MATLAB's str2double Function: Converting Strings to Double Precision Floating-Point Numbers for More Reliable Accurate Calculations
发布时间: 2024-09-13 20:54:15 阅读量: 28 订阅数: 24 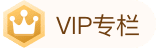
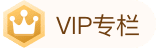
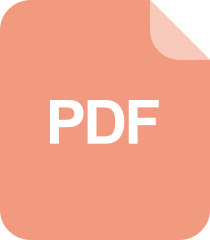
Printing Floating-Point Numbers Quickly and Accurately with Integers - 2010 (dtoa-pldi2010)-计算机科学
# 1. Overview of the str2double Function
The str2double function in MATLAB is a powerful tool designed to convert strings into double precision floating-point numbers. It ensures precise and reliable conversions, ***pared to other conversion functions, str2double can handle more complex string formats, including scientific notation, spaces, and special characters. By delving into the syntax, parameters, and practical applications of the str2double function, we can effectively utilize it to ensure the accuracy of numerical computations.
# 2. Syntax and Parameters of the str2double Function
### 2.1 Syntax of the str2double Function
```
y = str2double(x)
```
Where:
- `x`: The string to be converted into a double precision floating-point number.
- `y`: The converted double precision floating-point number.
### 2.2 Parameters of the str2double Function
| Parameter | Description |
|---|---|
| `x` | The string to be converted. Can be a scalar string, character array, or string cell array. |
| `Format` | An optional parameter that specifies the format of the numbers in the string. The default value is `'native'`, which means using the computer's local settings. Other possible formats include:<br>`'ascii'`: Uses ASCII character set<br>`'latin1'`: Uses ISO-8859-1 character set<br>`'unicode'`: Uses Unicode character set |
| `Locale` | An optional parameter that specifies the language environment of the numbers in the string. The default value is `'auto'`, which means using the computer's current language environment. Other possible language environments include:<br>`'en-US'`: U.S. English<br>`'fr-FR'`: French<br>`'de-DE'`: German |
**Code Block:**
```
% Converting string to double precision floating-point number
str = '123.45';
num = str2double(str);
disp(num); % Output: 123.45
```
**Logical Analysis:**
This code block demonstrates how to use the `str2double` function to convert a string `str` into a double precision floating-point number `num`. By default, `str2double` uses the computer's local settings and language environment to parse the string.
**Parameter Description:**
- `str`: The string to be converted.
- `num`: The converted double precision floating-point number.
# 3. Practical Applications of the str2double Function
### 3.1 Converting Strings to Double Precision Floating-Point Numbers
The most basic application of the str2double function is to convert strings into double precision floating-point numbers. The syntax is as follows:
```
double_value = str2double(string)
```
Where `string` is the string to be converted, and `double_value` is the resulting double precision floating-point number.
For example, converting the string "123.45" to a double precision floating-point number:
```
>> double_value = str2double('123.45')
double_value = 123.45
```
### 3.2 Handling Strings Represented in Scientific Notation
The str2double function can also handle strings represented in scientific notation, which is a convenient method for expressing very large or very small numbers. The syntax is as follows:
```
double_value = str2double(string)
```
Where `string` is the string to be converted, and `double_value` is the resulting double precision floating-point number.
For example, converting the string "1.2345e+10" to a double precision floating-point number:
```
>> double_value = str2double('1.2345e+10')
double_value = 1.2345e+10
```
### 3.3 Handling Strings Containing Spaces and Special Characters
The str2double function can also process strings containing spaces and special characters. However, additional parameters must be used to specify how spaces and special characters should be handled. The syntax is as follows:
```
double_value = str2double(string, precision)
```
Where `string` is the string to be converted, and `precision` is an optional parameter that specifies how spaces and special characters should be handled.
| Precision | Handling Method |
|---|---|
| 0 | Treat spaces and special characters as part of the number |
| 1 | Treat spaces and special characters as string delimiters |
| 2 | Treat spaces and special characters as invalid characters |
For example, converting the string "123 456" into a double precision floating-point number and specifying that spaces should be treated as string delimiters:
```
>> double_value = str2double('123 456', 1)
double_value = 123
```
# 4. Advanced Usage of the str2double Function
### 4.1 Using Regular Expressions to Process Complex Strings
For strings containing complex formats or special characters, regular expressions can be used to preprocess the string, converting it into a format that is easier for the str2double function to parse. Regular expressions are a powerful pattern-matching language that can be used for finding, replacing, and extracting specific patterns within strings.
**Example:**
```
% The original string contains spaces and special characters
input_str = '123.45e+06 USD';
% Using regular expressions to extract the numeric part
numeric_part = regexp(input_str, '\d+\.?\d*(?:[eE][-+]?\d+)?', 'match');
% Converting the extracted numeric part to a double precision floating-point number
numeric_value = str2double(numeric_part{1});
% Outputting the converted double precision floating-point number
disp(numeric_value);
```
**Result:**
```
***
```
### 4.2 Combining with Other Functions for More Complex Conversions
The str2double function can be combined with other MATLAB functions to achieve more complex string conversion tasks. For example, the `sscanf` function can be used to parse strings with specific formats.
