unity中各种集合类,如 List、Dictionary、Queue ,链表,队列,堆,帮我写一个案例把unity中各种集合都使用一次
时间: 2024-03-27 11:36:29 浏览: 12
好的,我可以帮你写一个案例,使用 List、Dictionary、Queue、LinkedList 和 Heap 等集合类。
首先,我们创建一个名为 CollectionExample 的脚本,并在其中声明以下集合变量:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CollectionExample : MonoBehaviour
{
// List
public List<string> namesList;
// Dictionary
public Dictionary<string, int> scoresDict;
// Queue
public Queue<int> numbersQueue;
// LinkedList
public LinkedList<float> weightsLinkedList;
// Heap
public SimpleHeap<int> numbersHeap;
// Start is called before the first frame update
void Start()
{
// 初始化 List
namesList = new List<string>();
namesList.Add("Tom");
namesList.Add("Jerry");
namesList.Add("Mickey");
// 初始化 Dictionary
scoresDict = new Dictionary<string, int>();
scoresDict.Add("Tom", 80);
scoresDict.Add("Jerry", 90);
scoresDict.Add("Mickey", 85);
// 初始化 Queue
numbersQueue = new Queue<int>();
numbersQueue.Enqueue(1);
numbersQueue.Enqueue(2);
numbersQueue.Enqueue(3);
// 初始化 LinkedList
weightsLinkedList = new LinkedList<float>();
weightsLinkedList.AddFirst(60.5f);
weightsLinkedList.AddLast(70.2f);
LinkedListNode<float> node = weightsLinkedList.First;
while (node != null)
{
Debug.Log("Weight: " + node.Value);
node = node.Next;
}
// 初始化 Heap
numbersHeap = new SimpleHeap<int>();
numbersHeap.Insert(10);
numbersHeap.Insert(20);
numbersHeap.Insert(5);
numbersHeap.Insert(25);
Debug.Log("Heap Top: " + numbersHeap.Top());
}
// Update is called once per frame
void Update()
{
// 遍历 List
foreach (string name in namesList)
{
Debug.Log("Name: " + name);
}
// 遍历 Dictionary
foreach (KeyValuePair<string, int> kvp in scoresDict)
{
Debug.Log("Name: " + kvp.Key + ", Score: " + kvp.Value);
}
// 使用 Queue
if (numbersQueue.Count > 0)
{
int num = numbersQueue.Dequeue();
Debug.Log("Number: " + num);
}
// 使用 LinkedList
LinkedListNode<float> node = weightsLinkedList.First;
while (node != null)
{
Debug.Log("Weight: " + node.Value);
node = node.Next;
}
// 使用 Heap
Debug.Log("Heap Top: " + numbersHeap.Top());
}
}
```
在 Start 方法中,我们分别初始化了 List、Dictionary 和 Queue,并添加了一些元素。同时,我们也初始化了 LinkedList 和 Heap,并插入了一些元素。
在 Update 方法中,我们分别使用了 foreach 循环遍历了 List 和 Dictionary,以及使用了 Queue 的 Dequeue 方法取出了队首元素。我们也使用了 LinkedList 的遍历操作,并使用 Heap 的 Top 方法获取堆顶元素。
现在,我们可以将 CollectionExample 脚本绑定到场景中的任意一个 GameObject 上,然后运行游戏,就可以看到各种集合的使用效果了。
相关推荐
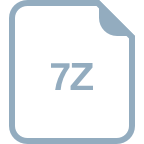
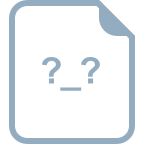














