MATLAB's disp Function: Easily Output Variables and Expressions, A Debugging Essential
发布时间: 2024-09-13 21:03:58 阅读量: 24 订阅数: 24 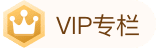
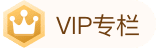
# Introduction to the disp Function in MATLAB: Effortlessly Output Variables and Expressions, a Debugging Essential
The disp function in MATLAB is a powerful utility designed for displaying the values of variables and expressions. It facilates the viewing and debugging of programs by effortlessly outputting data to the command window. With its simple and intuitive syntax, disp requires only one argument to output a variable or expression. The fundamental syntax is as follows:
```matlab
disp(x)
```
Here, x represents the variable or expression to be output. The disp function will display the value of x or the result of the expression in the command window.
# 2. disp Function Syntax and Parameters
### 2.1 Basic Syntax
The fundamental syntax of the disp function in MATLAB is as follows:
```
disp(x)
```
Where x is the variable or expression to be output.
### 2.2 Optional Parameters
The disp function also offers several optional parameters for controlling output formats and behavior. These parameters include:
| Parameter | Description |
|---|---|
| `'size'` | Outputs the matrix size format |
| `'compact'` | Outputs in a compact format, without line breaks |
| `'loose'` | Outputs in a loose format, with line breaks |
| `'double'` | Outputs in double precision format |
| `'single'` | Outputs in single precision format |
| `'hex'` | Outputs in hexadecimal format |
| `'short'` | Outputs in a short format, omitting unnecessary spaces |
| `'long'` | Outputs in a detailed format, displaying all details |
| `'rat'` | Outputs in rational number format |
| `'fixed'` | Outputs in fixed-point format |
| `'scientific'` | Outputs in scientific notation format |
| `'hyperlink'` | Converts output into a hyperlink |
| `'newline'` | Adds a newline character at the end of the output |
**Code Block:**
```
% Output variable value
x = 10;
disp(x);
% Output expression result
y = x + 5;
disp(y);
% Output formatted data
z = [1, 2, 3; 4, 5, 6];
disp(['Matrix z: ', num2str(z)]);
```
**Logical Analysis:**
* The first line outputs the value of variable x, resulting in 10.
* The second line outputs the result of the expression y = x + 5, resulting in 15.
* The third line uses the num2str function to convert matrix z into a string and uses the disp function to output the formatted data, resulting in:
```
Matrix z: [1 2 3; 4 5 6]
```
# 3. disp Function Applications
The disp function has a wide range of applications in MATLAB. It can be used to output variable values, expression results, and formatted data.
### 3.1 Output Variable Values
The most basic function of the disp function is to output variable values. The syntax is as follows:
```matlab
disp(variable_name)
```
Here, `variable_name` is the name of the variable to be output. For example:
```matlab
x = 10;
disp(x)
```
Output result:
0
0
相关推荐
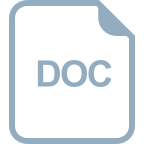
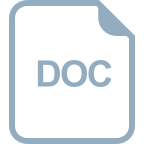
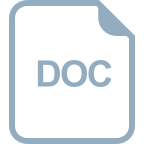
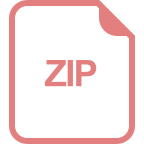
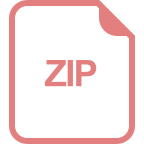
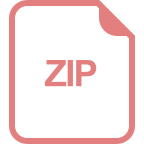
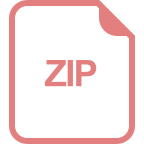
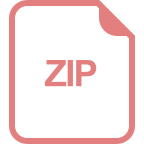
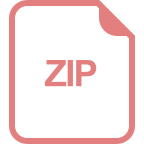