当QGraphicsItem超出QGraphicsScene边界,不做扩展边界处理
时间: 2024-01-24 11:15:31 浏览: 63
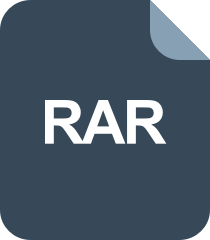
QT QGraphicsScene

当QGraphicsItem超出QGraphicsScene边界时,不做扩展边界处理的方法如下:
1. 可以在QGraphicsItem的moveEvent中,手动判断是否超出边界,如果超出边界则不移动或者移动到边界位置。
2. 也可以通过设置QGraphicsScene的边界来限制QGraphicsItem的移动范围,不允许超出边界。
具体实现可以参考以下代码:
```python
class MyItem(QGraphicsItem):
def __init__(self):
super().__init__()
def moveEvent(self, event):
# 获取当前场景范围
scene_rect = self.scene().sceneRect()
# 获取当前item的范围
item_rect = self.boundingRect()
# 计算item的新位置
new_pos = event.pos()
if new_pos.x() + item_rect.width() > scene_rect.right():
new_pos.setX(scene_rect.right() - item_rect.width())
if new_pos.x() < scene_rect.left():
new_pos.setX(scene_rect.left())
if new_pos.y() + item_rect.height() > scene_rect.bottom():
new_pos.setY(scene_rect.bottom() - item_rect.height())
if new_pos.y() < scene_rect.top():
new_pos.setY(scene_rect.top())
# 移动item
self.setPos(new_pos)
```
或者:
```python
class MyScene(QGraphicsScene):
def __init__(self):
super().__init__()
# 设置场景范围
self.setSceneRect(0, 0, 800, 600)
def mouseMoveEvent(self, event):
# 获取当前item的范围
item_rect = self.selectedItems()[0].boundingRect()
# 获取当前鼠标位置
mouse_pos = event.scenePos()
# 判断是否超出边界
if mouse_pos.x() + item_rect.width() > self.sceneRect().right():
mouse_pos.setX(self.sceneRect().right() - item_rect.width())
if mouse_pos.x() < self.sceneRect().left():
mouse_pos.setX(self.sceneRect().left())
if mouse_pos.y() + item_rect.height() > self.sceneRect().bottom():
mouse_pos.setY(self.sceneRect().bottom() - item_rect.height())
if mouse_pos.y() < self.sceneRect().top():
mouse_pos.setY(self.sceneRect().top())
# 移动item
self.selectedItems()[0].setPos(mouse_pos)
```
阅读全文
相关推荐
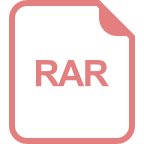
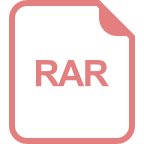


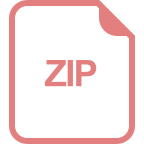
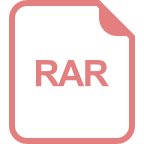

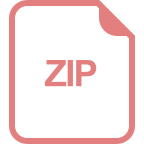
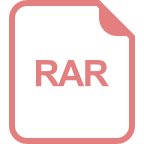
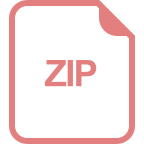
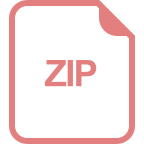






