TensorFlow 中的数据增强技术详解
发布时间: 2024-05-03 01:07:16 阅读量: 104 订阅数: 36 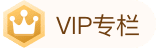
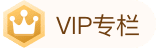
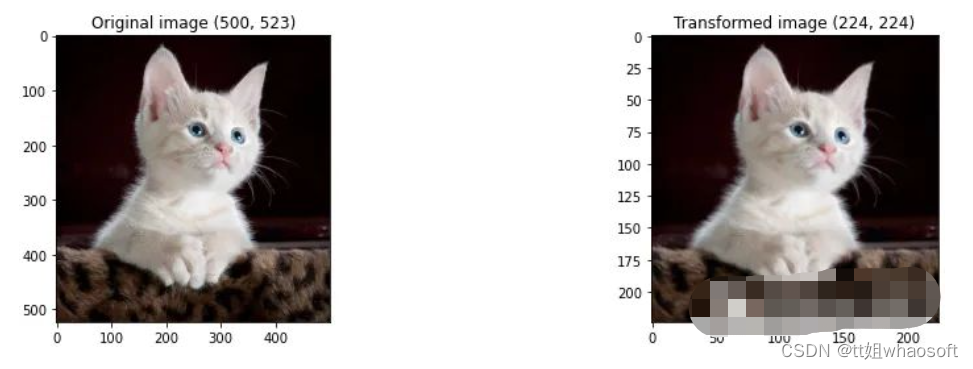
# 1. TensorFlow数据增强概述
TensorFlow数据增强是一种通过对原始数据进行变换,从而生成更多样化和丰富的数据集的技术。它可以有效地提高机器学习模型的泛化能力,防止过拟合,并提升模型在真实世界中的表现。TensorFlow提供了丰富的API,支持对图像和序列数据进行各种增强操作,包括翻转、旋转、裁剪、缩放、时间平移和长度改变等。
# 2. TensorFlow数据增强基础
### 2.1 图像增强基础
图像增强是数据增强中最常用的技术之一,它可以通过对原始图像进行各种变换来生成新的图像,从而增加训练数据集的多样性。TensorFlow提供了丰富的图像增强操作,包括图像翻转、旋转、裁剪、缩放等。
#### 2.1.1 图像翻转
图像翻转是一种简单的增强技术,它可以将图像沿水平或垂直轴翻转。TensorFlow提供了`tf.image.flip_left_right()`和`tf.image.flip_up_down()`函数来实现图像翻转。
```python
import tensorflow as tf
# 读取原始图像
image = tf.io.read_file('image.jpg')
image = tf.image.decode_jpeg(image)
# 水平翻转图像
flipped_image = tf.image.flip_left_right(image)
# 垂直翻转图像
flipped_image = tf.image.flip_up_down(image)
```
#### 2.1.2 图像旋转
图像旋转是一种更复杂的增强技术,它可以将图像绕任意角度旋转。TensorFlow提供了`tf.image.rot90()`函数来实现图像旋转,它可以将图像旋转90度。
```python
# 旋转图像90度
rotated_image = tf.image.rot90(image, k=1)
```
### 2.2 序列增强基础
序列增强与图像增强类似,但它适用于序列数据,如时间序列或文本序列。TensorFlow提供了丰富的序列增强操作,包括序列时间平移、长度改变等。
#### 2.2.1 序列时间平移
序列时间平移是一种增强技术,它可以将序列中的元素向左或向右移动一定的时间步长。TensorFlow提供了`tf.data.Dataset.window()`函数来实现序列时间平移。
```python
# 创建一个时间序列数据集
dataset = tf.data.Dataset.from_tensor_slices([1, 2, 3, 4, 5])
# 将序列中的元素向左移动1个时间步长
windowed_dataset = dataset.window(size=2, shift=1, drop_remainder=True)
```
#### 2.2.2 序列长度改变
序列长度改变是一种增强技术,它可以改变序列的长度。TensorFlow提供了`tf.data.Dataset.padded_batch()`和`tf.data.Dataset.unbatch()`函数来实现序列长度改变。
```python
# 将序列中的元素填充到指定长度
padded_dataset = dataset.padded_batch(batch_size=32, padded_shapes=(None,))
# 将序列中的元素解批处理
unbatched_dataset = padded_dataset.unbatch()
```
# 3.1 图像翻转与旋转增强
#### 3.1.1 tf.image.flip_left_right()
`tf.image.flip_left_right()` 函数用于沿图像的水平轴进行翻转。它接受一个图像张量作为输入,并返回一个翻转后的图像张量。
**参数:**
* `image`: 输入图像张量,形状为 `[height, width, channels]`。
* `seed`: 用于随机数生成器的种子。默认值为 `None`,表示使用全局种子。
**代码块:**
```python
import tensorflow as tf
image = tf.random.uniform(shape=(256, 256, 3))
flipped_image = tf.image.flip_left_right(image)
```
