用C语言写一个迪杰斯特拉的算法
时间: 2023-08-31 12:34:06 浏览: 88
### 回答1:
好的,迪杰斯特拉算法是一种用于解决最短路径问题的算法。下面是一个使用C语言实现迪杰斯特拉算法的例子:
```
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
#define MIN(X,Y) ((X) < (Y) ? (X) : (Y))
#define MAX_VERTICES 100
#define INFINITE INT_MAX
#define UNDEFINED -1
int n, m;
int u, v, w;
int distance[MAX_VERTICES];
int previous[MAX_VERTICES];
int visited[MAX_VERTICES];
int adjacency_matrix[MAX_VERTICES][MAX_VERTICES];
int main(int argc, char *argv[]) {
int i, j;
int source;
int destination;
// Initialize the distances and the previous vertex for each vertex
for (i = 0; i < MAX_VERTICES; i++) {
distance[i] = INFINITE;
previous[i] = UNDEFINED;
}
// Read in the number of vertices and edges
scanf("%d%d", &n, &m);
// Read in the adjacency matrix
for (i = 0; i < n; i++) {
for (j = 0; j < n; j++) {
scanf("%d", &adjacency_matrix[i][j]);
}
}
// Read in the source and destination vertices
scanf("%d%d", &source, &destination);
// Set the distance to the source vertex to 0
distance[source] = 0;
// Dijkstra's algorithm
while (1) {
// Find the vertex with the minimum distance
int min_distance = INFINITE;
int min_distance_vertex = UNDEFINED;
for (i = 0; i < n; i++) {
if (!visited[i] && distance[i] < min_distance) {
min_distance = distance[i];
min_distance_vertex = i;
}
}
// Break if all vertices have been visited
if (min_distance_vertex == UNDEFINED) {
break;
}
// Mark the vertex as visited
visited[min_distance_vertex] = 1;
// Update the distances of the neighboring vertices
for (i = 0; i < n; i++) {
if (adjacency_matrix[min_distance_vertex][i] != INFINITE) {
if (distance[i] > distance[min_distance_vertex] + adjacency_matrix[min_distance_vertex][i]) {
distance[i] = distance[min_distance_vertex] + adjacency_matrix[min
### 回答2:
迪杰斯特拉(Dijkstra)算法是一种用于解决单源最短路径问题的算法。以下是用C语言实现迪杰斯特拉算法的伪代码:
1. 首先,创建一个用于储存图的邻接矩阵的二维数组graph,将所有的边的权重初始化为无穷大,将顶点的距离初始化为无穷大,创建一个记录顶点是否被访问的数组visited。
2. 选择一个起始顶点start,将其距离设为0,并将visited[start]标记为真。
3. 对于所有与start顶点有直接边连接的顶点v,更新其距离为graph[start][v],将距离储存在一个一维数组dist中。
4. 重复以下过程,直到所有的顶点都被访问:
a. 从dist数组中选择一个最小距离的顶点u,将visited[u]标记为真。
b. 遍历所有未被访问的顶点v,如果dist[u] + graph[u][v] < dist[v],则更新dist[v]的值。
5. 最终,dist数组中保存了起始顶点start到其他顶点的最短路径长度。
以下是C语言实现迪杰斯特拉算法的示例代码:
```c
#include <stdio.h>
#define INFINITY 9999
#define MAX_NODES 100
void dijkstra(int graph[MAX_NODES][MAX_NODES], int start, int dist[MAX_NODES], int visited[MAX_NODES], int num_nodes) {
int i, j, min_distance, next_node;
// 初始化距离和访问状态
for (i = 0; i < num_nodes; i++) {
dist[i] = INFINITY;
