编写程序,使用fork()产生两个子进程,首先显示一下两个子进程及父进 程的进程标识符,然后让父进程显示1~26个数字,子进程1显示26个大写字母, 子进程2显示26个小写字母。让大小写字母及数字是混合交错输出的。
时间: 2024-03-02 07:49:34 浏览: 134
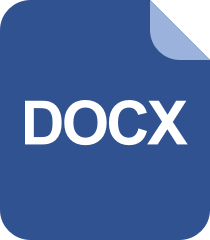
fork创建多个子进程

好的,这是一个比较基础的进程间通信问题,可以使用管道来实现进程之间的通信,以下是一个示例程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <ctype.h>
#include <sys/wait.h>
#define BUFFER_SIZE 100
int main()
{
pid_t pid1, pid2;
int fd1[2], fd2[2];
char buf[BUFFER_SIZE];
int i;
if (pipe(fd1) < 0 || pipe(fd2) < 0) {
perror("pipe error");
exit(EXIT_FAILURE);
}
if ((pid1 = fork()) < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid1 == 0) {
// child process 1
close(fd1[0]); // close read end
close(fd2[0]);
close(fd2[1]);
for (i = 0; i < 26; i++) {
buf[i] = 'A' + i;
}
write(fd1[1], buf, 26);
close(fd1[1]);
exit(EXIT_SUCCESS);
}
if ((pid2 = fork()) < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid2 == 0) {
// child process 2
close(fd1[0]);
close(fd1[1]);
close(fd2[0]);
for (i = 0; i < 26; i++) {
buf[i] = 'a' + i;
}
write(fd2[1], buf, 26);
close(fd2[1]);
exit(EXIT_SUCCESS);
}
// parent process
close(fd1[1]);
close(fd2[1]);
for (i = 0; i < 26; i++) {
int n = read(fd1[0], buf, 1);
if (n > 0) {
printf("%c", buf[0]);
}
n = read(fd2[0], buf, 1);
if (n > 0) {
printf("%c", buf[0]);
}
printf("%d ", i+1);
}
printf("\n");
close(fd1[0]);
close(fd2[0]);
wait(NULL);
wait(NULL);
return 0;
}
```
该程序使用了两个管道,一个用于父进程与子进程1之间的通信,另一个用于父进程与子进程2之间的通信。子进程1和子进程2分别向它们对应的管道中写入字母表中的大写字母和小写字母,父进程从两个管道中读取数据并输出。由于管道是一个字节一个字节地传递数据的,因此需要对每个字节进行读写操作。
阅读全文
相关推荐
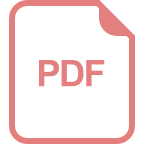
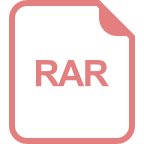