**逻辑分析:**
该代码块生成一个随机图像,然后使用 `tf.image.flip_left_right()` 函数沿水平轴将其翻转。
#### 3.1.2 tf.image.random_flip_left_right()
`tf.image.random_flip_left_right()` 函数用于随机沿图像的水平轴进行翻转。它接受一个图像张量作为输入,并返回一个随机翻转后的图像张量。
**参数:**
* `image`: 输入图像张量,形状为 `[height, width, channels]`。
* `seed`: 用于随机数生成器的种子。默认值为 `None`,表示使用全局种子。
**代码块:**
```python
import tensorflow as tf
image = tf.random.uniform(shape=(256, 256, 3))
random_flipped_image = tf.image.random_flip_left_right(image)
```
**逻辑分析:**
该代码块生成一个随机图像,然后使用 `tf.image.random_flip_left_right()` 函数随机沿水平轴将其翻转。
#### 3.1.3 tf.image.random_flip_up_down()
`tf.image.random_flip_up_down()` 函数用于随机沿图像的垂直轴进行翻转。它接受一个图像张量作为输入,并返回一个随机翻转后的图像张量。
**参数:**
* `image`: 输入图像张量,形状为 `[height, width, channels]`。
* `seed`: 用于随机数生成器的种子。默认值为 `None`,表示使用全局种子。
**代码块:**
```python
import tensorflow as tf
image = tf.random.uniform(shape=(256, 256, 3))
random_flipped_image = tf.image.random_flip_up_down(image)
```
**逻辑分析:**
该代码块生成一个随机图像,然后使用 `tf.image.random_flip_up_down()` 函数随机沿垂直轴将其翻转。
#### 3.1.4 tf.image.random_rotation()
`tf.image.random_rotation()` 函数用于随机旋转图像。它接受一个图像张量作为输入,并返回一个随机旋转后的图像张量。
**参数:**
* `image`: 输入图像张量,形状为 `[height, width, channels]`。
* `max_angle`: 旋转的最大角度,以弧度为单位。
* `seed`: 用于随机数生成器的种子。默认值为 `None`,表示使用全局种子。
**代码块:**
```python
import tensorflow as tf
image = tf.random.uniform(shape=(256, 256, 3))
random_rotated_image = tf.image.random_rotation(image, max_angle=0.5)
```
**逻辑分析:**
该代码块生成一个随机图像,然后使用 `tf.image.random_rotation()` 函数随机将其旋转最大 0.5 弧度。
# 4. TensorFlow序列增强实践
### 4.1 序列时间平移增强
#### 4.1.1 tf.data.Dataset.window()
`tf.data.Dataset.window()` 函数用于在序列中创建滑动窗口,允许对序列中的元素进行时间平移。其语法如下:
```python
window(size, shift=None, drop_remainder=False) -> Dataset
```
其中:
* `size`: 窗口的大小。
* `shift`: 窗口在序列中移动的步长。如果未指定,则默认为 `size`。
* `drop_remainder`: 是否丢弃窗口大小之外的剩余元素。如果为 `True`,则丢弃剩余元素;如果为 `False`,则保留剩余元素。
**代码示例:**
```python
import tensorflow as tf
# 创建一个序列数据集
dataset = tf.data.Dataset.range(10)
# 使用窗口大小为 3 的滑动窗口
window_dataset = dataset.window(3)
# 迭代窗口数据集
for window in window_dataset:
print(window)
```
**输出:**
```
tf.Tensor([0 1 2], shape=(3,), dtype=int64)
