用java代码编写调用flink restful接口提交批处理作业
时间: 2024-01-26 07:14:26 浏览: 30
好的,以下是一个简单的Java代码示例,用于调用Flink RESTful接口提交批处理作业:
```java
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.ContentType;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClientBuilder;
import java.io.IOException;
public class FlinkRestClient {
public static void main(String[] args) throws IOException {
String flinkRestUrl = "http://localhost:8081/jars/upload"; // Flink RESTful接口URL
String jarPath = "/path/to/your/jar/file"; // 批处理作业jar包路径
// 构造请求体
String requestBody = "{\"entryClass\":\"com.example.batch.BatchJob\","
+ "\"programArgs\":[\"--input\",\"/path/to/input\",\"--output\",\"/path/to/output\"]}";
// 构造POST请求
HttpPost postRequest = new HttpPost(flinkRestUrl);
postRequest.setHeader("Accept", "application/json");
postRequest.setHeader("Content-type", "application/json");
postRequest.setHeader("User-Agent", "FlinkRestClient/1.0");
// 设置jar文件实体
StringEntity fileEntity = new StringEntity(jarPath, ContentType.APPLICATION_OCTET_STREAM);
postRequest.setEntity(fileEntity);
// 设置请求体实体
StringEntity bodyEntity = new StringEntity(requestBody, ContentType.APPLICATION_JSON);
postRequest.setEntity(bodyEntity);
// 发送POST请求
HttpResponse response = HttpClientBuilder.create().build().execute(postRequest);
// 解析响应
if (response.getStatusLine().getStatusCode() == 200) {
System.out.println("Job submitted successfully!");
} else {
System.out.println("Failed to submit job.");
}
}
}
```
以上代码仅供参考,实际情况中可能需要根据不同的Flink版本和RESTful接口进行修改。
相关推荐
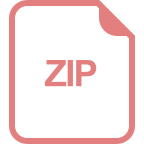
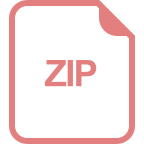
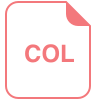
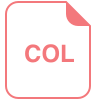
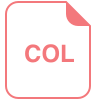
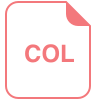
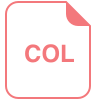