visited[i] = 0;
}
dist[start] = 0;
for (i = 0; i < num_nodes; i++) {
min_distance = INFINITY;
// 选择距离最小的未访问顶点
for (j = 0; j < num_nodes; j++) {
if (!visited[j] && dist[j] < min_distance) {
min_distance = dist[j];
next_node = j;
}
}
visited[next_node] = 1;
// 更新与next_node连接的顶点的距离
for (j = 0; j < num_nodes; j++) {
if (!visited[j] && graph[next_node][j] != 0 && dist[next_node] + graph[next_node][j] < dist[j]) {
dist[j] = dist[next_node] + graph[next_node][j];
}
}
}
}
int main() {
int graph[MAX_NODES][MAX_NODES] = {
{0, 5, 0, 0, 9},
{5, 0, 2, 0, 0},
{0, 2, 0, 7, 0},
{0, 0, 7, 0, 1},
{9, 0, 0, 1, 0}
};
int num_nodes = 5;
int start_node = 0;
int dist[MAX_NODES], visited[MAX_NODES];
dijkstra(graph, start_node, dist, visited, num_nodes);
printf("Shortest distances from node %d:\n", start_node);
for (int i = 0; i < num_nodes; i++) {
printf("Node %d: %d\n", i, dist[i]);
}
return 0;
}
```
这段代码使用邻接矩阵来表示图,其中的0表示两个顶点之间没有边相连。程序输出起始顶点到其他顶点的最短路径长度。
### 回答3:
迪杰斯特拉算法是一种用于寻找带权重图中单源最短路径的算法。以下是用C语言编写的迪杰斯特拉算法的示例:
```c
#include <stdio.h>
#include <limits.h>
#define V 9 // 图中顶点的数量
int minDistance(int dist[], int sptSet[])
{
int min = INT_MAX, min_index;
for (int v = 0; v < V; v++)
{
if (sptSet[v] == 0 && dist[v] <= min)
{
min = dist[v];
min_index = v;
}
}
return min_index;
}
void printSolution(int dist[])
{
printf("顶点 距离\n");
for (int i = 0; i < V; i++)
{
printf("%d %d\n", i, dist[i]);
}
}
void dijkstra(int graph[V][V], int src)
{
int dist[V]; // 存储源点到顶点i的最短距离
int sptSet[V]; // 记录顶点是否在最短路径树中
for (int i = 0; i < V; i++)
{
dist[i] = INT_MAX;
sptSet[i] = 0;
}
dist[src] = 0; // 将源点到自身的距离设为0
for (int count = 0; count < V - 1; count++)
{
int u = minDistance(dist, sptSet);
sptSet[u] = 1;
for (int v = 0; v < V; v++)
{
if (sptSet[v] == 0 && graph[u][v] && dist[u] != INT_MAX && dist[u] + graph[u][v] < dist[v])
{
dist[v] = dist[u] + graph[u][v];
}
}
}
printSolution(dist);
}
int main()
{
int graph[V][V] = {
{0, 4, 0, 0, 0, 0, 0, 8, 0},
{4, 0, 8, 0, 0, 0, 0, 11, 0},
{0, 8, 0, 7, 0, 4, 0, 0, 2},
{0, 0, 7, 0, 9, 14, 0, 0, 0},
{0, 0, 0, 9, 0, 10, 0, 0, 0},
{0, 0, 4, 14, 10, 0, 2, 0, 0},
{0, 0, 0, 0, 0, 2, 0, 1, 6},
{8, 11, 0, 0, 0, 0, 1, 0, 7},
{0, 0, 2, 0, 0, 0, 6, 7, 0}
};
dijkstra(graph, 0);
return 0;
}
```
这段代码实现了迪杰斯特拉算法来找到从源顶点0到其他所有顶点的最短路径。程序中的`graph`数组代表了一个带权重的有向图,可以根据需要进行调整。算法首先创建一个`dist`数组用于存储源点到各顶点的最短距离,然后通过循环遍历顶点并选择最短距离的顶点放入最短路径树中。接下来更新其他顶点的最短距离,最后输出各顶点和它们距离源点的最短距离。
阅读全文
相关推荐
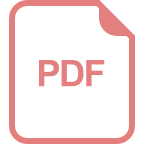
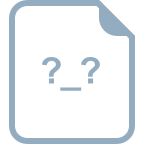















