注释一下这段代码public static class SalesReducer extends Reducer<Text, Text, Text, Text> { private Text reduceOutputKey = new Text(); private Text reduceOutputValue = new Text(); public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException { String[] tokens = key.toString().split(" "); String month = tokens[0]; String sales = tokens[1]; List<String> productList = new ArrayList<>(); for (Text value : values) { productList.add(value.toString()); } Collections.sort(productList); productList.add(values.toString()); Collections.reverse(productList); for (String product : productList) { reduceOutputKey.set(month + " " + product); reduceOutputValue.set(sales); context.write(reduceOutputKey, reduceOutputValue); } } }
时间: 2024-02-14 09:16:33 浏览: 22
这是一段 Java 代码,以下是对其的注释:
```
public static class SalesReducer extends Reducer<Text, Text, Text, Text> {
// 定义 reduce 阶段的输出键和值
private Text reduceOutputKey = new Text();
private Text reduceOutputValue = new Text();
// reduce 函数,对每个 key 所对应的 values 进行处理
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
// 对 key 进行分割,获取 month 和 sales
String[] tokens = key.toString().split(" ");
String month = tokens[0];
String sales = tokens[1];
// 定义用于存放产品名的列表
List<String> productList = new ArrayList<>();
// 将 values 中的产品名加入 productList
for (Text value : values) {
productList.add(value.toString());
}
// 对 productList 进行排序和反转
Collections.sort(productList);
Collections.reverse(productList);
// 对 productList 中的每个产品,设置 reduceOutputKey 和 reduceOutputValue,并将其写入 context
for (String product : productList) {
reduceOutputKey.set(month + " " + product);
reduceOutputValue.set(sales);
context.write(reduceOutputKey, reduceOutputValue);
}
}
}
```
注释解释:
- `public static class SalesReducer extends Reducer<Text, Text, Text, Text>` 定义了一个 SalesReducer 类,该类继承了 Reducer 类,并指定了输入键和值的类型为 Text,输出键和值的类型也为 Text。
- `private Text reduceOutputKey = new Text();` 和 `private Text reduceOutputValue = new Text();` 定义了 reduce 阶段的输出键和值。
- `public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException` 是 reduce 函数,对每个 key 所对应的 values 进行处理。
- `String[] tokens = key.toString().split(" ");` 对 key 进行分割,获取 month 和 sales。
- `List<String> productList = new ArrayList<>();` 定义用于存放产品名的列表。
- `for (Text value : values) { productList.add(value.toString()); }` 将 values 中的产品名加入 productList。
- `Collections.sort(productList);` 对 productList 进行排序。
- `Collections.reverse(productList);` 对 productList 进行反转。
- `reduceOutputKey.set(month + " " + product);` 和 `reduceOutputValue.set(sales);` 分别设置 reduce 阶段的输出键和值。
- `context.write(reduceOutputKey, reduceOutputValue);` 将 reduce 阶段的输出键和值写入 context。
相关推荐
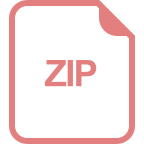
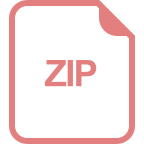
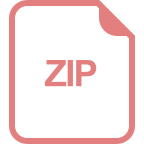










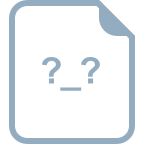
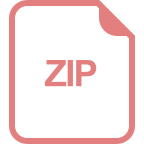
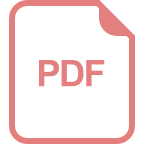
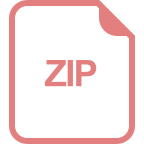
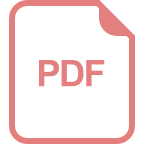