Bellman ford算法,c++代码
时间: 2023-11-10 09:01:00 浏览: 92
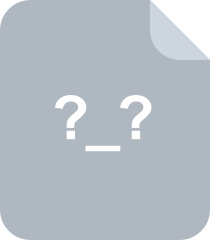
bellman ford 算法

Bellman-Ford算法是一种用于解决单源最短路径问题的算法,它可以处理边权值为负数的情况。下面是C语言实现的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <limits.h>
#define MAX_VERTICES 1000
#define MAX_EDGES 2000
typedef struct Edge {
int source;
int destination;
int weight;
} Edge;
Edge edges[MAX_EDGES];
int distances[MAX_VERTICES];
int parents[MAX_VERTICES];
int num_vertices;
int num_edges;
void bellman_ford(int source) {
// 初始化距离数组和前驱数组
for (int i = 0; i < num_vertices; i++) {
distances[i] = INT_MAX;
parents[i] = -1;
}
distances[source] = 0;
// 对每条边进行V-1次松弛操作
for (int i = 1; i <= num_vertices - 1; i++) {
for (int j = 0; j < num_edges; j++) {
Edge edge = edges[j];
int u = edge.source;
int v = edge.destination;
int w = edge.weight;
if (distances[u] != INT_MAX && distances[u] + w < distances[v]) {
distances[v] = distances[u] + w;
parents[v] = u;
}
}
}
// 检测负权环
for (int i = 0; i < num_edges; i++) {
Edge edge = edges[i];
int u = edge.source;
int v = edge.destination;
int w = edge.weight;
if (distances[u] != INT_MAX && distances[u] + w < distances[v]) {
printf("Graph contains a negative-weight cycle\n");
return;
}
}
// 输出结果
for (int i = 0; i < num_vertices; i++) {
printf("Vertex %d, distance = %d, parent = %d\n", i, distances[i], parents[i]);
}
}
int main() {
// 从文件读取图的信息
FILE *fp = fopen("graph.txt", "r");
fscanf(fp, "%d %d", &num_vertices, &num_edges);
for (int i = 0; i < num_edges; i++) {
fscanf(fp, "%d %d %d", &edges[i].source, &edges[i].destination, &edges[i].weight);
}
fclose(fp);
// 执行Bellman-Ford算法
bellman_ford(0);
return 0;
}
```
其中,`num_vertices`和`num_edges`分别表示图中的顶点数和边数,`distances`和`parents`分别表示每个顶点到源点的最短距离和最短路径上的前驱顶点,`edges`数组保存了每条边的起点、终点和权值。`bellman_ford`函数实现了Bellman-Ford算法的主要逻辑,首先对距离数组和前驱数组进行初始化,然后对每条边进行V-1次松弛操作,最后检测负权环并输出结果。
阅读全文
相关推荐
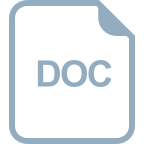
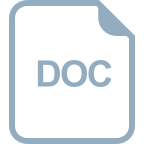

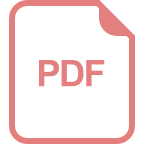
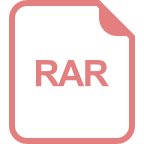
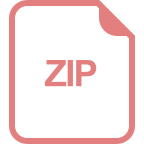
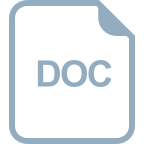
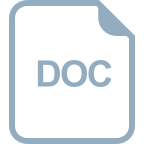
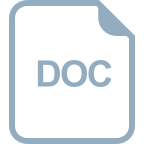
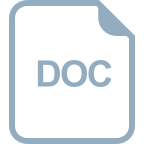
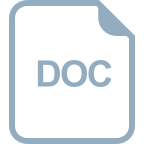



