代码time_start = time.time() results = list() iterations = 2001 lr = 1e-2 model = func_critic_model(input_shape=(None, train_img.shape[1]), act_func='relu') loss_func = tf.keras.losses.MeanSquaredError() alg = "gd" # alg = "gd" for kk in range(iterations): with tf.GradientTape() as tape: predict_label = model(train_img) loss_val = loss_func(predict_label, train_lbl) grads = tape.gradient(loss_val, model.trainable_variables) overall_grad = tf.concat([tf.reshape(grad, -1) for grad in grads], 0) overall_model = tf.concat([tf.reshape(weight, -1) for weight in model.weights], 0) overall_grad = overall_grad + 0.001 * overall_model ## adding a regularization term results.append(loss_val.numpy()) if alg == 'gd': overall_model -= lr * overall_grad ### gradient descent elif alg == 'gdn': ## gradient descent with nestrov's momentum overall_vv_new = overall_model - lr * overall_grad overall_model = (1 + gamma) * oerall_vv_new - gamma * overall_vv overall_vv = overall_new pass model_start = 0 for idx, weight in enumerate(model.weights): model_end = model_start + tf.size(weight) weight.assign(tf.reshape()) for grad, ww in zip(grads, model.weights): ww.assign(ww - lr * grad) if kk % 100 == 0: print(f"Iter: {kk}, loss: {loss_val:.3f}, Duration: {time.time() - time_start:.3f} sec...") input_shape = train_img.shape[1] - 1 model = tf.keras.Sequential([ tf.keras.layers.Input(shape=(input_shape,)), tf.keras.layers.Dense(30, activation="relu"), tf.keras.layers.Dense(20, activation="relu"), tf.keras.layers.Dense(1) ]) n_epochs = 20 batch_size = 100 learning_rate = 0.01 momentum = 0.9 sgd_optimizer = tf.keras.optimizers.SGD(learning_rate=learning_rate, momentum=momentum) model.compile(loss="mean_squared_error", optimizer=sgd_optimizer) history = model.fit(train_img, train_lbl, epochs=n_epochs, batch_size=batch_size, validation_data=(test_img, test_lbl)) nag_optimizer = tf.keras.optimizers.SGD(learning_rate=learning_rate, momentum=momentum, nesterov=True) model.compile(loss="mean_squared_error", optimizer=nag_optimizer) history = model.fit(train_img, train_lbl, epochs=n_epochs, batch_size=batch_size, validation_data=(test_img, test_lbl))运行后报错TypeError: Missing required positional argument,如何改正
时间: 2023-06-26 10:09:26 浏览: 78
这个错误信息并没有指明是哪个函数缺少了必要的位置参数,因此需要仔细检查代码。根据代码的结构,可能是在使用`tf.reshape()`函数时缺少了必要的参数。
具体来说,下面这行代码中出现了这个错误:
```
weight.assign(tf.reshape())
```
`tf.reshape()`函数需要传入两个必要的参数:`tensor`和`shape`。因此,你需要指定这个函数的第一个参数`tensor`,也就是`weight`,以及希望将其reshape成的新形状`shape`。例如,如果你希望将`weight`变成一个形状为`(batch_size, input_shape)`的张量,可以这样写:
```
weight.assign(tf.reshape(weight, (batch_size, input_shape)))
```
请根据你的实际需求修改这行代码,确保`tf.reshape()`函数的两个参数都被正确地指定。
相关问题
如果输入层和隐藏层神经元数均改为5,这段代码怎么改# 第1种取数据方法: X = data_set.iloc[:, 0:20].values.T # 前四列是特征,T表示转置 Y = data_set.iloc[:, 20:].values.T # 后三列是标签 Y = Y.astype('uint8') # 开始训练 start_time = datetime.datetime.now() # 输入20个节点,隐层20个节点,输出1个节点,迭代75000次 parameters = nn_model(X, Y, n_h=20, n_input=20, n_output=1, num_iterations=75000, print_cost=True) end_time = datetime.datetime.now() print("用时:" + str((end_time - start_time).seconds) + 's' + str(round((end_time - start_time).microseconds / 1000)) + 'ms') # 对模型进行测试 # data_test = pd.read_csv('D:\\iris_classification_BPNeuralNetwork-master\\bpnn_V2数据集\\iris_test.csv', header=None) data_test = pd.read_csv('E:\\Program\\nnbc1\\test.csv', header=None) x_test = data_test.iloc[:, 0:20].values.T y_test = data_test.iloc[:, 20:].values.T y_test = y_test.astype('uint8')
如果要将输入层和隐藏层神经元数均改为5,需要将nn_model函数中的参数n_h和n_input改为5,如下所示:
```
parameters = nn_model(X, Y, n_h=5, n_input=5, n_output=1, num_iterations=75000, print_cost=True)
```
同时,需要修改X的维数,将其改为(5, m),如下所示:
```
X = data_set.iloc[:, 0:5].values.T.reshape(5, -1)
```
其他部分的代码保持不变即可。需要注意的是,如果将隐藏层神经元数改为5,可能会导致模型的性能下降,需要根据具体情况进行调整。
erode_hsv = cv2.erode(hsv, None, iterations=2)
这行代码使用了 OpenCV 库中的函数 `cv2.erode()`,用于对图像进行腐蚀操作。其中,`hsv` 是输入的图像,`None` 表示没有使用自定义的卷积核,`iterations=2` 表示进行两次腐蚀操作。腐蚀操作可以将图像中的小斑点或噪点去除,从而提高后续图像处理算法的准确性。在进行腐蚀操作之前,需要将图像转换为 HSV 颜色空间。
相关推荐
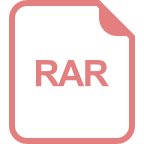













