C++解题:链接:https://www.luogu.com.cn/problem/SP1730
时间: 2023-10-07 13:13:12 浏览: 99
题目描述
给出 $n$ 个数,其中存在一些数是可以被表示成 $a^k$ 的形式的,求这些数中最大的那个。
输入格式
第一行一个正整数 $n$,表示数据组数。
每组数据第一行是一个正整数 $m$,表示该组数据共有 $m$ 个数。
接下来一行 $m$ 个整数,表示这 $m$ 个数。
输出格式
每组数据输出一行,表示这组数据中最大的那个可以表示成 $a^k$ 的数,若不存在,则输出 $-1$。
数据范围
$1\le n\le 10^3,1\le m\le 10^4,1\le a\le 10^5,2\le k\le 30$
输入样例1
2
3
27 2 3
4
1 1 1 1
输出样例1
27
-1
输入样例2
1
5
1 2 3 4 5
输出样例2
4
C++代码
相关问题
https://www.luogu.com.cn/problem/P1335C++代码
以下是一份参考代码,可以用于计算 $f(n)$ 的值:
```c++
#include <iostream>
using namespace std;
int main() {
int n;
cin >> n;
int f1 = 1, f2 = 2, f3;
if (n == 1) {
cout << f1 << endl;
} else if (n == 2) {
cout << f2 << endl;
} else {
for (int i = 3; i <= n; i++) {
f3 = 2 * f2 - f1 + 2;
f1 = f2;
f2 = f3;
}
cout << f3 << endl;
}
return 0;
}
```
代码中使用了三个变量 $f1,f2,f3$ 分别表示 $f(n-2),f(n-1),f(n)$ 的值,通过递推计算得出 $f(n)$ 的值。
需要注意的是,当 $n=1$ 或 $n=2$ 时,需要特判处理。
帮我用C++写一道题,这道题的链接是这样的:https://www.luogu.com.cn/problem/P1598
好的,这是一道比较简单的题目,以下是C++代码实现:
```c++
#include<iostream>
#include<vector>
using namespace std;
int main()
{
vector<int> a(26, 0); // 存储每个字母的数量
string s;
for(int i = 0; i < 4; i++)
{
getline(cin, s); // 读入每一行
for(int j = 0; j < s.size(); j++)
{
if(s[j] >= 'A' && s[j] <= 'Z') // 判断是否为大写字母
{
a[s[j] - 'A']++; // 计数加1
}
}
}
int maxn = 0;
for(int i = 0; i < 26; i++)
{
maxn = max(maxn, a[i]); // 找到出现次数最多的字母数量
}
for(int i = maxn; i > 0; i--)
{
for(int j = 0; j < 26; j++)
{
if(a[j] >= i)
{
cout << "* ";
}
else
{
cout << " ";
}
}
cout << endl;
}
for(int i = 0; i < 26; i++)
{
cout << (char)('A' + i) << " "; // 输出字母
}
return 0;
}
```
希望能对你有所帮助!
阅读全文
相关推荐
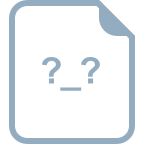













