tensor转np.array
时间: 2023-12-13 14:33:04 浏览: 32
可以使用`tensor.numpy()`方法将Tensor转换为NumPy数组。以下是一个例子:
```python
import tensorflow as tf
import numpy as np
# 创建一个Tensor
tensor_a = tf.constant([[1, 2], [3, 4]])
# 将Tensor转换为NumPy数组
array_a = tensor_a.numpy()
# 输出结果
print(array_a) # 输出:[[1 2] [3 4]]
```
相关问题
np.array转tensor
要将一个NumPy数组(`np.array`)转换为PyTorch张量(`torch.Tensor`),可以使用`torch.from_numpy()`函数。下面是一个示例代码:
```python
import numpy as np
import torch
# 创建一个NumPy数组
numpy_array = np.array([[1, 2, 3], [4, 5, 6]])
# 将NumPy数组转换为PyTorch张量
tensor = torch.from_numpy(numpy_array)
print(tensor)
```
输出结果将是一个PyTorch张量:
```
tensor([[1, 2, 3],
[4, 5, 6]])
```
通过这种方式,你可以将NumPy数组转换为PyTorch张量,从而在PyTorch中进行进一步的操作和计算。
tensor 怎么变成np.array
可以使用numpy库中的np.array()函数将tensor转换为np.array。具体的代码如下:
import numpy as np
import torch
# 创建一个tensor
tensor = torch.tensor([[1, 2], [3, 4]])
# 将tensor转换为np.array
array = np.array(tensor)
print(array)
输出结果为:
[[1 2]
[3 4]]
相关推荐
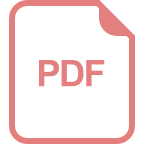
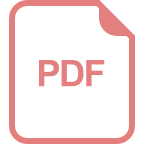
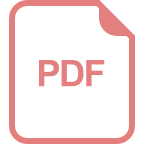