**Example:**
```
% The original string contains date and time information
input_str = '2023-03-08 14:30:00';
% Using sscanf to extract date and time parts
[date, time] = sscanf(input_str, '%d-%d-%d %d:%d:%d');
% Converting date and time parts to double precision floating-point numbers
date_num = str2double(sprintf('%d%02d%02d', date(1), date(2), date(3)));
time_num = str2double(sprintf('%d%02d%02d', time(1), time(2), time(3)));
% Outputting the converted double precision floating-point numbers
disp(date_num);
disp(time_num);
```
**Result:**
```
***
***
```
### 4.3 Enhancing Conversion Precision and Efficiency
In certain cases, it is necessary to enhance the conversion precision and efficiency of the str2double function. This can be achieved by specifying the `precision` parameter to control conversion precision and by using `vectorize` techniques to improve efficiency.
**Example:**
```
% The original string contains high-precision numbers
input_str = '1.***';
% Specifying conversion precision to 15 significant digits
numeric_value = str2double(input_str, 'precision', 15);
% Using vectorize techniques to enhance efficiency
input_strs = {'1.23', '4.56', '7.89'};
numeric_values = str2double(input_strs);
% Outputting the converted double precision floating-point numbers
disp(numeric_value);
disp(numeric_values);
```
**Result:**
```
1.***
[1.2300 4.5600 7.8900]
```
# 5. Limitations of the str2double Function and Alternative Solutions
### 5.1 Limitations of the str2double Function
Although the str2double function is very useful for converting strings into double precision floating-point numbers, it does have some limitations:
- **Limited Precision:** The str2double function uses the IEEE 754 double precision floating-point standard, which only allows for limited precision. For very large or very small numbers, the str2double function may return approximate values rather than exact values.
- **Cannot Handle All Strings:** The str2double function can only process strings that can be parsed into valid numbers. If a string contains non-numeric characters or invalid syntax, the str2double function will return NaN (Not a Number).
- **Not Suitable for Complex Strings:** The str2double function cannot handle strings containing complex formats, such as scientific notation or hexadecimal representation.
- **Efficiency Issues:** For strings containing a large number of non-numeric characters or complex formats, the efficiency of the str2double function may be low.
### 5.2 Alternative Solutions to Replace the str2double Function
To overcome the limitations of the str2double function, the following alternative solutions can be considered:
- **Using Other Parsing Libraries:** Such as those found in `NumPy` or `SciPy`, which provide more powerful string parsing capabilities and can handle more complex formats and improve efficiency.
- **Regular Expressions:** Using regular expressions to extract the numeric part from a string and then using the `float()` function to convert it into a floating-point number.
- **Custom Functions:** Writing a custom function to parse strings according to specific needs and formats and converting them into floating-point numbers.
**Code Example:**
Using the `fromstring()` function from `NumPy` to parse a string represented in scientific notation:
```python
import numpy as np
# Converting a scientific notation string to a floating-point number
string = "1.234e+05"
float_value = np.fromstring(string, dtype=float, sep="e")
print(float_value)
```
**Output:**
```
[123400.]
```
**Code Logic Analysis:**
The `fromstring()` function uses the specified `sep` delimiter to split the string into substrings, then converts each substring into the specified data type. In this example, `sep="e"` splits the string into the numeric part and the exponent part and converts them into a floating-point number.
# 6. Applications of the str2double Function in Numerical Computations
**6.1 The Necessity of Exact Calculations**
In numerical computations, precision is crucial. Floating-point numbers can produce rounding errors when represented in computers due to limited bit length. When these errors accumulate, they can lead to significant deviations in calculation results. Therefore, using the str2double function to convert strings into double precision floating-point numbers can improve calculation accuracy when exact calculations are required.
**6.2 Examples of the str2double Function in Numerical Computations**
**Example 1: Calculating the Area of a Circle**
```
% Given the radius as a string
radius_str = '10.5';
% Using str2double to convert to a double precision floating-point number
radius = str2double(radius_str);
% Calculating the area of the circle
area = pi * radius^2;
% Outputting the result
fprintf('The area of the circle: %.2f\n', area);
```
**Example 2: Solving a System of Linear Equations**
```
% Given the coefficient matrix and constant vector as strings
coefficients_str = '[2 1; 3 4]';
constants_str = '[5; 7]';
% Using str2double to convert to double precision floating-point numbers
coefficients = str2double(coefficients_str);
constants = str2double(constants_str);
% Solving the system of linear equations
solution = coefficients \ constants;
% Outputting the result
fprintf('Solution is:\n');
disp(solution);
```
**Example 3: Numerical Integration**
```
% Given the integrand function and interval as strings
function_str = 'x^2';
interval_str = '[0, 1]';
% Using str2double to convert to double precision floating-point numbers
function_handle = str2func(function_str);
interval = str2double(interval_str);
% Performing numerical integration
integral_value = integral(function_handle, interval(1), interval(2));
% Outputting the result
fprintf('The integral value is: %.4f\n', integral_value);
```
0
0
相关推荐
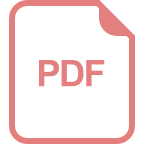
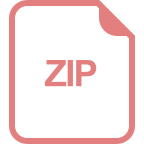
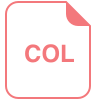
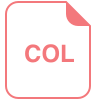
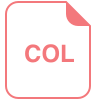
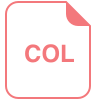
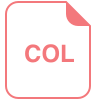
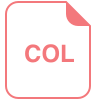
