float rotateRate = Mathf.Clamp(handleForce / cp.bendForce, 0f, 1.0f);
时间: 2024-04-17 17:22:57 浏览: 9
这行代码计算了一个名为 `rotateRate` 的浮点数,其值是 `handleForce` 除以控制点 `cp` 的弯曲力(`bendForce`)的比值,并将结果限制在 0 到 1 之间。
`handleForce` 是一个表示处理力的浮点数,而 `cp.bendForce` 是控制点 `cp` 的弯曲力属性。
通过将 `handleForce` 除以 `cp.bendForce`,我们得到一个表示处理力与弯曲力之间比值的浮点数。然后,使用 `Mathf.Clamp` 函数将结果限制在 0 到 1 的范围内,确保 `rotateRate` 的值不会超出这个范围。
这样的操作可能是为了根据处理力和弯曲力的比值来确定旋转率,在后续的计算或操作中使用该值来控制物体的旋转速度或角度变化。具体的应用和目的,还需要根据代码中其他部分的实现来确定。
相关问题
var pixelUV = raycastHit.textureCoord; //计算笔刷所覆盖的区域 var PuX = Mathf.FloorToInt(pixelUV.x * controlTex.width); var PuY = Mathf.FloorToInt(pixelUV.y * controlTex.height); var x = Mathf.Clamp(PuX - brushSizeInModel / 2, 0, controlTex.width - 1); var y = Mathf.Clamp(PuY - brushSizeInModel / 2, 0, controlTex.height - 1); var width = Mathf.Clamp((PuX + brushSizeInModel / 2), 0, controlTex.width) - x; var height = Mathf.Clamp((PuY + brushSizeInModel / 2), 0, controlTex.height) - y; Color[] terrainBay = controlTex.GetPixels(x, y, width, height, 0);//获取Control贴图被笔刷所覆盖的区域的颜色 Texture2D TBrush = brushTex[selBrush] as Texture2D;//获取笔刷性状贴图 var brushAlpha = new float[brushSizeInModel * brushSizeInModel];//笔刷透明度 //根据笔刷贴图计算笔刷的透明度 for (var i = 0; i < brushSizeInModel; i++) { for (var j = 0; j < brushSizeInModel; j++) { brushAlpha[j * brushSizeInModel + i] = TBrush.GetPixelBilinear(((float)i) / brushSizeInModel, ((float)j) / brushSizeInModel).a; } } //计算绘制后的颜色 for (var i = 0; i < height; i++) { for (var j = 0; j < width; j++) { var index = (i * width) + j; var Stronger = brushAlpha[Mathf.Clamp((y + i) - (PuY - brushSizeInModel / 2), 0, brushSizeInModel - 1) * brushSizeInModel + Mathf.Clamp((x + j) - (PuX - brushSizeInModel / 2), 0, brushSizeInModel - 1)] * brushStronger; terrainBay[index] = Color.Lerp(terrainBay[index], targetColor, Stronger); } } Undo.RegisterCompleteObjectUndo(controlTex, "meshPaint");//保存历史记录以便撤销 controlTex.SetPixels(x, y, width, height, terrainBay, 0);/这段代码生成的位置是从右上角开始的如何修改成左下角
要将代码生成的位置从右上角改为左下角,需要对获取Control贴图被笔刷所覆盖的区域的颜色和计算绘制后的颜色的部分进行修改。
具体来说,需要将以下代码:
```
var x = Mathf.Clamp(PuX - brushSizeInModel / 2, 0, controlTex.width - 1);
var y = Mathf.Clamp(PuY - brushSizeInModel / 2, 0, controlTex.height - 1);
var width = Mathf.Clamp((PuX + brushSizeInModel / 2), 0, controlTex.width) - x;
var height = Mathf.Clamp((PuY + brushSizeInModel / 2), 0, controlTex.height) - y;
...
var Stronger = brushAlpha[Mathf.Clamp((y + i) - (PuY - brushSizeInModel / 2), 0, brushSizeInModel - 1) * brushSizeInModel + Mathf.Clamp((x + j) - (PuX - brushSizeInModel / 2), 0, brushSizeInModel - 1)] * brushStronger;
terrainBay[index] = Color.Lerp(terrainBay[index], targetColor, Stronger);
```
修改为:
```
var x = Mathf.Clamp(PuX - brushSizeInModel / 2, 0, controlTex.width - 1);
var y = Mathf.Clamp(controlTex.height - (PuY + brushSizeInModel / 2), 0, controlTex.height - 1);
var width = Mathf.Clamp((PuX + brushSizeInModel / 2), 0, controlTex.width) - x;
var height = Mathf.Clamp((PuY + brushSizeInModel / 2), 0, controlTex.height) - y;
...
var Stronger = brushAlpha[Mathf.Clamp((controlTex.height - (y + i)) - (PuY - brushSizeInModel / 2), 0, brushSizeInModel - 1) * brushSizeInModel + Mathf.Clamp((x + j) - (PuX - brushSizeInModel / 2), 0, brushSizeInModel - 1)] * brushStronger;
terrainBay[index] = Color.Lerp(terrainBay[index], targetColor, Stronger);
```
这样就可以将代码生成的位置从右上角改为左下角。
using System.Collections; using System.Collections.Generic; using UnityEngine; public class FollowTouch : MonoBehaviour { private Vector3 initialPosition; void Start() { initialPosition = transform.position; } void Update() { if (Input.touchCount > 0) { Touch touch = Input.GetTouch(0); Vector3 touchPosition = Camera.main.ScreenToWorldPoint(touch.position); touchPosition.z = 0f; transform.position = touchPosition; } float clampedX = Mathf.Clamp(transform.position.x, -5f, 5f); float clampedY = Mathf.Clamp(transform.position.y, -3f, 3f); transform.position = new Vector3(clampedX, clampedY, transform.position.z); } }上述代码运行后位置不对,拖动没有效果
根据你提供的代码,初步判断可能是因为物体的初始位置和拖动后的位置没有对齐导致的。你可以尝试在Update函数中添加一个判断条件,只有当触摸点触碰到物体时才继续执行拖动操作。修改后的代码如下:
```
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FollowTouch : MonoBehaviour
{
private Vector3 initialPosition;
void Start()
{
initialPosition = transform.position;
}
void Update()
{
if (Input.touchCount > 0)
{
Touch touch = Input.GetTouch(0);
Vector3 touchPosition = Camera.main.ScreenToWorldPoint(touch.position);
touchPosition.z = 0f;
// 判断触摸点是否在物体上
Collider2D collider = Physics2D.OverlapPoint(touchPosition);
if (collider != null && collider.gameObject == gameObject)
{
transform.position = touchPosition;
}
}
float clampedX = Mathf.Clamp(transform.position.x, -5f, 5f);
float clampedY = Mathf.Clamp(transform.position.y, -3f, 3f);
transform.position = new Vector3(clampedX, clampedY, transform.position.z);
}
}
```
另外,你还可以在Unity编辑器中检查物体的碰撞器组件是否已经添加,并且是否正确设置了碰撞器的形状和大小。
相关推荐
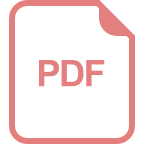
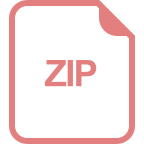












