如何用rospy实现ros小海龟在(5.54,5.54),(5.54,8.54),(6.54,7.54),(5.54,6.54),(5.54,5.54)这几个坐标点中循环移动,要求是小海龟先转弯后直行,且能实现闭环控制
时间: 2024-02-02 08:03:26 浏览: 22
首先,需要安装Turtlebot3 package,通过以下命令安装:
```
sudo apt-get install ros-<ros_version>-turtlebot3
```
接着,可以创建一个ROS package(假设名称为`turtlebot3_nav`),并在`src`文件夹下创建一个`nav.py`文件,用于控制小海龟移动。
```python
#!/usr/bin/env python
import rospy
from geometry_msgs.msg import Twist
from turtlesim.msg import Pose
from math import pow, atan2, sqrt
class TurtleBot:
def __init__(self):
rospy.init_node('turtlebot_controller', anonymous=True)
self.velocity_publisher = rospy.Publisher('/cmd_vel', Twist, queue_size=10)
self.pose_subscriber = rospy.Subscriber('/pose', Pose, self.update_pose)
self.pose = Pose()
self.rate = rospy.Rate(10)
def update_pose(self, data):
self.pose = data
self.pose.x = round(self.pose.x, 4)
self.pose.y = round(self.pose.y, 4)
def move(self, goal_pose):
distance_tolerance = 0.1
vel_msg = Twist()
while self.get_distance(self.pose, goal_pose) > distance_tolerance:
# Proportional controller
# Linear velocity in the x-axis
vel_msg.linear.x = 1.5 * sqrt(pow((goal_pose.x - self.pose.x), 2) + pow((goal_pose.y - self.pose.y), 2))
vel_msg.linear.y = 0
vel_msg.linear.z = 0
# Angular velocity in the z-axis
vel_msg.angular.x = 0
vel_msg.angular.y = 0
vel_msg.angular.z = 4 * (atan2(goal_pose.y - self.pose.y, goal_pose.x - self.pose.x) - self.pose.theta)
# Publishing our vel_msg
self.velocity_publisher.publish(vel_msg)
# Publish at the desired rate.
self.rate.sleep()
# Stopping our robot after the movement is over
vel_msg.linear.x = 0
vel_msg.angular.z = 0
self.velocity_publisher.publish(vel_msg)
def get_distance(self, goal_pose_a, goal_pose_b):
distance = sqrt(pow((goal_pose_a.x - goal_pose_b.x), 2) + pow((goal_pose_a.y - goal_pose_b.y), 2))
return distance
if __name__ == '__main__':
try:
x = [5.54, 5.54, 6.54, 5.54, 5.54]
y = [5.54, 8.54, 7.54, 6.54, 5.54]
turtle = TurtleBot()
while not rospy.is_shutdown():
for i in range(len(x)):
goal_pose = Pose()
goal_pose.x = x[i]
goal_pose.y = y[i]
turtle.move(goal_pose)
except rospy.ROSInterruptException:
pass
```
在主函数中,`x`和`y`分别存储了5个坐标点。在`while`循环中,将每个坐标点作为目标位置传递给`move`函数,小海龟将依次移动到这些位置。
在`move`函数中,通过计算小海龟当前位置与目标位置之间的距离,使用比例控制器计算线速度和角速度,控制小海龟移动。当小海龟到达目标位置时,将停止移动。
最后,需要在终端中运行以下命令以启动ROS节点:
```
roscore
```
在另一个终端中,使用以下命令启动Gazebo仿真环境:
```
roslaunch turtlebot3_gazebo turtlebot3_world.launch
```
然后,运行`nav.py`文件:
```
rosrun turtlebot3_nav nav.py
```
小海龟将在给定的5个坐标点之间循环移动。
相关推荐
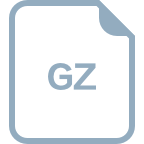
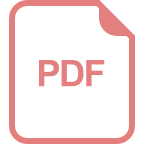














