用easyx写一个俄罗斯方块游戏
时间: 2023-12-21 10:07:30 浏览: 85
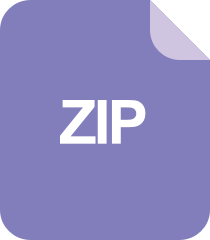
用easyX实现的简单俄罗斯方块小游戏
好的,下面是一个简单的俄罗斯方块游戏代码示例,使用了 EasyX 图形库:
```cpp
#include <graphics.h>
#include <conio.h>
#include <time.h>
#define ROWS 20
#define COLS 10
#define BLOCK_SIZE 30
int score = 0;
int speed = 500;
int blocks[7][4][4] = {
// I
{
{ 0, 1, 0, 0 },
{ 0, 1, 0, 0 },
{ 0, 1, 0, 0 },
{ 0, 1, 0, 0 }
},
// J
{
{ 0, 1, 0, 0 },
{ 0, 1, 1, 0 },
{ 0, 0, 1, 0 },
{ 0, 0, 0, 0 }
},
// L
{
{ 0, 0, 1, 0 },
{ 0, 0, 1, 0 },
{ 0, 1, 1, 0 },
{ 0, 0, 0, 0 }
},
// O
{
{ 0, 0, 0, 0 },
{ 0, 1, 1, 0 },
{ 0, 1, 1, 0 },
{ 0, 0, 0, 0 }
},
// S
{
{ 0, 0, 0, 0 },
{ 0, 0, 1, 1 },
{ 0, 1, 1, 0 },
{ 0, 0, 0, 0 }
},
// T
{
{ 0, 0, 0, 0 },
{ 0, 1, 1, 1 },
{ 0, 0, 1, 0 },
{ 0, 0, 0, 0 }
},
// Z
{
{ 0, 0, 0, 0 },
{ 0, 1, 1, 0 },
{ 0, 0, 1, 1 },
{ 0, 0, 0, 0 }
}
};
int board[ROWS][COLS] = { 0 };
struct Block {
int x, y;
int type;
int dir;
};
void draw_block(int x, int y, int type) {
setfillcolor(RGB(255, 0, 0));
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (blocks[type][i][j]) {
solidrectangle((x + j) * BLOCK_SIZE, (y + i) * BLOCK_SIZE, (x + j + 1) * BLOCK_SIZE, (y + i + 1) * BLOCK_SIZE);
}
}
}
}
void draw_board() {
setfillcolor(RGB(0, 0, 0));
solidrectangle(0, 0, COLS * BLOCK_SIZE, ROWS * BLOCK_SIZE);
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (board[i][j]) {
setfillcolor(RGB(255, 255, 255));
solidrectangle(j * BLOCK_SIZE, i * BLOCK_SIZE, (j + 1) * BLOCK_SIZE, (i + 1) * BLOCK_SIZE);
}
}
}
}
bool check_collision(int x, int y, int type, int dir) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (blocks[type][i][j]) {
int rx = x + j;
int ry = y + i;
if (rx < 0 || rx >= COLS || ry >= ROWS || (ry >= 0 && board[ry][rx])) {
return true;
}
}
}
}
return false;
}
void add_block_to_board(Block& block) {
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
if (blocks[block.type][i][j]) {
int rx = block.x + j;
int ry = block.y + i;
if (rx >= 0 && rx < COLS && ry >= 0 && ry < ROWS) {
board[ry][rx] = 1;
}
}
}
}
}
bool check_full_line(int row) {
for (int i = 0; i < COLS; i++) {
if (!board[row][i]) {
return false;
}
}
return true;
}
void remove_full_lines() {
int count = 0;
for (int i = ROWS - 1; i >= 0; i--) {
if (check_full_line(i)) {
count++;
for (int j = i; j > 0; j--) {
for (int k = 0; k < COLS; k++) {
board[j][k] = board[j - 1][k];
}
}
for (int k = 0; k < COLS; k++) {
board[0][k] = 0;
}
i++;
}
}
score += count * 100;
}
void game_over() {
settextstyle(60, 0, _T("Consolas"));
settextcolor(RGB(255, 0, 0));
outtextxy(100, 200, _T("GAME OVER"));
outtextxy(150, 300, _T("Score: "));
TCHAR str[16];
_stprintf_s(str, _T("%d"), score);
outtextxy(400, 300, str);
_getch();
exit(0);
}
void run() {
Block block = { COLS / 2 - 2, 0, rand() % 7, 0 };
while (true) {
draw_board();
draw_block(block.x, block.y, block.type);
Sleep(speed);
if (check_collision(block.x, block.y + 1, block.type, block.dir)) {
add_block_to_board(block);
remove_full_lines();
if (check_collision(COLS / 2 - 2, 0, rand() % 7, 0)) {
game_over();
}
block = { COLS / 2 - 2, 0, rand() % 7, 0 };
}
else {
block.y++;
}
if (_kbhit()) {
char ch = _getch();
switch (ch) {
case 'a':
if (!check_collision(block.x - 1, block.y, block.type, block.dir)) {
block.x--;
}
break;
case 'd':
if (!check_collision(block.x + 1, block.y, block.type, block.dir)) {
block.x++;
}
break;
case 'w':
if (!check_collision(block.x, block.y, block.type, (block.dir + 1) % 4)) {
block.dir = (block.dir + 1) % 4;
}
break;
case 's':
speed = 50;
break;
}
}
else {
speed = 500;
}
}
}
int main() {
initgraph(COLS * BLOCK_SIZE, ROWS * BLOCK_SIZE);
srand((unsigned int)time(NULL));
run();
return 0;
}
```
这个示例代码中,使用了 EasyX 图形库来绘制界面,并且实现了基本的游戏逻辑,包括方块的绘制、移动、旋转,以及行的消除等。
阅读全文
相关推荐
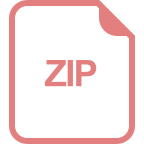
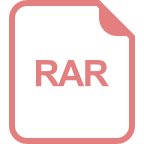















