将金字塔模块插入到一个五层unet网络中,给出python代码
时间: 2024-02-17 17:01:15 浏览: 71
好的,以下是一个示例的五层UNet网络中插入金字塔模块的Python代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
# 定义金字塔模块
class PyramidModule(nn.Module):
def __init__(self, in_channels):
super(PyramidModule, self).__init__()
self.conv1 = nn.Conv2d(in_channels, in_channels // 2, kernel_size=1)
self.conv2 = nn.Conv2d(in_channels // 2, in_channels // 2, kernel_size=3, padding=1)
self.conv3 = nn.Conv2d(in_channels // 2, in_channels // 2, kernel_size=3, padding=1)
self.conv4 = nn.Conv2d(in_channels // 2, in_channels, kernel_size=1)
def forward(self, x):
x1 = self.conv1(x)
x2 = self.conv2(x1)
x3 = self.conv3(x2)
x4 = self.conv4(x3)
out = torch.cat([x1, x2, x3, x4], dim=1)
return out
# 定义五层UNet网络
class UNet(nn.Module):
def __init__(self, in_channels, out_channels):
super(UNet, self).__init__()
self.conv1 = nn.Conv2d(in_channels, 64, kernel_size=3, padding=1)
self.conv2 = nn.Conv2d(64, 128, kernel_size=3, padding=1)
self.conv3 = nn.Conv2d(128, 256, kernel_size=3, padding=1)
self.conv4 = nn.Conv2d(256, 512, kernel_size=3, padding=1)
self.conv5 = nn.Conv2d(512, 1024, kernel_size=3, padding=1)
self.pool = nn.MaxPool2d(kernel_size=2, stride=2)
self.upsample = nn.Upsample(scale_factor=2, mode='bilinear', align_corners=True)
self.pyramid = PyramidModule(1024)
self.upconv4 = nn.ConvTranspose2d(1024, 512, kernel_size=2, stride=2)
self.upconv3 = nn.ConvTranspose2d(512, 256, kernel_size=2, stride=2)
self.upconv2 = nn.ConvTranspose2d(256, 128, kernel_size=2, stride=2)
self.upconv1 = nn.ConvTranspose2d(128, 64, kernel_size=2, stride=2)
self.outconv = nn.Conv2d(64, out_channels, kernel_size=1)
def forward(self, x):
x1 = F.relu(self.conv1(x))
x2 = F.relu(self.conv2(self.pool(x1)))
x3 = F.relu(self.conv3(self.pool(x2)))
x4 = F.relu(self.conv4(self.pool(x3)))
x5 = F.relu(self.conv5(self.pool(x4)))
x5 = self.pyramid(x5) # 插入金字塔模块
x4 = F.relu(self.upconv4(x5) + x4)
x3 = F.relu(self.upconv3(x4) + x3)
x2 = F.relu(self.upconv2(x3) + x2)
x1 = F.relu(self.upconv1(x2) + x1)
out = self.outconv(x1)
return out
```
这里我们定义了一个金字塔模块(PyramidModule),并将其插入到了一个五层UNet网络(UNet)中。具体来说,我们在原本的UNet网络的最后一层卷积层后面插入了金字塔模块。在前向传播过程中,我们首先对输入图像进行五次卷积操作,然后再通过金字塔模块进行特征融合。最后,我们通过四次反卷积操作将图像大小还原,并输出最终的预测结果。
阅读全文
相关推荐
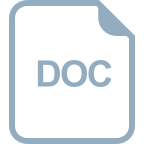
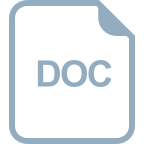
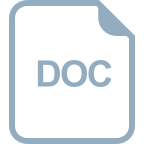













