resource = requests.get(url=url,params=None,headers=headers)
时间: 2023-10-24 09:05:15 浏览: 90
这是一个使用Python的requests库发送HTTP GET请求的基本语法。其中:
- url是要请求的URL地址。
- params是一个字典类型的参数,可以包含在URL中的查询字符串参数。
- headers是一个字典类型的参数,包含HTTP请求头部信息。
例如,以下代码将向https://www.example.com发送一个不包含查询参数的GET请求,并设置了一个自定义的HTTP头部:
```python
import requests
url = 'https://www.example.com'
headers = {'User-Agent': 'Mozilla/5.0'}
response = requests.get(url=url, headers=headers)
print(response.status_code)
print(response.text)
```
这将输出服务器响应的状态码和内容。
相关问题
resource = requests.get(url=url,params=None,headers=headers)报错
这个报错信息比较简略,可能是由于多种原因引起的。一般来说,可以从以下几个方面排查问题:
1. 检查 `url` 变量是否正确,并且确保能够访问该 URL。
2. 检查 `headers` 变量是否正确,如果需要添加特定的请求头,可以查看 API 文档中的要求并添加对应的请求头。
3. 如果需要传递参数,检查 `params` 变量是否正确,并且确保参数格式正确。
4. 如果以上步骤都没有问题,可能是由于网络原因导致的,可以尝试重试请求或者检查网络连接是否正常。
如果以上方法仍然无法解决问题,建议提供更详细的报错信息或者上下文,以便更好地定位问题。
session.get
Session.get() is a method used to send an HTTP GET request to a specified URL and return the response. It is a part of the Requests library in Python and is used to retrieve information from a server.
Syntax:
response = session.get(url, params=None, **kwargs)
Here,
- url: A string representing the URL of the resource to be retrieved.
- params: (optional) A dictionary, list of tuples, or bytes to be sent in the query string of the URL.
- **kwargs: (optional) Any other parameters that can be passed to the requests library, such as headers or timeout.
The session object in Requests allows you to persist certain parameters across requests. This can be useful when working with APIs that require authentication or when you need to send multiple requests to the same server.
Example:
import requests
# create a session object
session = requests.Session()
# set headers for all requests
headers = {'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.36'}
session.headers.update(headers)
# send a GET request to a URL
response = session.get('https://www.example.com')
# print the response content
print(response.content)
阅读全文
相关推荐
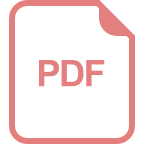
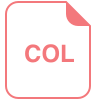
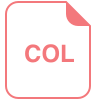
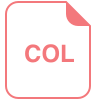
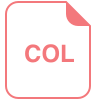
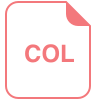
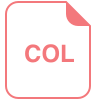
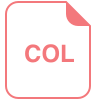
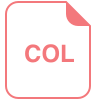
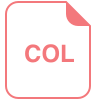
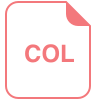
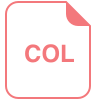
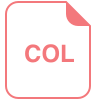
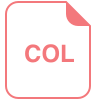
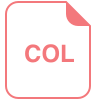