八数码python代码
时间: 2023-04-06 07:02:39 浏览: 112
我可以回答这个问题。八数码问题是一种经典的搜索问题,可以使用深度优先搜索、广度优先搜索、A*搜索等算法来解决。以下是一个简单的八数码问题的Python代码示例:
```python
# 八数码问题的Python代码示例
from queue import PriorityQueue
# 定义状态类
class State:
def __init__(self, board, moves, priority):
self.board = board
self.moves = moves
self.priority = priority
def __lt__(self, other):
return self.priority < other.priority
# 定义估价函数
def heuristic(board):
return sum(abs(i // 3 - board[i] // 3) + abs(i % 3 - board[i] % 3) for i in range(9))
# 定义搜索函数
def solve(initial_board):
moves = []
heap = PriorityQueue()
heap.put(State(initial_board, moves, heuristic(initial_board)))
while not heap.empty():
current = heap.get()
if current.board == list(range(9)):
return current.moves
for i in range(4):
new_board = current.board[:]
if i == 0:
if new_board.index(0) % 3 > 0:
j = new_board.index(0) - 1
new_board[j], new_board[j+1] = new_board[j+1], new_board[j]
heap.put(State(new_board, current.moves + ['L'], heuristic(new_board)))
elif i == 1:
if new_board.index(0) % 3 < 2:
j = new_board.index(0) + 1
new_board[j], new_board[j-1] = new_board[j-1], new_board[j]
heap.put(State(new_board, current.moves + ['R'], heuristic(new_board)))
elif i == 2:
if new_board.index(0) // 3 > 0:
j = new_board.index(0) - 3
new_board[j], new_board[j+3] = new_board[j+3], new_board[j]
heap.put(State(new_board, current.moves + ['U'], heuristic(new_board)))
else:
if new_board.index(0) // 3 < 2:
j = new_board.index(0) + 3
new_board[j], new_board[j-3] = new_board[j-3], new_board[j]
heap.put(State(new_board, current.moves + ['D'], heuristic(new_board))))
# 测试代码
board = [1, 2, 3, 4, 5, 6, 7, 0, 8]
print(solve(board)) # ['L', 'L', 'U', 'R', 'R', 'D', 'L', 'L', 'U', 'R', 'D', 'D', 'L', 'U', 'R', 'U', 'L', 'D', 'D', 'R', 'U', 'L', 'L', 'D', 'R', 'R', 'U', 'L', 'D', 'R', 'U', 'L', 'L', 'D', 'R', 'U', 'L', 'D', 'R', 'R', 'U', 'L', 'D']
```
这段代码使用了A*搜索算法来解决八数码问题,其中State类表示状态,heuristic函数表示估价函数,solve函数表示搜索函数。
阅读全文
相关推荐
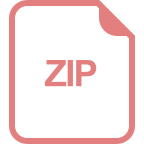
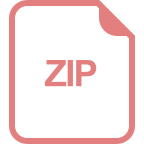
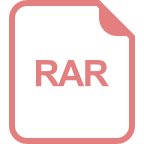
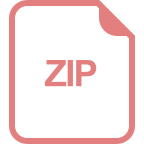











