def get_max_pos_step(self, max_pos, max_score, next_c,next_r,round, chess_color): if self.is_pos_inside(next_r,next_c)and not self.matrix[next_r][next_c]: score = self.evaluate(next_r,next_c,round,chess_color) if score >max_score: max_pos,max_score = (next_r,next_c),score return max_pos,max_score def is_pos_inside(self,row,col): return 0 <= row < self.n and 0 <= col < self.n
时间: 2024-04-12 21:33:31 浏览: 113
这段代码看起来是一个类的两个方法。第一个方法是`get_max_pos_step`,它接受一些参数,并返回最大分数和对应的位置。在这个方法中,它首先检查给定的位置是否在棋盘内并且没有棋子。如果满足条件,它会调用另一个方法`evaluate`来计算给定位置的分数。如果计算的分数大于当前最大分数,它会更新最大分数和对应的位置。最后,它返回最大分数和对应的位置。
第二个方法是`is_pos_inside`,它接受行和列作为参数,并检查给定的位置是否在棋盘内。如果行和列的值都在合法范围内(0到n-1),则返回True,否则返回False。
这些方法可能是一个棋盘游戏中的一部分,用于找到下一步的最佳位置。
相关问题
def get_logic_pos(self,x,y): return (y-self.margin + self.cell_width//2)//self.cell_width, (x-self.margin + self.cell_width//2)//self.cell_width def judge_line(self,row,col,direct,chess_color): c = 1 for i in range(1,6): next_row, next_col = row + direct[0][0] * i, col + direct[0][1] * i if self.matrix[next_row][next_col] == chess_color: c +=1 else: break for i in range(1, 6): next_row, next_col = row + direct[1][0] * i, col + direct[1][1] * i if self.matrix[next_row][next_col] == chess_color: c +=1 else: break return c def judge(self,row,col,chess_color): for direct in [[(-1,0),(1,0)],[(0,-1),(0,1)],[(-1,1),(1,-1)],[(-1,-1),(1,1)]]: if self.judge_line(row,col,direct,chess_color) ==6: return chess_color if len(self.history) == self.n * self.n: return -1 return 0 def deal_with_judge(self, judge_result): if not judge_result: return if judge_result == 1: txt = 'Black Win' elif judge_result == 2: txt = 'White Win' elif judge_result == -1: txt = 'Draw Chess' self.gameboard.draw_box(txt) self.full_matrix(self.n) def put_chess(self,x,y): l = len(self.history) chess_color = (l+1) % 4 // 2+1 if chess_color == self.auto_color: row, col = self.AI.generate_next(self.history, 1 - len(self.history) % 2, chess_color) else: row,col = self.get_logic_pos(x,y) if self.matrix[row][col] == 0: self.history.append((row, col, chess_color)) self.matrix[row][col] = chess_color self.gameboard.drawchess(row, col, chess_color) self.gameboard.draw_now_chess(chess_color) self.deal_with_judge(self.judge(row,col,chess_color)) def full_matrix(self,n): for i in range(self.n): for j in range(self.n): self.matrix[i][j] = 1
这段代码是GameBoard类的一些额外方法。让我来逐个解释它们的功能:
1. get_logic_pos方法接受鼠标点击的屏幕坐标x和y,计算出逻辑位置(行、列)并返回。
2. judge_line方法用于判断指定位置的棋子在指定方向上是否连成一条线。它接受行、列、方向和棋子颜色作为参数,并返回连线的数量。
3. judge方法用于判断给定位置的棋子是否达成胜利条件。它接受行、列和棋子颜色作为参数,并根据棋子在水平、垂直和斜向上的连线数量判断胜负情况。
4. deal_with_judge方法根据judge方法返回的结果进行处理。如果有胜负或平局,它将在游戏板上显示相应的文本框。
5. put_chess方法用于在指定位置放置棋子。它接受鼠标点击的屏幕坐标x和y,并根据当前该轮是玩家还是AI下棋来决定棋子的颜色及位置。如果位置合法,它会更新历史记录、矩阵和游戏板的显示,并调用deal_with_judge方法判断胜负情况。
6. full_matrix方法用于将整个矩阵填充为1,表示所有位置都已经下满棋子。
这些方法似乎是一个与GameBoard类相关的游戏逻辑的一部分。
def evaluate(self,row,col,round,chess_color): score = 0 for d in [((0,-1),(0,1)),((-1,-1),(1,1)),((-1,0),(1,0)),((-1,1), (1,-1))]: c1, c2, c3= self.count_line(d,row,col,chess_color) print(row, col, chess_color, c1,c2,c3) tp11, tp12 = self.get_chess_type(c1, c2, c3) c1, c2, c3 = self.count_line(d, row, col, 3-chess_color) print(row, col, 3-chess_color, c1,c2,c3) tp21, tp22 = self.get_chess_type(c1, c2, c3) print("tp11, tp12, tp21, tp22", tp11, tp12, tp21, tp22) score += self.dic_score[tp11][tp12][2-round] + self.dic_score[tp21][tp22][0] return score def get_chess_type(selfself,c1,c2,c3): if c1 >= 6: return 6,0 else: if c2 >1: if c3 < 6: return c2,0 elif c3 ==6: return c2,1 else: return c2,2 else: return 1 , 0
这段代码是一个类的两个方法。第一个方法是`evaluate`,它接受一些参数,并返回一个分数。在这个方法中,它使用一个循环来遍历四个方向的线(水平、垂直和两个对角线)。对于每个方向,它调用`count_line`方法来计算给定位置在该方向上的连续棋子数。然后,它调用`get_chess_type`方法来确定给定连续棋子数的类型。然后,它根据棋子类型查找一个预先定义的字典`dic_score`来获取分数,并将该分数添加到总分数中。最后,它返回总分数。
第二个方法是`get_chess_type`,它接受三个连续棋子数作为参数,并根据这些数值确定棋子类型。如果第一个数大于等于6,它返回6和0作为类型。否则,它检查第二个数的值是否大于1。如果是,它进一步检查第三个数的值。根据不同的情况,它返回不同的类型值。如果第二个数小于等于1,则返回1和0作为类型。
这些方法可能是用于评估给定位置的棋子类型并计算相应的分数。这可能是用于决策下一步最佳位置的一部分。
阅读全文
相关推荐
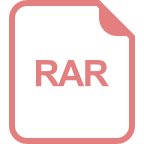
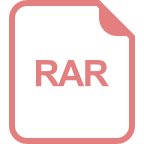
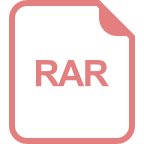



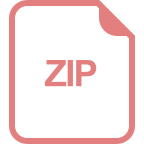
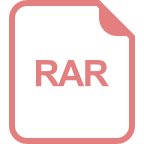
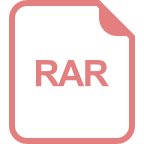
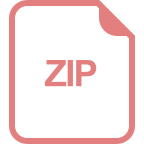






