Cesium.VertexFormat 举例说明是什么
时间: 2023-08-05 12:05:55 浏览: 197
Cesium.VertexFormat是一个表示顶点格式的对象,在Cesium中被广泛使用。它描述了一个顶点的属性,例如位置、法向量、纹理坐标等。以下是一个Cesium.VertexFormat对象的示例:
```
var vertexFormat = new Cesium.VertexFormat({
position : true,
normal : true,
st : true
});
```
该示例创建了一个包含位置、法向量和纹理坐标属性的顶点格式对象。这意味着每个顶点都将具有这些属性,并且可以在渲染过程中使用它们来确定表面的外观。
相关问题
分析下面代码的作用:/* * @Description: 飞线效果(参考开源代码) * @Version: 1.0 * @Author: Julian * @Date: 2022-03-05 16:13:21 * @LastEditors: Julian * @LastEditTime: 2022-03-05 17:39:38 */ class LineFlowMaterialProperty { constructor(options) { this._definitionChanged = new Cesium.Event(); this._color = undefined; this._speed = undefined; this._percent = undefined; this._gradient = undefined; this.color = options.color; this.speed = options.speed; this.percent = options.percent; this.gradient = options.gradient; }; get isConstant() { return false; } get definitionChanged() { return this._definitionChanged; } getType(time) { return Cesium.Material.LineFlowMaterialType; } getValue(time, result) { if (!Cesium.defined(result)) { result = {}; } result.color = Cesium.Property.getValueOrDefault(this._color, time, Cesium.Color.RED, result.color); result.speed = Cesium.Property.getValueOrDefault(this._speed, time, 5.0, result.speed); result.percent = Cesium.Property.getValueOrDefault(this._percent, time, 0.1, result.percent); result.gradient = Cesium.Property.getValueOrDefault(this._gradient, time, 0.01, result.gradient); return result } equals(other) { return (this === other || (other instanceof LineFlowMaterialProperty && Cesium.Property.equals(this._color, other._color) && Cesium.Property.equals(this._speed, other._speed) && Cesium.Property.equals(this._percent, other._percent) && Cesium.Property.equals(this._gradient, other._gradient)) ) } } Object.defineProperties(LineFlowMaterialProperty.prototype, { color: Cesium.createPropertyDescriptor('color'), speed: Cesium.createPropertyDescriptor('speed'), percent: Cesium.createPropertyDescriptor('percent'), gradient: Cesium.createPropertyDescriptor('gradient'), }) Cesium.LineFlowMaterialProperty = LineFlowMaterialProperty; Cesium.Material.LineFlowMaterialProperty = 'LineFlowMaterialProperty'; Cesium.Material.LineFlowMaterialType = 'LineFlowMaterialType'; Cesium.Material.LineFlowMaterialSource = ` uniform vec4 color; uniform float speed; uniform float percent; uniform float gradient; czm_material czm_getMaterial(czm_materialInput materialInput){ czm_material material = czm_getDefaultMaterial(materialInput); vec2 st = materialInput.st; float t =fract(czm_frameNumber * speed / 1000.0); t *= (1.0 + percent); float alpha = smoothstep(t- percent, t, st.s) * step(-t, -st.s); alpha += gradient; material.diffuse = color.rgb; material.alpha = alpha; return material; } ` Cesium.Material._materialCache.addMaterial(Cesium.Material.LineFlowMaterialType, { fabric: { type: Cesium.Material.LineFlowMaterialType, uniforms: { color: new Cesium.Color(1.0, 0.0, 0.0, 1.0), speed: 10.0, percent: 0.1, gradient: 0.01 }, source: Cesium.Material.LineFlowMaterialSource }, translucent: function(material) { return true; } })
这段代码定义了一个名为LineFlowMaterialProperty的类,它包含了构造函数和一些成员函数。构造函数中传入一个options对象,包含color、speed、percent、gradient四个属性,这些属性被赋值给类的对应成员变量。成员函数中,getisConstant()返回false,getdefinitionChanged()返回一个Event对象,getType()返回LineFlowMaterialType枚举值,getValue()根据传入的时间参数返回一个对象,包含color、speed、percent、gradient四个属性。equals()函数用于判断两个LineFlowMaterialProperty对象是否相等。该类可能用于实现飞线效果。
