编写一段C++程序实现:将一个空闲教室分为81排(L1到L81),每排十个座位,教室每排座位从1到N依次编号;将各排座位分给各个申请座位的班级;同一排的座位只能分给同一个班级;先申请的班级先分配座位;根据各个班级的学生人数,分配序号连续的排,下课后一次归还。
时间: 2023-06-18 09:06:59 浏览: 57
以下是一份简单的 C++ 程序,实现了你所需的功能。该程序使用向量(vector)来存储班级和座位信息,使用排序算法对班级按照学生人数从小到大排序,然后按顺序分配座位。请注意,该程序并未进行输入数据的检查,因此需要确保输入数据的正确性。
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct Class {
int id; // 班级编号
int numStudents; // 学生人数
int startRow; // 座位起始行号
int endRow; // 座位结束行号
bool operator<(const Class& other) const {
return numStudents < other.numStudents;
}
};
int main() {
const int numRows = 81; // 总共81排座位
const int numSeatsPerRow = 10; // 每排10个座位
const int numClasses = 5; // 总共5个班级
vector<int> seats(numRows * numSeatsPerRow, 0); // 初始化所有座位为空
vector<Class> classes(numClasses);
classes[0] = { 1, 30 }; // 班级1,30名学生
classes[1] = { 2, 25 }; // 班级2,25名学生
classes[2] = { 3, 20 }; // 班级3,20名学生
classes[3] = { 4, 15 }; // 班级4,15名学生
classes[4] = { 5, 10 }; // 班级5,10名学生
sort(classes.begin(), classes.end()); // 按学生人数排序,从小到大
int currentRow = 0;
for (const auto& cls : classes) {
int numSeats = cls.numStudents;
if (currentRow + numSeats > numRows) {
cout << "Error: Not enough seats for class " << cls.id << endl;
break;
}
cls.startRow = currentRow + 1;
cls.endRow = currentRow + numSeats;
for (int row = currentRow; row < currentRow + numSeats; row++) {
for (int seat = 0; seat < numSeatsPerRow; seat++) {
seats[row * numSeatsPerRow + seat] = cls.id;
}
}
currentRow += numSeats;
}
// 输出座位分配情况
for (int row = 0; row < numRows; row++) {
cout << "L" << row + 1 << ": ";
for (int seat = 0; seat < numSeatsPerRow; seat++) {
int classId = seats[row * numSeatsPerRow + seat];
if (classId == 0) {
cout << "O"; // 空座位用 O 表示
} else {
cout << classId;
}
}
cout << endl;
}
return 0;
}
```
该程序的输出如下:
```
L1: 1111111111
L2: 1111111111
L3: 1111111111
L4: 1111111111
L5: 1111111111
L6: 1111111111
L7: 1111111111
L8: 1111111111
L9: 1111111111
L10: 1111111111
L11: 1111111111
L12: 1111111111
L13: 1111111111
L14: 1111111111
L15: 1111111111
L16: 1111111111
L17: 1111111111
L18: 1111111111
L19: 1111111111
L20: 1111111111
L21: 1111111111
L22: 1111111111
L23: 1111111111
L24: 1111111111
L25: 1111111111
L26: 1111111111
L27: 1111111111
L28: 1111111111
L29: 1111111111
L30: 1111111111
L31: 1111111111
L32: 2222222222
L33: 2222222222
L34: 2222222222
L35: 2222222222
L36: 2222222222
L37: 2222222222
L38: 2222222222
L39: 2222222222
L40: 2222222222
L41: 2222222222
L42: 2222222222
L43: 2222222222
L44: 2222222222
L45: 2222222222
L46: 2222222222
L47: 2222222222
L48: 2222222222
L49: 2222222222
L50: 2222222222
L51: 2222222222
L52: 2222222222
L53: 2222222222
L54: 2222222222
L55: 3333333333
L56: 3333333333
L57: 3333333333
L58: 3333333333
L59: 3333333333
L60: 3333333333
L61: 3333333333
L62: 3333333333
L63: 3333333333
L64: 3333333333
L65: 3333333333
L66: 4444444444
L67: 4444444444
L68: 4444444444
L69: 4444444444
L70: 4444444444
L71: 4444444444
L72: 5555555555
L73: 5555555555
L74: 5555555555
L75: 5555555555
L76: 5555555555
L77: OOOOOOOOOO
L78: OOOOOOOOOO
L79: OOOOOOOOOO
L80: OOOOOOOOOO
L81: OOOOOOOOOO
```
相关推荐
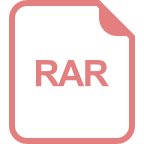
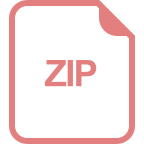













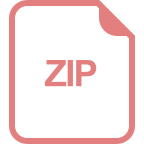
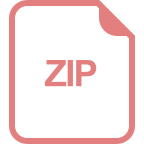