#include<stdio.h> #include<stdlib.h> #include<malloc.h> typedef struct Node {int data; struct node* next; }node; typedef struct qtype {node *front; node *rear; }qtype; int initiatest(qtype *q) { q->front=0; q->rear=0; } int enter(qtype *q,int x) { node *p; p=(node*)malloc(sizeof(node)); p->data=x; p->next=NULL;//新队尾结点 q->rear->next=p;//键入队列中 q->rear=p;//修改队尾指针 } int deletel(qtype *q) {node *p; int x; if(q->front==q->rear) { printf("链队列为空"); return (NULL); } else {p=q->front->next; x=p->data; q->front->next=p->next; if(p->next==NULL) q->rear=q->front; free(p); return (x); } } int print(qtype *q) { int p; p=q->front; while(p!=q->rear) { printf("链队列为:"); return 0; } printf("\n"); } int main() { qtype q=(node*)malloc(sizeof(node)); initiatest(q); enter(q,1); enter(q,2); enter(q,3); enter(q,4); enter(q,5); print(q); deletel(q); print(q); return 0; }
时间: 2024-03-05 15:50:45 浏览: 254
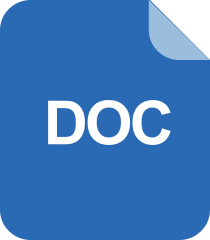
栈和队列的基础上设计模拟停车场管理程序 停车场管理问题 #include<stdio.h> #include<stdlib.h> struct { char status; int num; int time; }a; /*命令的结构*/ typedef struct{ int num; int time; }Element; struct { Element *base; Element *top; in
这段代码中有几处错误:
1. 在结构体 `Node` 中定义了一个指向 `node` 的指针,但是应该是指向 `Node` 的指针,因为 `Node` 是结构体名称,`node` 是指针变量名。所以应该将 `node* next` 修改为 `struct Node* next`。
2. 函数 `initiatest` 没有返回值,但是应该返回一个整型值,表示初始化是否成功。可以将函数类型改为 `int` 并在函数末尾添加 `return 0;`。
3. 函数 `print` 中的循环体没有更新 `p` 的值,导致死循环。可以在循环体中添加 `p = p->next;`。
4. 函数 `print` 中始终只输出了一次字符串 "链队列为:",应该将其移到循环体外。
5. 函数 `deletel` 中的返回值类型应该是 `int` 而不是 `NULL`,因为 `NULL` 是指针类型。
6. 在 `main` 函数中,`q` 的类型应该是 `qtype*` 而不是 `qtype`,因为 `q` 是一个指针变量。所以应该将 `qtype q` 改为 `qtype* q`,并在初始化时使用 `q = (qtype*)malloc(sizeof(qtype));`。
下面是修改后的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <malloc.h>
typedef struct Node {
int data;
struct Node* next;
} node;
typedef struct qtype {
node* front;
node* rear;
} qtype;
int initiatest(qtype* q) {
q->front = NULL;
q->rear = NULL;
return 0;
}
int enter(qtype* q, int x) {
node* p;
p = (node*)malloc(sizeof(node));
p->data = x;
p->next = NULL; // 新队尾结点
if (q->front == NULL) {
q->front = p;
q->rear = p;
} else {
q->rear->next = p; // 键入队列中
q->rear = p; // 修改队尾指针
}
return 0;
}
int deletel(qtype* q) {
node* p;
int x;
if (q->front == NULL) {
printf("链队列为空");
return -1;
} else {
p = q->front->next;
x = p->data;
q->front->next = p->next;
if (p->next == NULL)
q->rear = q->front;
free(p);
return x;
}
}
int print(qtype* q) {
node* p;
p = q->front;
printf("链队列为:");
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return 0;
}
int main() {
qtype* q;
q = (qtype*)malloc(sizeof(qtype));
initiatest(q);
enter(q, 1);
enter(q, 2);
enter(q, 3);
enter(q, 4);
enter(q, 5);
print(q);
deletel(q);
print(q);
return 0;
}
```
阅读全文
相关推荐
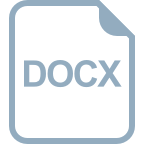
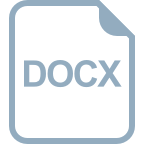
















