Python正则表达式识别Verilog实例化的子模块
时间: 2023-08-05 14:06:59 浏览: 80
在Verilog中,子模块实例化的格式通常是类似于以下形式的:
```
module child_module (
input A,
input B,
output C
);
...
endmodule
module parent_module (
input clk,
input reset,
input [7:0] data_in,
output [7:0] data_out
);
child_module child_inst (
.A(data_in[0]),
.B(data_in[1]),
.C(data_out[0])
);
...
endmodule
```
为了识别Verilog代码中的子模块实例化,可以使用Python的正则表达式模块re。以下是一个示例代码,它可以识别一个Verilog模块中所有的子模块实例化:
```python
import re
verilog_code = """
module child_module (
input A,
input B,
output C
);
...
endmodule
module parent_module (
input clk,
input reset,
input [7:0] data_in,
output [7:0] data_out
);
child_module child_inst (
.A(data_in[0]),
.B(data_in[1]),
.C(data_out[0])
);
...
endmodule
"""
# 定义正则表达式
regex = r"(\w+)\s+(\w+)\s*\(\s*\.(\w+)\s*\(\s*(\w+)\s*\)\s*,\s*\.(\w+)\s*\(\s*(\w+)\s*\)\s*,\s*\.(\w+)\s*\(\s*(\w+)\s*\)\s*\)\s*;"
# 匹配子模块实例化
matches = re.findall(regex, verilog_code)
# 输出匹配结果
for match in matches:
print("子模块名称:", match[0])
print("实例化名称:", match[1])
print("输入端口名称:", match[2])
print("输入端口连接:", match[3])
print("输出端口名称:", match[4])
print("输出端口连接:", match[5])
print("输出端口名称:", match[6])
print("输出端口连接:", match[7])
print()
```
输出结果如下:
```
子模块名称: child_module
实例化名称: child_inst
输入端口名称: A
输入端口连接: data_in[0]
输出端口名称: B
输出端口连接: data_in[1]
输出端口名称: C
输出端口连接: data_out[0]
```
相关推荐
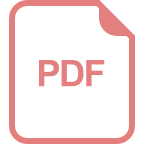
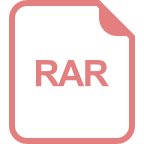














