#include<stdio.h> #include<time.h> #include<stdlib.h> #include<sys/ipc.h> #include<sys/shm.h> #include<string.h> #include <fcntl.h> #include <sys/types.h> #include<unistd.h> struct shared_msg{ char text[BUFSIZ]; int update; }; static int shmid; int cnt=0;//统计计算次数 int flag=0; char str1[100]={0}, str2[100]={0}; struct shared_msg *msg=NULL; int main(){ srand(time(NULL)); //创建共享内存 shmid=shmget((key_t)1234,sizeof(struct shared_msg),IPC_CREAT); if(shmid<0){printf("错误,key 对应的共享内存已存在");exit(-1);} //映射共享内存 msg=(struct shared_msg *)shmat(shmid,0,0); if( msg < (struct shared_msg *)0){printf("映射内存出错!\n");exit(- 2);} msg->update=0; while(1){ while(msg->update==0&&flag==0){ //在屏幕上打印计算后的结果 if(strcmp(msg->text,"end")!=0){ printf("%s\n",msg->text);} if(cnt==10){sprintf(msg->text,"end");flag=1;break;} sprintf(msg->text, "%d+%d", rand()%100, rand()%100); msg->update=1; cnt++; } if(strcmp(msg->text,"over")==0){printf("%s\n",msg->text);break;} } if(shmdt(msg)==-1){//断开共享内存的映射 printf("断开共享内存失败\n"); exit(-3);} if(shmctl(shmid,IPC_RMID,0)==-1){//删除共享内存 printf("删除共享内存失败\n"); exit(-4);} return 0; }
时间: 2024-04-03 14:31:36 浏览: 97
这段代码是一个使用共享内存实现的进程间通信的例子,它包含了以下步骤:
1. 定义了一个名为shared_msg的结构体,它包含了两个成员变量:一个长度为BUFSIZ的字符数组text和一个整型变量update。这个结构体用于定义共享内存中存储的数据类型。
2. 使用shmget函数创建一个共享内存区域(标识符为1234),大小为struct shared_msg的大小,并指定IPC_CREAT选项表示如果内存不存在则创建它。
3. 使用shmat函数将共享内存区域映射到当前进程的地址空间中,并返回一个指向共享内存区域的指针msg。
4. 在一个循环中,不断生成两个随机数,计算它们的和,并将结果存储到共享内存中的text成员变量中。每次更新共享内存后,将update成员变量设置为1,表示共享内存中的数据已经被更新过。
5. 在每次更新共享内存后,等待另一个进程读取共享内存中的数据。为了实现同步,使用msg->update变量进行控制。如果update为0,则表示共享内存中的数据还未被更新,此时当前进程需要等待;如果update为1,则表示共享内存中的数据已经被更新,此时可以读取共享内存中的数据。
6. 当计算次数达到10次时,向共享内存中写入"end"字符串,表示计算已经结束。当另一个进程读取到"end"字符串时,退出循环。
7. 在程序结束前,使用shmdt函数断开共享内存的映射,并使用shmctl函数删除共享内存区域。
相关问题
#include <pthread.h> #include <stdio.h> #include <stdlib.h> #include <string.h> #include "MQTTClient.h" #include <stdint.h> #include <signal.h> /* sigaction */ #include <errno.h> #include "sqlite3.h" #include "pan_reg.h" #include "spi_func.h" #include "auxiliary.h" #if !defined(WIN32) #include <unistd.h> #else #include <windows.h> #endif #include <netdb.h> #include <sys/types.h> #include <sys/socket.h> #include <netinet/in.h> #include <math.h> /* modf */ #include "cJSON.h" #include <fcntl.h> #include "uart.h" #include <sys/types.h> #include <sys/ipc.h> #include <sys/msg.h>
这是一段 C 语言的代码,包含了一些头文件的引用,如 pthread.h、stdio.h、stdlib.h、string.h、MQTTClient.h、stdint.h、signal.h、errno.h、sqlite3.h、netdb.h、sys/types.h、sys/socket.h、netinet/in.h、math.h、cJSON.h、fcntl.h 和 uart.h。这段代码中可能包含了一些线程库、网络库、消息队列、JSON 解析等相关的函数。具体实现需要查看代码的具体逻辑。
解释代码#include <sys/types.h> #include <sys/ipc.h> #include <sys/msg.h> #include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <string.h> #define MSGKEY 75 struct msgform { long mt
这段代码定义了一个消息队列,在 Linux 系统中,消息队列是一种进程间通信的方式。代码中包含了以下头文件:
- sys/types.h:定义了系统调用所使用的数据类型,例如 pid_t、size_t 等。
- sys/ipc.h:定义了用于进程间通信的 IPC(Inter-Process Communication)相关函数和数据结构,例如消息队列、信号量、共享内存等。
- sys/msg.h:定义了消息队列相关的函数和数据结构,例如 msgget、msgsnd、msgrcv 等。
- stdio.h:定义了输入输出函数,例如 printf、scanf 等。
- stdlib.h:定义了内存管理函数,例如 malloc、free 等。
- unistd.h:定义了一些 UNIX 标准的函数和符号常量,例如 sleep、fork、getpid 等。
- string.h:定义了一些字符串处理函数,例如 memcpy、memset 等。
在代码中,使用了宏定义 MSGKEY 定义了消息队列的键值。结构体 msgform 定义了消息的格式,包含了一个长整型变量 mt 和一个字符数组 mtext。后面的代码中使用了 msgget 函数获取消息队列的标识符,使用了 msgsnd 函数发送消息,使用了 msgrcv 函数接收消息。
阅读全文
相关推荐
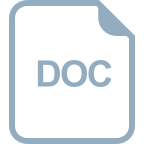
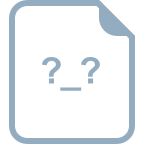













