写一个基于stm32f103c8t6的驱动步进电机的代码
时间: 2023-08-28 07:07:48 浏览: 118
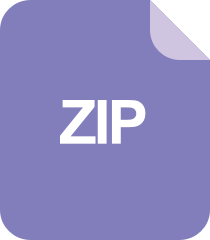
STM32控制步进电机28BYJ-48.zip

以下是一个简单的基于STM32F103C8T6的驱动步进电机的代码,使用的是HAL库:
```c
#include "stm32f1xx_hal.h"
#define STEPPER_MOTOR_STEPS_PER_REV 200 // 步进电机每转的步数
#define STEPPER_MOTOR_MOTOR_STEPS (STEPPER_MOTOR_STEPS_PER_REV * 4) // 步进电机总步数(4相驱动)
// 步进电机引脚定义
#define STEPPER_MOTOR_PIN_1 GPIO_PIN_0
#define STEPPER_MOTOR_PIN_2 GPIO_PIN_1
#define STEPPER_MOTOR_PIN_3 GPIO_PIN_2
#define STEPPER_MOTOR_PIN_4 GPIO_PIN_3
#define STEPPER_MOTOR_PORT GPIOA
// 步进电机运动方向定义
#define STEPPER_MOTOR_DIR_FORWARD 0 // 正向
#define STEPPER_MOTOR_DIR_BACKWARD 1 // 反向
// 步进电机速度定义
#define STEPPER_MOTOR_SPEED_1 100 // 速度1
#define STEPPER_MOTOR_SPEED_2 50 // 速度2
// 步进电机控制状态定义
typedef enum {
STEPPER_MOTOR_STOPPED = 0, // 停止状态
STEPPER_MOTOR_MOVING_FORWARD, // 正向运动中
STEPPER_MOTOR_MOVING_BACKWARD // 反向运动中
} stepper_motor_state_t;
// 步进电机控制结构体
typedef struct {
uint16_t steps_per_rev; // 每转的步数
uint16_t motor_steps; // 总步数
GPIO_TypeDef* port; // 引脚所在端口
uint16_t pin_1; // 引脚1
uint16_t pin_2; // 引脚2
uint16_t pin_3; // 引脚3
uint16_t pin_4; // 引脚4
stepper_motor_state_t state; // 控制状态
uint16_t speed; // 速度
uint16_t current_step; // 当前步数
} stepper_motor_t;
// 步进电机初始化
void stepper_motor_init(stepper_motor_t* motor) {
// 初始化引脚
GPIO_InitTypeDef GPIO_InitStruct = {0};
GPIO_InitStruct.Pin = motor->pin_1 | motor->pin_2 | motor->pin_3 | motor->pin_4;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(motor->port, &GPIO_InitStruct);
// 初始化控制状态
motor->state = STEPPER_MOTOR_STOPPED;
motor->speed = STEPPER_MOTOR_SPEED_1;
motor->current_step = 0;
}
// 步进电机启动
void stepper_motor_start(stepper_motor_t* motor, uint8_t direction) {
// 设置控制状态
motor->state = direction ? STEPPER_MOTOR_MOVING_BACKWARD : STEPPER_MOTOR_MOVING_FORWARD;
// 开始控制电机
while (motor->state != STEPPER_MOTOR_STOPPED) {
// 计算下一步的位置
int8_t step_number = motor->current_step % 4;
// 控制引脚输出
switch (step_number) {
case 0:
HAL_GPIO_WritePin(motor->port, motor->pin_1, GPIO_PIN_SET);
HAL_GPIO_WritePin(motor->port, motor->pin_2, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_3, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_4, GPIO_PIN_RESET);
break;
case 1:
HAL_GPIO_WritePin(motor->port, motor->pin_1, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_2, GPIO_PIN_SET);
HAL_GPIO_WritePin(motor->port, motor->pin_3, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_4, GPIO_PIN_RESET);
break;
case 2:
HAL_GPIO_WritePin(motor->port, motor->pin_1, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_2, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_3, GPIO_PIN_SET);
HAL_GPIO_WritePin(motor->port, motor->pin_4, GPIO_PIN_RESET);
break;
case 3:
HAL_GPIO_WritePin(motor->port, motor->pin_1, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_2, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_3, GPIO_PIN_RESET);
HAL_GPIO_WritePin(motor->port, motor->pin_4, GPIO_PIN_SET);
break;
}
// 延时,控制电机速度
HAL_Delay(motor->speed);
// 更新当前步数
motor->current_step += direction ? -1 : 1;
// 判断是否到达目标位置
if (motor->current_step >= motor->motor_steps || motor->current_step < 0) {
motor->state = STEPPER_MOTOR_STOPPED;
}
}
}
// 步进电机停止
void stepper_motor_stop(stepper_motor_t* motor) {
motor->state = STEPPER_MOTOR_STOPPED;
}
// 示例代码
int main(void) {
// 初始化步进电机控制结构体
stepper_motor_t motor = {
.steps_per_rev = STEPPER_MOTOR_STEPS_PER_REV,
.motor_steps = STEPPER_MOTOR_MOTOR_STEPS,
.port = STEPPER_MOTOR_PORT,
.pin_1 = STEPPER_MOTOR_PIN_1,
.pin_2 = STEPPER_MOTOR_PIN_2,
.pin_3 = STEPPER_MOTOR_PIN_3,
.pin_4 = STEPPER_MOTOR_PIN_4,
.state = STEPPER_MOTOR_STOPPED,
.speed = STEPPER_MOTOR_SPEED_1,
.current_step = 0
};
stepper_motor_init(&motor);
// 启动步进电机,正向运动
stepper_motor_start(&motor, STEPPER_MOTOR_DIR_FORWARD);
// 停止步进电机
stepper_motor_stop(&motor);
// 启动步进电机,反向运动
stepper_motor_start(&motor, STEPPER_MOTOR_DIR_BACKWARD);
// 停止步进电机
stepper_motor_stop(&motor);
while (1) {}
}
```
注意:这只是一个简单的示例代码,实际使用时需要根据具体的步进电机型号和驱动器进行调整。同时,步进电机的控制需要较高的精度和稳定性,建议使用专门的步进电机驱动器。
阅读全文
相关推荐
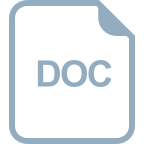
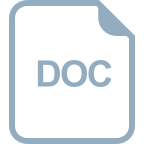

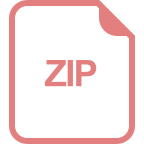
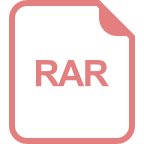
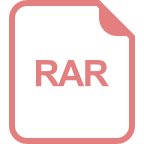