tf.Tensor([1 2 3], shape=(3,), dtype=int64)
tf.Tensor([2 3 4], shape=(3,), dtype=int64)
tf.Tensor([3 4 5], shape=(3,), dtype=int64)
tf.Tensor([4 5 6], shape=(3,), dtype=int64)
tf.Tensor([5 6 7], shape=(3,), dtype=int64)
tf.Tensor([6 7 8], shape=(3,), dtype=int64)
tf.Tensor([7 8 9], shape=(3,), dtype=int64)
```
**逻辑分析:**
`window()` 函数创建了一个滑动窗口,窗口大小为 3。窗口在序列中移动一步,每次移动都会生成一个新的窗口。窗口中包含的元素是序列中连续的 3 个元素。
#### 4.1.2 tf.data.Dataset.batch()
`tf.data.Dataset.batch()` 函数用于将序列中的元素打包成批次。其语法如下:
```python
batch(batch_size) -> Dataset
```
其中:
* `batch_size`: 每个批次的大小。
**代码示例:**
```python
# 使用窗口大小为 3 的滑动窗口
window_dataset = dataset.window(3)
# 将窗口打包成批次,批次大小为 2
batched_dataset = window_dataset.batch(2)
# 迭代批次数据集
for batch in batched_dataset:
print(batch)
```
**输出:**
```
tf.Tensor([[0 1 2]
[1 2 3]], shape=(2, 3), dtype=int64)
tf.Tensor([[2 3 4]
[3 4 5]], shape=(2, 3), dtype=int64)
tf.Tensor([[4 5 6]
[5 6 7]], shape=(2, 3), dtype=int64)
tf.Tensor([[6 7 8]
[7 8 9]], shape=(2, 3), dtype=int64)
```
**逻辑分析:**
`batch()` 函数将窗口打包成批次,每个批次包含 2 个窗口。批次中的窗口是连续的,并且批次中的元素是序列中连续的元素。
### 4.2 序列长度改变增强
#### 4.2.1 tf.data.Dataset.padded_batch()
`tf.data.Dataset.padded_batch()` 函数用于将序列中的元素打包成批次,并对序列进行填充。其语法如下:
```python
padded_batch(batch_size, padded_shapes, padding_values=None, drop_remainder=False) -> Dataset
```
其中:
* `batch_size`: 每个批次的大小。
* `padded_shapes`: 一个形状列表,指定每个批次中每个元素的填充形状。
* `padding_values`: 一个值列表,指定填充值。如果未指定,则使用默认值。
* `drop_remainder`: 是否丢弃窗口大小之外的剩余元素。如果为 `True`,则丢弃剩余元素;如果为 `False`,则保留剩余元素。
**代码示例:**
```python
# 创建一个序列数据集
dataset = tf.data.Dataset.range(10)
# 将序列打包成批次,批次大小为 2,填充形状为 [3]
padded_dataset = dataset.padded_batch(2, [3])
# 迭代批次数据集
for batch in padded_dataset:
print(batch)
```
**输出:**
```
tf.Tensor([[0 1 2]
[3 4 5]], shape=(2, 3), dtype=int64)
tf.Tensor([[6 7 8]
[9 0 0]], shape=(2, 3), dtype=int64)
```
**逻辑分析:**
`padded_batch()` 函数将序列打包成批次,每个批次包含 2 个序列。序列的长度被填充到 3,填充值默认为 0。批次中的序列是连续的,并且批次中的元素是序列中连续的元素。
#### 4.2.2 tf.data.Dataset.unbatch()
`tf.data.Dataset.unbatch()` 函数用于将批次中的元素解压缩成序列。其语法如下:
```python
unbatch() -> Dataset
```
**代码示例:**
```python
