visualization_msgs::MarkerArray
时间: 2023-08-28 19:07:22 浏览: 56
`visualization_msgs::MarkerArray` 是ROS中的一个消息类型,用于在RViz中显示多个标记(marker)。它是 `visualization_msgs::Marker` 消息的数组,每个 `visualization_msgs::Marker` 对象都描述了一个标记的属性和位置。通过使用 `visualization_msgs::MarkerArray`,我们可以在RViz中同时显示多个标记,例如多个点、线或箭头。
相关问题
visualization_msgs::MarkerArray markers;示例程序
以下是一个简单的示例程序,使用 `visualization_msgs::MarkerArray` 发布多个可视化标记:
```
#include <ros/ros.h>
#include <visualization_msgs/MarkerArray.h>
int main(int argc, char** argv)
{
ros::init(argc, argv, "marker_array_publisher");
ros::NodeHandle nh;
ros::Publisher marker_pub = nh.advertise<visualization_msgs::MarkerArray>("markers", 10);
// 创建多个标记
visualization_msgs::Marker marker1, marker2, marker3;
marker1.header.frame_id = "map";
marker1.header.stamp = ros::Time();
marker1.ns = "markers";
marker1.id = 1;
marker1.type = visualization_msgs::Marker::SPHERE;
marker1.action = visualization_msgs::Marker::ADD;
marker1.pose.position.x = 1.0;
marker1.pose.position.y = 2.0;
marker1.pose.position.z = 0.0;
marker1.pose.orientation.x = 0.0;
marker1.pose.orientation.y = 0.0;
marker1.pose.orientation.z = 0.0;
marker1.pose.orientation.w = 1.0;
marker1.scale.x = 0.5;
marker1.scale.y = 0.5;
marker1.scale.z = 0.5;
marker1.color.r = 1.0;
marker1.color.g = 0.0;
marker1.color.b = 0.0;
marker1.color.a = 1.0;
marker1.lifetime = ros::Duration();
marker2.header.frame_id = "map";
marker2.header.stamp = ros::Time();
marker2.ns = "markers";
marker2.id = 2;
marker2.type = visualization_msgs::Marker::CYLINDER;
marker2.action = visualization_msgs::Marker::ADD;
marker2.pose.position.x = 3.0;
marker2.pose.position.y = 4.0;
marker2.pose.position.z = 0.0;
marker2.pose.orientation.x = 0.0;
marker2.pose.orientation.y = 0.0;
marker2.pose.orientation.z = 0.0;
marker2.pose.orientation.w = 1.0;
marker2.scale.x = 0.5;
marker2.scale.y = 0.5;
marker2.scale.z = 1.0;
marker2.color.r = 0.0;
marker2.color.g = 1.0;
marker2.color.b = 0.0;
marker2.color.a = 1.0;
marker2.lifetime = ros::Duration();
marker3.header.frame_id = "map";
marker3.header.stamp = ros::Time();
marker3.ns = "markers";
marker3.id = 3;
marker3.type = visualization_msgs::Marker::ARROW;
marker3.action = visualization_msgs::Marker::ADD;
marker3.pose.position.x = 5.0;
marker3.pose.position.y = 6.0;
marker3.pose.position.z = 0.0;
marker3.pose.orientation.x = 0.0;
marker3.pose.orientation.y = 0.0;
marker3.pose.orientation.z = 0.0;
marker3.pose.orientation.w = 1.0;
marker3.scale.x = 1.0;
marker3.scale.y = 0.2;
marker3.scale.z = 0.2;
marker3.color.r = 0.0;
marker3.color.g = 0.0;
marker3.color.b = 1.0;
marker3.color.a = 1.0;
marker3.lifetime = ros::Duration();
// 把所有的标记打包到单个 MarkerArray 消息中
visualization_msgs::MarkerArray markers;
markers.markers.push_back(marker1);
markers.markers.push_back(marker2);
markers.markers.push_back(marker3);
// 发布 MarkerArray 消息
while (ros::ok())
{
marker_pub.publish(markers);
ros::spinOnce();
ros::Duration(1).sleep();
}
return 0;
}
```
在这个示例程序中,我们定义了三个不同类型的标记:一个球体,一个圆柱体和一个箭头。每个标记都有其自己的位置,方向,大小和颜色。在程序的最后,我们把这些标记打包到一个单独的 `visualization_msgs::MarkerArray` 消息中,并在一个循环中不断发布它们,每秒发布一次。
Displays visualization_msgs::MarkerArray messages without presuming the topic name ends in "_array"
To display visualization_msgs::MarkerArray messages without presuming the topic name ends in "_array", you can use the rviz MarkerArray display plugin. Here are the steps to do it:
1. Launch rviz:
```
rosrun rviz rviz
```
2. Add a MarkerArray display plugin to the rviz window:
- Click the "Add" button on the left panel.
- Select "MarkerArray" from the list of display types.
- Click "OK".
3. Configure the MarkerArray display plugin:
- In the "Topic" field, enter the name of the topic that publishes the MarkerArray messages.
- If needed, adjust the other parameters such as "Queue Size", "Frame" and "Namespace".
- Click "OK".
4. View the MarkerArray messages:
- The MarkerArray messages should now be displayed in the rviz window.
- You can adjust the display properties such as color, size, shape, etc. by using the "Properties" panel on the right.
Note that the MarkerArray messages should follow the format specified in the visualization_msgs/MarkerArray message type, regardless of the topic name.
相关推荐
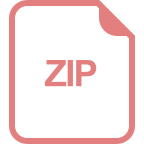
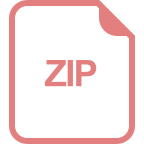











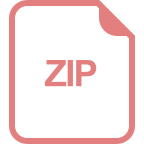