使用多线程并行计算来加速warpAffine并带参数WARP_INVERSE_MAP C++例程
时间: 2023-09-11 15:09:22 浏览: 88
以下是一个使用多线程并行计算来加速warpAffine并带参数WARP_INVERSE_MAP的C++例程:
```cpp
#include <opencv2/opencv.hpp>
#include <thread>
using namespace cv;
void warpAffineParallel(const Mat& src, Mat& dst, const Mat& M, int flags, int start_row, int end_row) {
Mat dst_row = dst.rowRange(start_row, end_row);
warpAffine(src, dst_row, M, dst_row.size(), flags | WARP_INVERSE_MAP);
}
void warpAffineParallelWrapper(const Mat& src, Mat& dst, const Mat& M, int flags, int num_threads) {
int rows_per_thread = dst.rows / num_threads;
std::vector<std::thread> threads;
for (int i = 0; i < num_threads - 1; ++i) {
threads.emplace_back(warpAffineParallel, std::ref(src), std::ref(dst), std::ref(M), flags, i * rows_per_thread, (i + 1) * rows_per_thread);
}
threads.emplace_back(warpAffineParallel, std::ref(src), std::ref(dst), std::ref(M), flags, (num_threads - 1) * rows_per_thread, dst.rows);
for (auto& thread : threads) {
thread.join();
}
}
int main() {
Mat src = imread("input.jpg");
Mat M = getRotationMatrix2D(Point(src.cols / 2, src.rows / 2), 30, 1);
Mat dst(src.size(), src.type());
int num_threads = std::thread::hardware_concurrency();
warpAffineParallelWrapper(src, dst, M, INTER_LINEAR, num_threads);
imshow("input", src);
imshow("output", dst);
waitKey(0);
return 0;
}
```
该代码使用`warpAffineParallelWrapper`函数来将图像分成若干个块,然后使用多个线程并行计算warpAffine。`warpAffineParallel`函数是一个单独的线程函数,它接收源图像、目标图像、变换矩阵、标志以及当前处理的行范围。在`warpAffineParallelWrapper`函数中,我们使用`std::thread::hardware_concurrency()`获取系统的线程数,并将每个线程分配给每个块。最后,我们等待所有线程完成,然后显示输出图像。
请注意,该代码仅用于演示多线程并行计算如何加速warpAffine,并带参数WARP_INVERSE_MAP,实际上并不适合在所有情况下使用。在某些情况下,使用多线程可能会降低性能,因为线程管理和调度开销可能会超过并行计算的好处。在实际应用中,您需要根据具体情况进行测试和优化。
阅读全文
相关推荐
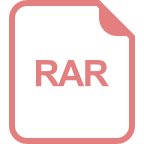
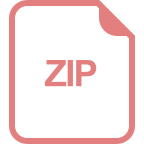











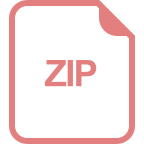
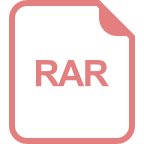
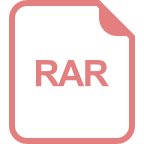
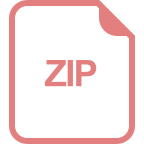
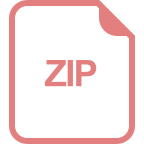