pandas取前十行的值并化为list
时间: 2023-08-15 19:54:43 浏览: 89
要将 Pandas DataFrame 的前十行的值转换为一个列表,可以使用 `head()` 方法来获取前十行,然后使用 `values.tolist()` 方法将其转换为列表。下面是一个示例代码:
```python
import pandas as pd
# 创建示例数据
data = {'A': [1, 2, 3, 4, 5, 6, 7, 8, 9, 10], 'B': ['foo', 'bar', 'baz', 'qux', 'quux', 'corge', 'grault', 'garply', 'waldo', 'fred']}
df = pd.DataFrame(data)
# 取前十行并转换为列表
first_ten_rows = df.head(10).values.tolist()
# 打印列表
print(first_ten_rows)
```
运行上述代码后,得到的输出结果为:
```
[[1, 'foo'], [2, 'bar'], [3, 'baz'], [4, 'qux'], [5, 'quux'], [6, 'corge'], [7, 'grault'], [8, 'garply'], [9, 'waldo'], [10, 'fred']]
```
在这个例子中,我们使用 `head(10)` 方法获取 DataFrame 的前十行数据,然后使用 `values.tolist()` 方法将其转换为一个列表。
注意,如果 DataFrame 中包含多个列,每一行的值将作为一个子列表的元素。
相关问题
import math import numpy as np import pandas as pd import matplotlib.pyplot as plt def get_list_x(a, b, h): list_x = [] # [0,n+1) nn = math.ceil((b - a )/ h) for i in range(nn): list_x.append(a + i * h) #list.append(a) 在列表后添加 a list_x.append(b) return list_x # 求K值。 fx为方程表达式 def get_k(x, y): k = x+1-y return k # 主循环进行计算 list_fx 为 存储fx值的list def runge_kutta(h): k1 = get_k(x, y) k2 = get_k(x + h/2, y + h/2 * k1) k3 = get_k(x + h/2, y + h/2 * k2) k4 = get_k(x + h, y + h * k3) value_y = y + h * (k1 + 2 * k2 + 2 * k3 + k4) / 6 kk = [k1, k2, k3, k4] return kk, value_y list_x = get_list_x(a=0, b=0.5, h=0.1) list_y = [1] for i in range(len(list_x)): x = list_x[i] y = list_y[i] value_y = runge_kutta(h=0.1) list_y.append(value_y) print(list_y[1])
这段代码有一些问题:
1. 第一行的导入语句应该每个模块名之间用逗号隔开,而不是直接连在一起。
2. 在 `get_list_x` 函数中,如果 `h` 不能整除 `(b-a)`,那么最后一个元素可能会小于或等于 `b`,这样可能导致后续计算出错。应该将 `nn` 的计算方式修改为向上取整。
3. 在 `runge_kutta` 函数中,没有将 `value_y` 返回,而是返回了一个包含 `k1` 到 `k4` 的列表和 `value_y`。应该将这个函数的返回值修改为 `value_y`。
4. 在 `runge_kutta` 函数中,`get_k` 函数的参数 `x` 和 `y` 没有传入,需要在调用 `runge_kutta` 函数时将它们传入。
5. 在 `list_y` 列表的初始化中,应该将第一个元素初始化为 `1.0`,而不是 `1`。
下面是修改后的代码:
```python
import math
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
def get_list_x(a, b, h):
list_x = []
nn = math.ceil((b - a) / h)
for i in range(nn):
list_x.append(a + i * h)
if list_x[-1] < b:
list_x.append(b)
return list_x
def get_k(x, y):
k = x + 1 - y
return k
def runge_kutta(x, y, h):
k1 = get_k(x, y)
k2 = get_k(x + h/2, y + h/2 * k1)
k3 = get_k(x + h/2, y + h/2 * k2)
k4 = get_k(x + h, y + h * k3)
value_y = y + h * (k1 + 2 * k2 + 2 * k3 + k4) / 6
return value_y
list_x = get_list_x(a=0, b=0.5, h=0.1)
list_y = [1.0]
for i in range(len(list_x) - 1):
x = list_x[i]
y = list_y[-1]
value_y = runge_kutta(x, y, h=0.1)
list_y.append(value_y)
print(list_y[-1])
```
注意,这个代码中只是修复了一些语法和逻辑上的错误,但并没有检查数学上是否正确。因此,如果你在运行代码时发现结果不正确,可能需要重新检查算法的正确性。
pandas_highcharts
引用提到了一个基于Python的第三方库python-highcharts,该库可以帮助不擅长前端语言的人使用Highcharts来创建图表。你可以在GitHub上找到该库的详细说明和使用方法。引用给出了一个使用python-highcharts库创建区间柱状图的示例代码,其中使用了最小值和最大值来表示区间范围,并设置了图表的各种属性和样式。引用则提供了一个使用pandas和pandas_highcharts库创建折线图的示例代码,其中使用了DataFrame的数据来添加折线图的数值。
所以,pandas_highcharts是一个用于在Python中创建图表的库,它可以与pandas库一起使用,方便地将数据可视化为各种类型的图表,包括区间柱状图和折线图。这个库的使用方法可以参考以上提到的示例代码和GitHub上的文档。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [又一个可视化神器Highcharts,Python版也有哦!](https://blog.csdn.net/lemonbit/article/details/114324625)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
- *3* [在使用Python Pyecharts可视化库显示Pandas数据图时无法正常显示的错误及解决方法](https://blog.csdn.net/weixin_55674264/article/details/116431034)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐
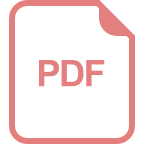
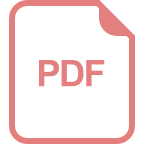
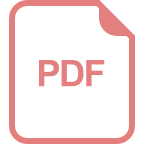
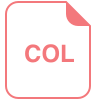
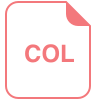