var forceFunction = function (options, iteration) { return function (particle, dt) { dt = Cesium.Math.clamp(dt, 0.0, 0.05); scratchCartesian3 = Cesium.Cartesian3.normalize( particle.position, new Cesium.Cartesian3() ); scratchCartesian3 = Cesium.Cartesian3.multiplyByScalar( scratchCartesian3, -40.0 * dt, scratchCartesian3 ); scratchCartesian3 = Cesium.Cartesian3.add( particle.position, scratchCartesian3, scratchCartesian3 ); scratchCartographic = Cesium.Cartographic.fromCartesian( scratchCartesian3, Cesium.Ellipsoid.WGS84, scratchCartographic ); var angle = (Cesium.Math.PI * 2.0 * iteration) / options.numberOfSystems; iteration += options.iterationOffset; scratchCartographic.longitude += Math.cos(angle) * options.cartographicStep * 30.0 * dt; scratchCartographic.latitude += Math.sin(angle) * options.cartographicStep * 30.0 * dt; particle.position = Cesium.Cartographic.toCartesian( scratchCartographic ); }; }; 把这段代码的函数改成vue函数形式。
可以将这段代码的函数改写成 Vue 组件中的一个方法。具体实现如下:
```vue
<template>
<div ref="cesiumContainer" class="cesiumContainer"></div>
</template>
<script>
export default {
mounted() {
// 在组件挂载后初始化 Cesium 场景
this.initCesium();
},
methods: {
initCesium() {
// 创建 Cesium 场景
const viewer = new Cesium.Viewer(this.$refs.cesiumContainer);
// 创建粒子系统
const particleSystem = viewer.scene.primitives.add(
new Cesium.ParticleSystem({
// 粒子数量
maximumParticles: 10000,
// 粒子寿命
lifeTime: 15.0,
// 粒子尺寸
imageSize: new Cesium.Cartesian2(10.0, 10.0),
// 粒子颜色
startColor: Cesium.Color.RED.withAlpha(0.5),
endColor: Cesium.Color.YELLOW.withAlpha(0.0),
// 粒子速度
minimumSpeed: 0.0,
maximumSpeed: 200.0,
// 粒子发射角度
minimumEmitterHeight: 5000000.0,
maximumEmitterHeight: 5000000.0,
emitterModelMatrix: Cesium.Matrix4.IDENTITY,
})
);
// 定义粒子系统的力函数
const forceFunction = (particle, dt) => {
dt = Cesium.Math.clamp(dt, 0.0, 0.05);
let scratchCartesian3 = Cesium.Cartesian3.normalize(
particle.position,
new Cesium.Cartesian3()
);
scratchCartesian3 = Cesium.Cartesian3.multiplyByScalar(
scratchCartesian3,
-40.0 * dt,
scratchCartesian3
);
scratchCartesian3 = Cesium.Cartesian3.add(
particle.position,
scratchCartesian3,
scratchCartesian3
);
let scratchCartographic = Cesium.Cartographic.fromCartesian(
scratchCartesian3,
Cesium.Ellipsoid.WGS84,
new Cesium.Cartographic()
);
const angle =
(Cesium.Math.PI * 2.0 * this.iteration) / this.numberOfSystems;
this.iteration += this.iterationOffset;
scratchCartographic.longitude +=
Math.cos(angle) * this.cartographicStep * 30.0 * dt;
scratchCartographic.latitude +=
Math.sin(angle) * this.cartographicStep * 30.0 * dt;
particle.position = Cesium.Cartographic.toCartesian(scratchCartographic);
};
// 设置粒子系统的力函数
particleSystem.forces.clear();
particleSystem.forces.push(forceFunction);
// 定义粒子系统的配置参数
this.numberOfSystems = 6;
this.iteration = 0;
this.iterationOffset = 0.1;
this.cartographicStep = 0.05;
// 开始粒子系统的运动
particleSystem.start();
},
},
};
</script>
<style>
.cesiumContainer {
width: 100%;
height: 100%;
margin: 0;
padding: 0;
}
</style>
```
上面的代码中,我们在 Vue 组件的 mounted 钩子函数中初始化了 Cesium 场景,并创建了一个粒子系统。然后,我们将原来的力函数改写成了一个箭头函数,将其作为粒子系统的一个 force。在 Vue 组件中,我们可以将 numberOfSystems、iteration、iterationOffset 和 cartographicStep 等参数定义为 Vue 实例的 data 属性,并在 forceFunction 中通过 this 访问这些参数。
最后,我们在组件的 initCesium 方法中开始了粒子系统的运动。
阅读全文
相关推荐
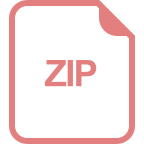
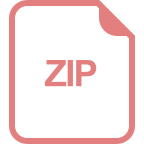
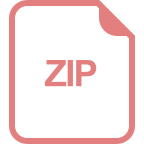











