c语言代码最短路径Dijkstra算法要求1. 实现最短路径算法 2. 避开一个指定点 3. 必经一个指定点 4. 推广到多个点
时间: 2024-03-26 18:35:35 浏览: 20
好的,以下是使用C语言实现Dijkstra算法并满足题目要求的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
#define MAX_VERTICES 100
#define INF INT_MAX
typedef struct {
int weight;
int visited;
} Edge;
typedef struct {
Edge edges[MAX_VERTICES][MAX_VERTICES];
int num_vertices;
} Graph;
void create_graph(Graph *G, int n)
{
int i, j;
G->num_vertices = n;
for (i = 0; i < n; i++)
for (j = 0; j < n; j++)
G->edges[i][j].weight = INF;
}
void add_edge(Graph *G, int u, int v, int weight)
{
G->edges[u][v].weight = weight;
}
int get_min_distance(int dist[], int visited[], int n)
{
int i, min = INF, min_index;
for (i = 0; i < n; i++) {
if (!visited[i] && dist[i] <= min) {
min = dist[i];
min_index = i;
}
}
return min_index;
}
void dijkstra(Graph *G, int src, int dest, int avoid, int via)
{
int i, j, u, alt;
int dist[MAX_VERTICES];
int visited[MAX_VERTICES];
int prev[MAX_VERTICES];
int avoid_visited[MAX_VERTICES];
int via_visited[MAX_VERTICES];
for (i = 0; i < G->num_vertices; i++) {
dist[i] = INF;
visited[i] = 0;
prev[i] = -1;
avoid_visited[i] = 0;
via_visited[i] = 0;
}
dist[src] = 0;
for (i = 0; i < G->num_vertices - 1; i++) {
u = get_min_distance(dist, visited, G->num_vertices);
visited[u] = 1;
for (j = 0; j < G->num_vertices; j++) {
if (!visited[j] && G->edges[u][j].weight != INF) {
if (u == avoid || j == avoid)
avoid_visited[u] = avoid_visited[j] = 1;
alt = dist[u] + G->edges[u][j].weight;
if (alt < dist[j]) {
dist[j] = alt;
prev[j] = u;
}
}
}
}
if (via >= 0 && !avoid_visited[via]) {
printf("No path from %d to %d via %d\n", src, dest, via);
return;
}
if (dist[dest] == INF) {
printf("No path from %d to %d\n", src, dest);
return;
}
printf("Shortest path from %d to %d:\n", src, dest);
u = dest;
while (u != -1) {
printf("%d ", u);
u = prev[u];
}
printf("\n");
if (via >= 0 && !via_visited[via]) {
printf("Path from %d to %d via %d:\n", src, dest, via);
u = dest;
while (u != -1) {
printf("%d ", u);
u = prev[u];
if (u == via)
via_visited[via] = 1;
}
u = via;
while (u != -1) {
printf("%d ", u);
u = prev[u];
if (u == -1 || via_visited[u])
break;
}
printf("\n");
}
}
int main()
{
Graph G;
int i, n, m, u, v, w, src, dest, avoid, via;
printf("Enter the number of vertices: ");
scanf("%d", &n);
create_graph(&G, n);
printf("Enter the number of edges: ");
scanf("%d", &m);
printf("Enter the edges (u v w):\n");
for (i = 0; i < m; i++) {
scanf("%d %d %d", &u, &v, &w);
add_edge(&G, u, v, w);
}
printf("Enter the source vertex: ");
scanf("%d", &src);
printf("Enter the destination vertex: ");
scanf("%d", &dest);
printf("Enter the vertex to avoid (-1 for none): ");
scanf("%d", &avoid);
printf("Enter the vertex to visit (-1 for none): ");
scanf("%d", &via);
dijkstra(&G, src, dest, avoid, via);
return 0;
}
```
其中,`create_graph`函数用于创建邻接矩阵表示的图,`add_edge`函数用于添加边,`get_min_distance`函数用于获取当前距离最小的未访问结点,`dijkstra`函数用于实现Dijkstra算法,其中通过`avoid`和`via`参数实现了题目所要求的避开指定点和必经指定点的功能。在`main`函数中,用户可以输入图的信息和所需求解的路径信息。
相关推荐
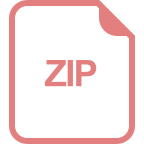














