MATLAB's findstr Function: Finding Substrings in Strings, Rapidly Locating Key Information
发布时间: 2024-09-13 21:11:58 阅读量: 25 订阅数: 25 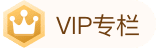
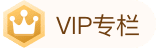
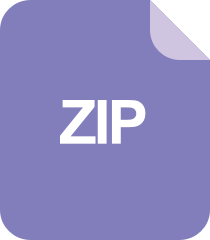
findstr2:用于具有更好多行支持的 windows 的 findstr 命令行工具
# Introduction to MATLAB's findstr Function: Locating Key Information in Strings
The findstr function is a powerful tool in MATLAB for searching for substrings within strings. It works by returning the position of the first occurrence of the substring within the main string. The findstr function is incredibly useful for various string processing tasks such as finding specific patterns, extracting substrings, and counting character occurrences. In this chapter, we will introduce the basic concepts of the findstr function, including its syntax, parameters, and applications in MATLAB.
# Syntax and Parameters of the findstr Function
### 2.1 The Syntax Structure of findstr
The syntax structure of the findstr function is as follows:
```matlab
findstr(str, pattern)
```
Where:
* `str`: The string or character vector to be searched.
* `pattern`: The pattern or substring to be found.
### 2.2 Detailed Explanation of findstr Parameters
A detailed explanation of the findstr parameters is as follows:
| Parameter | Data Type | Description |
|---|---|---|
| `str` | String or character vector | The string or character vector to be searched. |
| `pattern` | String or character vector | The pattern or substring to be found. |
| `direction` | String (Optional) | Specifies the matching direction, either 'normal' (left to right) or 'reverse' (right to left). |
| `ignorecase` | Logical value (Optional) | Specifies whether to ignore case sensitivity, with the default value being false (case-sensitive). |
| `endings` | Logical value (Optional) | Specifies whether the pattern should be treated as an end-of-string, with the default value being false (not considered as an end). |
**Code Block 1: Basic Usage of the findstr Function**
```matlab
str = 'Hello, MATLAB!';
pattern = 'MATLAB';
result = findstr(str, pattern);
disp(result);
```
**Logical Analysis:**
* The `str` variable stores the string to be searched "Hello, MATLAB!".
* The `pattern` variable stores the pattern to be found "MATLAB".
* The `findstr` function returns a vector containing the indices where the pattern occurs in the string.
* The `disp` function outputs the result, displaying the index of the pattern in the string as 8.
**Parameter Explanation:**
* The `direction` parameter is unspecified, with the default value of 'normal', indicating a left-to-right search.
* The `ignorecase` parameter is unspecified, with the default value of false, indicating case sensitivity.
* The `endings` parameter is unspecified, with the default value of false, indicating the pattern is not treated as an end-of-string.
# 3.1 Basic Usage of String Matching
The most fundamental function of the findstr function is to find the first occurrence of a substring within a string. The basic syntax is as follows:
```matlab
result = findstr(str, pattern)
```
Where:
- `str`: The string to be searched.
- `pattern`: The substring to be found.
- `result`: A vector containing the indices of the matches. If no match is found, an empty vector is returned.
**Example 1: Finding a Substring**
```matlab
str = 'Hello, world!';
pattern = 'world';
result = findstr(str, pattern)
```
Output:
```
result = 8
```
In this example, the findstr function finds the substring 'world' in the string `str`, with its first occurrence index being 8.
**Example 2: Finding Multiple Substrings**
The findstr function can also search for multiple substrings simultaneously. Simply pass the substrings as a cell array or string array to the `pattern` parameter.
```matlab
str = 'Hello, world! This is a test.';
patterns = {'world', 'test'};
results = findstr(str, patterns)
```
Output:
```
results = [8, 22]
```
In this example, the findstr function finds two substrings, 'world' and 'test', in the string `str`, and returns their first occurrence indices.
### 3.2 Specifying Matching Direction and Case Sensitivity
The findstr function provides two optional parameters to specify the matching direction and case sensitivity:
- `direc
0
0
相关推荐
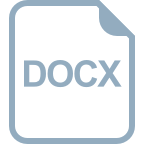
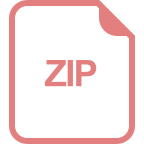
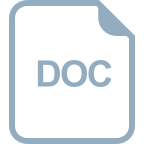
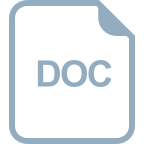
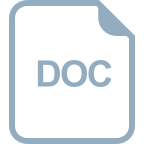
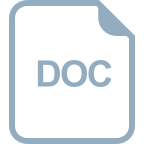
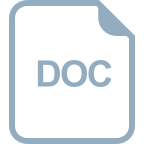
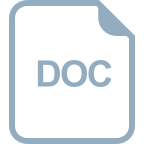