帮我写一段代码,实现在osgearth中显示中文的功能
时间: 2024-05-04 16:22:27 浏览: 203
在osgEarth中显示中文需要使用到中文字体文件,以及将中文字符串转换为utf-8编码。下面是一个简单的示例代码,可以实现在osgEarth中显示中文:
```cpp
#include <osgEarth/MapNode>
#include <osgEarth/AnnotationNode>
#include <osgEarth/Registry>
#include <osgEarthAnnotation/PlaceNode>
int main( int argc, char** argv )
{
// 初始化osgEarth
osg::ref_ptr<osgEarth::MapNode> mapNode = osgEarth::MapNode::create();
osg::ref_ptr<osgEarth::AnnotationNode> annoNode = new osgEarth::AnnotationNode(mapNode->getMap());
// 创建中文字体文件
osg::ref_ptr<osgText::Font> font = osgText::readFontFile("simhei.ttf");
// 创建中文字符串
std::string str = "你好,世界!";
std::wstring_convert<std::codecvt_utf8<wchar_t>> conv;
std::wstring wstr = conv.from_bytes(str);
// 将中文字符串转换为utf-8编码
std::string utf8str;
for (wchar_t ch : wstr)
{
if (ch < 0x80)
{
utf8str.push_back(static_cast<char>(ch));
}
else if (ch < 0x800)
{
utf8str.push_back(static_cast<char>((ch >> 6) | 0xC0));
utf8str.push_back(static_cast<char>((ch & 0x3F) | 0x80));
}
else
{
utf8str.push_back(static_cast<char>((ch >> 12) | 0xE0));
utf8str.push_back(static_cast<char>(((ch >> 6) & 0x3F) | 0x80));
utf8str.push_back(static_cast<char>((ch & 0x3F) | 0x80));
}
}
// 创建PlaceNode并显示中文字符串
osg::ref_ptr<osgEarth::Annotation::PlaceNode> placeNode = new osgEarth::Annotation::PlaceNode(mapNode->getMap(), osgEarth::GeoPoint(), utf8str, font.get());
annoNode->addChild(placeNode);
// 显示场景
osgViewer::Viewer viewer;
viewer.setSceneData(annoNode.get());
return viewer.run();
}
```
在上面的代码中,我们使用了 `std::wstring_convert` 类将中文字符串转换为 `std::string` 类型的 utf-8 编码。然后创建了一个 `PlaceNode` 对象,并将其添加到 `AnnotationNode` 子节点中,从而实现在osgEarth中显示中文的功能。
阅读全文
相关推荐
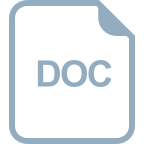
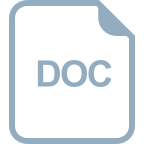
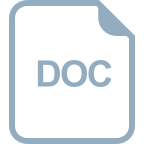















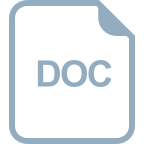