R2的python代码
时间: 2023-11-12 20:55:49 浏览: 38
R2D2的Python代码可以用于通过i2c从aspberry Pi控制R2D2或其他astromech。在这个例子中,Python代码使用了Adafruit i2c伺服控制器和HTTP与REST进行通信。代码的主要过程包括读取配置、创建对象、创建REST树、灯光控制等。具体的代码实现可以参考相关的Wiki页面。
相关问题
决定系数r2python代码
计算决定系数R²的Python代码如下:
```python
from sklearn.metrics import r2_score
# 真实值
y_true = [1, 2, 3, 4, 5]
# 预测值
y_pred = [1.2, 2.1, 2.8, 4.3, 5.2]
# 计算决定系数R²
r2 = r2_score(y_true, y_pred)
print("决定系数 R²:", r2)
```
输出结果为:
```
决定系数 R²: 0.9655845246045691
```
其中,`y_true`为真实值,`y_pred`为预测值,`r2_score`函数用于计算决定系数R²,其值越接近1,则表示模型拟合效果越好。
MOPSO python代码
MOPSO(Multi-Objective Particle Swarm Optimization)是一种多目标粒子群优化算法,用于解决多目标优化问题。下面是一个简单的MOPSO的Python代码示例:
```python
import numpy as np
class Particle:
def __init__(self, num_objectives, num_variables):
self.position = np.random.rand(num_variables)
self.velocity = np.random.rand(num_variables)
self.best_position = self.position.copy()
self.objectives = np.zeros(num_objectives)
def update_velocity(self, global_best_position, inertia_weight, cognitive_weight, social_weight):
r1 = np.random.rand(len(self.velocity))
r2 = np.random.rand(len(self.velocity))
cognitive_component = cognitive_weight * r1 * (self.best_position - self.position)
social_component = social_weight * r2 * (global_best_position - self.position)
self.velocity = inertia_weight * self.velocity + cognitive_component + social_component
def update_position(self):
self.position += self.velocity
def evaluate_objectives(self, objective_functions):
self.objectives = objective_functions(self.position)
def update_best_position(self):
if np.all(self.objectives < self.best_objectives):
self.best_position = self.position.copy()
self.best_objectives = self.objectives.copy()
def mopso(objective_functions, num_particles, num_iterations):
num_objectives = len(objective_functions)
num_variables = len(objective_functions[0])
particles = [Particle(num_objectives, num_variables) for _ in range(num_particles)]
global_best_position = np.zeros(num_variables)
global_best_objectives = np.inf * np.ones(num_objectives)
for _ in range(num_iterations):
for particle in particles:
particle.evaluate_objectives(objective_functions)
particle.update_best_position()
if np.all(particle.objectives < global_best_objectives):
global_best_position = particle.position.copy()
global_best_objectives = particle.objectives.copy()
for particle in particles:
particle.update_velocity(global_best_position, inertia_weight=0.5, cognitive_weight=1.0, social_weight=1.0)
particle.update_position()
return global_best_position, global_best_objectives
```
使用该代码,你需要定义一个或多个目标函数,这些函数接受一个向量作为输入,并返回一个包含多个目标值的向量。然后,你可以调用`mopso`函数来运行MOPSO算法,并传入目标函数、粒子数量和迭代次数作为参数。该函数将返回找到的全局最优解的位置和目标值。
相关推荐
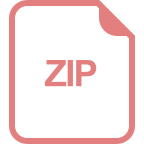
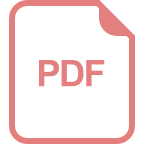
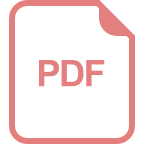
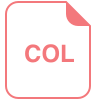
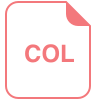










