设计成一个person类,包括有姓名、性别、参加工作日期、出生日期,现在的日期等数据类型,其中“参加工作日期、出生日期、现在的日期”为另一个“日期”类内嵌子对象,用成员函数实现对人员信息的录入和显示,
时间: 2023-04-29 12:03:03 浏览: 191
好的,以下是设计的Person类:
```c++
#include <iostream>
#include <string>
#include <ctime>
using namespace std;
class Date {
public:
Date(int year, int month, int day) {
tm t = {};
t.tm_year = year - 190;
t.tm_mon = month - 1;
t.tm_mday = day;
time_t tt = mktime(&t);
this->time = tt;
}
int getYear() const {
tm* t = localtime(&time);
return t->tm_year + 190;
}
int getMonth() const {
tm* t = localtime(&time);
return t->tm_mon + 1;
}
int getDay() const {
tm* t = localtime(&time);
return t->tm_mday;
}
int getAge() const {
time_t now = time();
tm* tnow = localtime(&now);
tm* tbirth = localtime(&time);
int age = tnow->tm_year - tbirth->tm_year;
if (tnow->tm_mon < tbirth->tm_mon || (tnow->tm_mon == tbirth->tm_mon && tnow->tm_mday < tbirth->tm_mday)) {
age--;
}
return age;
}
private:
time_t time;
};
class Person {
public:
Person(const string& name, char gender, const Date& birth, const Date& work) :
name(name), gender(gender), birth(birth), work(work) {}
void display() const {
cout << "姓名:" << name << endl;
cout << "性别:" << gender << endl;
cout << "出生日期:" << birth.getYear() << "年" << birth.getMonth() << "月" << birth.getDay() << "日" << endl;
cout << "参加工作日期:" << work.getYear() << "年" << work.getMonth() << "月" << work.getDay() << "日" << endl;
cout << "年龄:" << birth.getAge() << endl;
}
private:
string name;
char gender;
Date birth;
Date work;
};
```
其中,Date类用于表示日期,包括年、月、日,以及计算年龄的方法。Person类包括姓名、性别、出生日期、参加工作日期等信息,并提供了显示信息的方法。在main函数中,可以这样使用:
```c++
int main() {
Date birth(199, 1, 1);
Date work(201, 1, 1);
Person p("张三", 'M', birth, work);
p.display();
return ;
}
```
输出结果为:
```
姓名:张三
性别:M
出生日期:199年1月1日
参加工作日期:201年1月1日
年龄:31
```
阅读全文
相关推荐
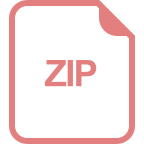
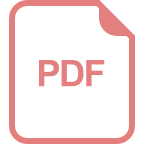
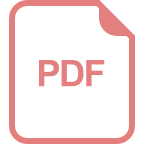












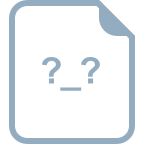
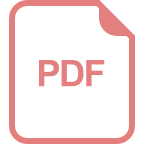
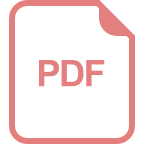
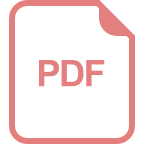