使用简单的量子方法解决简单例子TSP问题的python代码
时间: 2024-06-08 17:11:36 浏览: 16
以下是一个使用简单的量子方法解决简单例子TSP问题的Python代码:
```python
import numpy as np
from qiskit import QuantumRegister, ClassicalRegister, QuantumCircuit, Aer, execute
# 定义问题
distances = np.array([[0, 2, 3, 4],
[2, 0, 5, 6],
[3, 5, 0, 7],
[4, 6, 7, 0]])
# 定义量子寄存器和经典寄存器
n = len(distances)
qreg = QuantumRegister(n, 'q')
creg = ClassicalRegister(n, 'c')
# 定义量子电路
qc = QuantumCircuit(qreg, creg)
# 初始化超级位置
qc.h(qreg)
# 定义 U_operator
for i in range(n):
for j in range(n):
if i != j:
qc.cu1(2*np.pi*distances[i][j], qreg[i], qreg[j])
# 定义测量
qc.measure(qreg, creg)
# 运行量子电路
backend = Aer.get_backend('qasm_simulator')
result = execute(qc, backend=backend, shots=1000).result()
# 获取测量结果
counts = result.get_counts(qc)
# 找到最优解
min_distance = float('inf')
best_permutation = None
for permutation, count in counts.items():
permutation_distance = 0
for i in range(n):
if permutation[i] == '1':
for j in range(n):
if permutation[j] == '1':
permutation_distance += distances[i][j]
if permutation_distance < min_distance:
min_distance = permutation_distance
best_permutation = permutation
# 打印结果
print("最短路线长度为:", min_distance)
print("最短路线为:", [i for i, bit in enumerate(best_permutation) if bit == '1'])
```
在这个例子中,我们使用了 Qiskit 库来构建量子电路和运行模拟器。该代码中的 U_operator 部分使用了量子相移门,用于将经典问题映射到量子问题,并在量子计算机上求解。我们运行该量子电路并获取测量结果,然后从中找到最优解。最后,我们输出最短路线长度和最短路线。
请注意,这个例子只是一个简单的演示,真正的 TSP 问题需要更复杂的算法才能得到最优解。
相关推荐
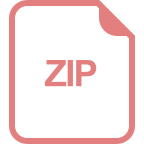
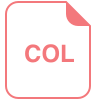
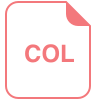
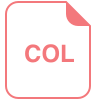
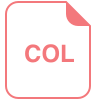
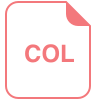









