题要求实现一个函数,实现三元组顺序表表示的稀疏矩阵转置。 函数接口定义: struct tripletable * trans(struct tripletable *t1);
时间: 2023-06-26 10:06:04 浏览: 76
好的,那么我们来一步步实现这个函数。
首先,我们需要定义三元组顺序表的结构体,包括行数、列数、非零元素个数和一个三元组数组。
```c
struct triple {
int row;
int col;
int val;
};
struct tripletable {
int rows;
int cols;
int nums;
struct triple data[MAXSIZE];
};
```
其中,`MAXSIZE` 是数组的最大长度,你可以自己定义一个合适的值。
接下来,我们需要实现 `trans` 函数。这个函数接受一个三元组顺序表 `t1` 作为参数,返回一个新的三元组顺序表,表示 `t1` 的转置矩阵。
```c
struct tripletable * trans(struct tripletable *t1) {
struct tripletable *t2;
int *num, *cpot;
int i, j, k;
// 创建一个新的三元组顺序表
t2 = (struct tripletable *) malloc(sizeof(struct tripletable));
if (!t2) {
printf("Memory allocation error\n");
exit(1);
}
t2->rows = t1->cols; // 新表的行数为旧表的列数
t2->cols = t1->rows; // 新表的列数为旧表的行数
t2->nums = t1->nums; // 非零元素个数不变
// 分配两个辅助数组
num = (int *) calloc(t1->cols + 1, sizeof(int));
if (!num) {
printf("Memory allocation error\n");
exit(1);
}
cpot = (int *) calloc(t1->cols + 1, sizeof(int));
if (!cpot) {
printf("Memory allocation error\n");
exit(1);
}
// 统计每一列的非零元素个数
for (i = 1; i <= t1->nums; i++) {
num[t1->data[i].col]++;
}
// 计算每一列的起始位置
cpot[1] = 1;
for (j = 2; j <= t1->cols; j++) {
cpot[j] = cpot[j-1] + num[j-1];
}
// 转置矩阵
for (k = 1; k <= t1->nums; k++) {
j = t1->data[k].col;
i = cpot[j];
t2->data[i].row = t1->data[k].col;
t2->data[i].col = t1->data[k].row;
t2->data[i].val = t1->data[k].val;
cpot[j]++;
}
// 释放辅助数组
free(num);
free(cpot);
return t2;
}
```
这个函数的实现过程,可以分为以下几个步骤:
1. 创建一个新的三元组顺序表 `t2`,行数为 `t1` 的列数,列数为 `t1` 的行数,非零元素个数不变。
2. 分配两个辅助数组 `num` 和 `cpot`,用于统计每一列的非零元素个数和计算每一列的起始位置。
3. 统计每一列的非零元素个数,并计算每一列的起始位置。
4. 转置矩阵,将 `t1` 中的每个三元组转换为 `t2` 中的一个三元组。
5. 释放辅助数组。
最后,需要注意的是,我们在函数中使用了动态内存分配函数 `malloc` 和 `calloc`,需要在适当的时候使用 `free` 函数释放内存,避免内存泄漏。
相关推荐
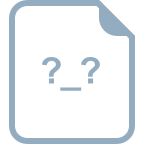
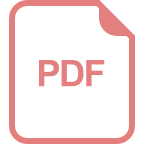
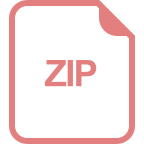