# 将批次中的元素解压缩成序列
unbatched_dataset = padded_dataset.unbatch()
# 迭代序列数据集
for element in unbatched_dataset:
print(element)
```
**输出:**
```
tf.Tensor(0, shape=(), dtype=int64)
tf.Tensor(1, shape=(), dtype=int64)
tf.Tensor(2, shape=(), dtype=int64)
tf.Tensor(3, shape=(), dtype=int64)
tf.Tensor(4, shape=(), dtype=int64)
tf.Tensor(5, shape=(), dtype=int64)
tf.Tensor(6, shape=(), dtype=int64)
tf.Tensor(7, shape=(), dtype=int64)
tf.Tensor(8, shape=(), dtype=int64)
tf.Tensor(9, shape=(), dtype=int64)
```
**逻辑分析:**
`unbatch()` 函数将批次中的元素解压缩成序列。序列中的元素是批次中元素的连续序列。
# 5.1 图像混合增强
图像混合增强是一种将两张或多张图像融合在一起以创建新图像的技术。TensorFlow 提供了 `tf.image.blend()` 和 `tf.image.blend_with_mask()` 函数来实现图像混合增强。
### 5.1.1 tf.image.blend()
`tf.image.blend()` 函数将两张图像融合在一起,创建一张新的图像。该函数接受以下参数:
- `image1`: 第一幅图像。
- `image2`: 第二幅图像。
- `alpha`: 一个浮点数,表示 `image2` 在混合图像中所占的权重。
```python
import tensorflow as tf
# 加载两张图像
image1 = tf.io.read_file('image1.jpg')
image1 = tf.image.decode_jpeg(image1, channels=3)
image2 = tf.io.read_file('image2.jpg')
image2 = tf.image.decode_jpeg(image2, channels=3)
# 混合图像
blended_image = tf.image.blend(image1, image2, alpha=0.5)
```
### 5.1.2 tf.image.blend_with_mask()
`tf.image.blend_with_mask()` 函数将两张图像融合在一起,使用掩码来控制混合的区域。该函数接受以下参数:
- `image1`: 第一幅图像。
- `image2`: 第二幅图像。
- `mask`: 一个二值掩码,表示 `image2` 应混合的区域。
```python
import tensorflow as tf
# 加载两张图像和掩码
image1 = tf.io.read_file('image1.jpg')
image1 = tf.image.decode_jpeg(image1, channels=3)
image2 = tf.io.read_file('image2.jpg')
image2 = tf.image.decode_jpeg(image2, channels=3)
mask = tf.io.read_file('mask.png')
mask = tf.image.decode_png(mask, channels=1)
# 混合图像
blended_image = tf.image.blend_with_mask(image1, image2, mask)
```
0
0
相关推荐
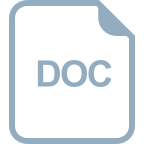
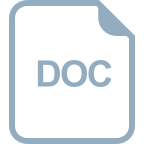
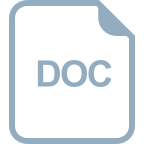
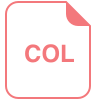
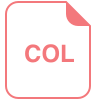
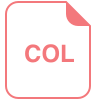
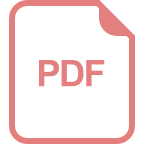
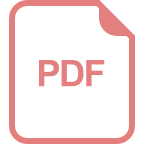
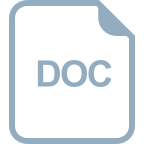