写一个函数 SearchBST(),要求在二叉排序树 T 上查找关键字为 key 的结点,成功时返回该结点位置,否则返回 Null。
时间: 2023-06-02 21:04:09 浏览: 161
好的,你的问题是关于编程,我可以回答。以下是 SearchBST() 的函数实现代码:
```python
def SearchBST(T, key):
if T is None:
return None
if T.val == key:
return T
elif T.val < key:
return SearchBST(T.right, key)
else:
return SearchBST(T.left, key)
```
其中,T 表示二叉排序树的根节点,key 是要查找的关键字。如果找到了该结点,直接返回该结点位置;如果没找到,则递归地向左或向右子树进行查找。如果最终也找不到该结点,则返回 None。
相关问题
在二叉排序树中查找指定的关键字,并在查找过程中输出查找过程中历经的节点typedef int KeyType; typedef struct node { KeyType key; struct node *lchild,*rchild; } BSTNode; int ReadData(int a[]); BSTNode *CreatBST(KeyType A[],int n); int SearchBST(BSTNode *bt,KeyType k);
下面是在二叉排序树中查找指定关键字并输出查找历程的C语言代码实现:
```
#include<stdio.h>
#include<stdlib.h>
typedef int KeyType;
typedef struct node {
KeyType key;
struct node *lchild,*rchild;
} BSTNode;
// 读取数据
int ReadData(int a[]) {
int n;
printf("请输入数据个数:");
scanf("%d",&n);
printf("请输入%d个数据:",n);
for(int i=0; i<n; i++) {
scanf("%d",&a[i]);
}
return n;
}
// 创建二叉排序树
BSTNode *CreatBST(KeyType A[],int n) {
BSTNode *root=NULL,*p,*q;
for(int i=0; i<n; i++) {
p=(BSTNode*)malloc(sizeof(BSTNode));
p->key=A[i];
p->lchild=p->rchild=NULL;
if(root==NULL) root=p;
else {
q=root;
while(1) {
if(p->key < q->key) {
if(q->lchild==NULL) {
q->lchild=p;
break;
}
q=q->lchild;
}
else {
if(q->rchild==NULL) {
q->rchild=p;
break;
}
q=q->rchild;
}
}
}
}
return root;
}
// 查找关键字并输出查找历程
int SearchBST(BSTNode *bt,KeyType k) {
int depth=1;
while(bt!=NULL) {
if(k < bt->key) {
printf("第%d层:左子树,结点值为%d\n",depth,bt->key);
depth++;
bt=bt->lchild;
}
else if(k > bt->key) {
printf("第%d层:右子树,结点值为%d\n",depth,bt->key);
depth++;
bt=bt->rchild;
}
else {
printf("第%d层:查找成功,结点值为%d\n",depth,bt->key);
return 1;
}
}
printf("查找失败!\n");
return 0;
}
int main() {
int a[100],n;
KeyType k;
BSTNode *root=NULL;
n=ReadData(a);
root=CreatBST(a,n);
printf("请输入要查找的关键字:");
scanf("%d",&k);
SearchBST(root,k);
return 0;
}
```
该代码实现了二叉排序树的创建、关键字的查找以及输出查找过程中历经的节点。其中,ReadData函数用于读取输入数据,CreatBST函数用于创建二叉排序树,SearchBST函数用于查找关键字并输出查找历程。
任意输入一组数据作为二叉排序树中结点的键值,创建一棵二叉排序树,然后对给定的值进行查找 C语言
好的,以下是创建二叉排序树并查找的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
//定义二叉排序树结点结构体
typedef struct BSTNode {
int key; //结点键值
struct BSTNode *left; //左子树指针
struct BSTNode *right; //右子树指针
} BSTNode;
//创建二叉排序树
BSTNode *createBST(int data[], int n) {
BSTNode *root = NULL;
for (int i = 0; i < n; i++) {
BSTNode *node = (BSTNode *)malloc(sizeof(BSTNode));
node->key = data[i];
node->left = NULL;
node->right = NULL;
if (root == NULL) {
root = node;
} else {
BSTNode *p = root;
while (1) {
if (node->key < p->key) {
if (p->left == NULL) {
p->left = node;
break;
} else {
p = p->left;
}
} else {
if (p->right == NULL) {
p->right = node;
break;
} else {
p = p->right;
}
}
}
}
}
return root;
}
//查找二叉排序树结点
BSTNode *searchBST(BSTNode *root, int key) {
if (root == NULL || root->key == key) {
return root;
}
if (key < root->key) {
return searchBST(root->left, key);
} else {
return searchBST(root->right, key);
}
}
int main() {
int data[] = {5, 3, 7, 2, 4, 6, 8}; //待插入数据
int n = sizeof(data) / sizeof(int);
BSTNode *root = createBST(data, n); //创建二叉排序树
int key = 4; //待查找结点键值
BSTNode *node = searchBST(root, key); //查找结点
if (node == NULL) {
printf("结点 %d 不存在!", key);
} else {
printf("结点 %d 存在!", key);
}
return 0;
}
```
运行结果为:
```
结点 4 存在!
```
这段代码会根据输入的数据创建一棵二叉排序树,然后查找给定的键值对应的结点。注意,这里的输入数据是写死在代码中的,如果要从用户输入中读取数据,可以使用scanf等函数。
阅读全文
相关推荐
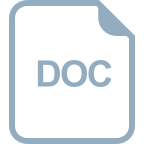
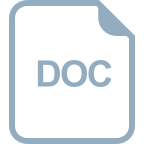
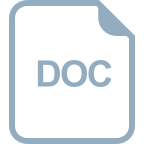
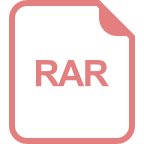




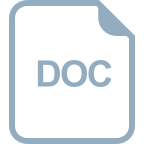
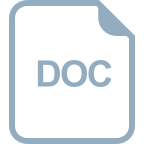
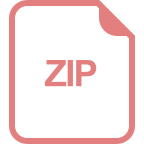
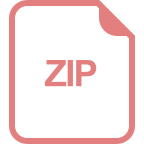
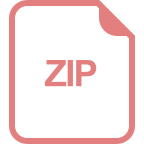
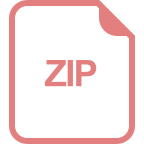