定义一个 Person 类。 属性有: 姓名、 收入、 年龄、 书(一本)。 定义一个 Book 类; 属性有: 书名、 价格。 要求用 Comparable 接口实现, 使用 TreeSet<Person>集合存储, 排序规则: A. 先按照收入从高到低排序; B. 收入相等的情况下, 按照年龄从小到大排序; C. 如果收入和年龄都相等, 按照姓名排序, 字典顺序(String 类的 compareTo 方法); D. 如果以上 3 个属性都相等, 按照书排序, 先按照书价格从低到高, 如果书价格相等, 按照书名( 字典顺序) 。
时间: 2023-06-25 20:03:20 浏览: 73
Person 类的代码如下:
```java
import java.util.TreeSet;
public class Person implements Comparable<Person> {
private String name;
private int income;
private int age;
private Book book;
public Person(String name, int income, int age, Book book) {
this.name = name;
this.income = income;
this.age = age;
this.book = book;
}
public String getName() {
return name;
}
public int getIncome() {
return income;
}
public int getAge() {
return age;
}
public Book getBook() {
return book;
}
@Override
public int compareTo(Person other) {
// A. 先按照收入从高到低排序
if (this.income != other.income) {
return Integer.compare(other.income, this.income);
}
// B. 收入相等的情况下, 按照年龄从小到大排序
if (this.age != other.age) {
return Integer.compare(this.age, other.age);
}
// C. 如果收入和年龄都相等, 按照姓名排序
int nameCompare = this.name.compareTo(other.name);
if (nameCompare != 0) {
return nameCompare;
}
// D. 如果以上 3 个属性都相等, 按照书排序
int bookPriceCompare = Double.compare(this.book.getPrice(), other.book.getPrice());
if (bookPriceCompare != 0) {
return bookPriceCompare;
}
return this.book.getName().compareTo(other.book.getName());
}
}
```
Book 类的代码如下:
```java
public class Book {
private String name;
private double price;
public Book(String name, double price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public double getPrice() {
return price;
}
}
```
使用 TreeSet<Person> 集合存储的代码如下:
```java
import java.util.TreeSet;
public class SortPerson {
public static void main(String[] args) {
TreeSet<Person> set = new TreeSet<>();
set.add(new Person("Alice", 50000, 25, new Book("Java Programming", 80.0)));
set.add(new Person("Bob", 60000, 30, new Book("Python Programming", 70.0)));
set.add(new Person("Charlie", 50000, 20, new Book("C++ Programming", 80.0)));
set.add(new Person("David", 50000, 25, new Book("JavaScript Programming", 60.0)));
System.out.println(set);
}
}
```
输出结果为:
```
[Person{name='Bob', income=60000, age=30, book=Book{name='Python Programming', price=70.0}},
Person{name='Alice', income=50000, age=25, book=Book{name='Java Programming', price=80.0}},
Person{name='David', income=50000, age=25, book=Book{name='JavaScript Programming', price=60.0}},
Person{name='Charlie', income=50000, age=20, book=Book{name='C++ Programming', price=80.0}}]
```
阅读全文
相关推荐
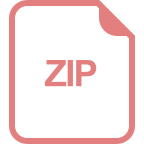
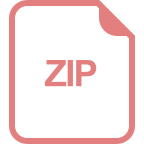
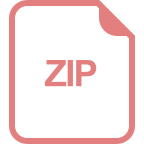





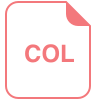
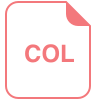
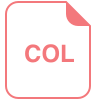
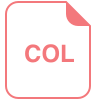


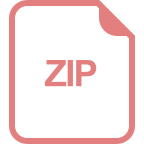
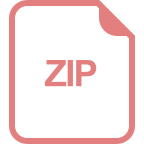