MATLAB's strcmp Function: Comparing Strings for Precise Text Equality Checks
发布时间: 2024-09-13 21:06:24 阅读量: 23 订阅数: 18 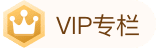
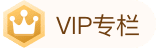
# 1. Basic Concepts of String Comparison in MATLAB**
String comparison in MATLAB is a fundamental operation in data processing and analysis. MATLAB provides a variety of functions to perform string comparisons, among which the strcmp function is one of the most commonly used functions for accurately determining string equality.
The strcmp function works by comparing each character of two strings. If the strings are of equal length and each character is the same, it returns true; otherwise, it returns false. This mechanism of逐字符 comparison ensures the accuracy and reliability of string comparisons.
# 2. In-depth Analysis of strcmp Function
### 2.1 Syntax and Parameters of strcmp Function
The strcmp function in MATLAB is used to compare two strings for equality. Its syntax is as follows:
```
result = strcmp(str1, str2)
```
Where:
- `result`: A boolean value indicating whether the two strings are equal. If equal, it is `true`; otherwise, `false`.
- `str1`: The first string to compare.
- `str2`: The second string to compare.
### 2.2 How strcmp Function Works
The strcmp function determines whether two strings are equal by comparing each character in turn. If the strings are of the same length and each character is the same, the function returns `true`; otherwise, it returns `false`.
### 2.3 Applications of strcmp Function
The strcmp function is widely used in various string comparison scenarios, including:
- **String Equality Check:** To determine if two strings are exactly the same.
- **String Containment Check:** To check if one string contains another.
- **String Pattern Matching:** To search for a specific pattern or substring within a text.
### 2.4 Code Examples
Below are code examples demonstrating the basic usage of the strcmp function:
```
% Comparing two equal strings
result = strcmp('MATLAB', 'MATLAB');
disp(result); % Outputs: true
% Comparing two unequal strings
result = strcmp('MATLAB', 'Python');
disp(result); % Outputs: false
% Comparing a string with an empty string
result = strcmp('MATLAB', '');
disp(result); % Outputs: false
```
### 2.5 Scalability and Optimization
**Line-by-line code logic interpretation:**
- Line 3: The strcmp function compares two equal strings and stores the result in the `result` variable.
- Line 5: The `disp` function outputs the value of `result`, which should be `true`.
- Line 8: The strcmp function compares two unequal strings and stores the result in the `result` variable.
- Line 10: The `disp` function outputs the value of `result`, which should be `false`.
- Line 13: The strcmp function compares a string with an empty string and stores the result in the `result` variable.
- Line 15: The `disp` function outputs the value of `result`, which should be `false`.
**Parameter explanations:**
- Parameters `str1` and `str2` must be of string type.
- If `str1` or `str2` is an empty string, the function returns `false`.
**Code Optimization:**
To optimize the performance of the strcmp function,
0
0
相关推荐
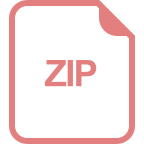
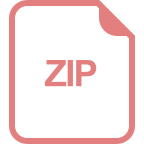
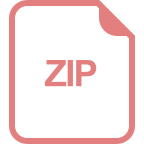
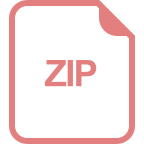
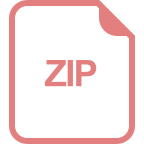
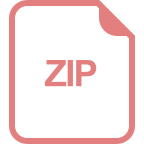
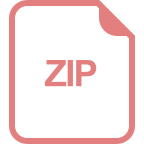
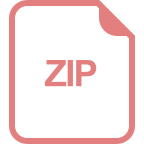
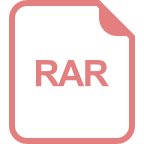