_command(cmd, data, len);
}
```
在这个示例中,bootloader首先检查应用程序区域是否为空。如果为空,则停留在bootloader中等待命令。如果不是空的,则检查是否由看门狗超时引起重启,并清除看门狗标志。然后,bootloader跳转到应用程序区域。
bootloader支持三个命令:擦除应用程序区域(E),将数据写入应用程序区域(W)和重启应用程序(R)。在收到命令后,bootloader会执行相应的操作。写入数据时,bootloader将数据写入应用程序区域的一页,然后将其擦除并写入下一页,直到所有数据都被写入。重启应用程序时,bootloader将重置向量设置为应用程序区域的起始地址,然后跳转到重置向量。
请注意,这只是一个示例代码,需要根据具体的需求进行修改和完善。特别是,需要实现与主机通信的UART接口和命令协议。
相关推荐
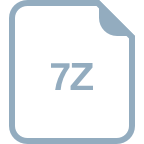













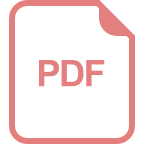