MATLAB's strtok Function: Splitting Strings with Delimiters for More Precise Text Parsing
发布时间: 2024-09-13 21:16:55 阅读量: 32 订阅数: 32 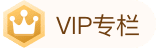
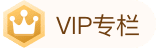
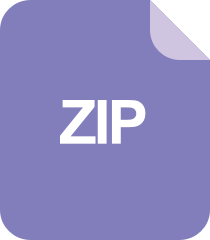
csplit:单个标头C库,轻松处理替换strtok的拆分和处理字符串
# Chapter 1: Overview of String Operations in MATLAB
MATLAB offers a rich set of functions for string manipulation, among which the `strtok` function stands out as a powerful tool for delimiter-driven string splitting. This chapter will introduce the basic syntax, usage, and return results of the `strtok` function, laying the groundwork for more in-depth exploration in subsequent sections.
# Chapter 2: The strtok Function - Delimiter-Driven String Splitting
## 2.1 Basic Syntax and Usage of the strtok Function
In MATLAB, the strtok function is used to split a string into multiple substrings based on specified **delimiters**. Its basic syntax is as follows:
```
token = strtok(str, delim)
```
Where:
- `str`: The string to be split.
- `delim`: The delimiter, which can be a single character or a character vector.
The strtok function works by scanning the string character by character, and when it encounters a delimiter, it splits the string into two substrings at that point:
- **Token**: The substring before the delimiter.
- **Remainder**: The substring after the delimiter.
## 2.2 Setting and Impact of Delimiters
The setting of delimiters has a significant impact on the results of string splitting. Delimiters can be:
- **Single Characters**: For example, using a comma (`,`) as a delimiter to split a string into comma-separated fields.
- **Character Vectors**: For example, using the string `"-, "` as a delimiter to split a string into fields separated by a hyphen (-) or a comma (,).
## 2.3 Return Results of the strtok Function
The strtok function returns the split **tokens**. If the function reaches the end of the string, it returns an empty string.
**Code Example:**
```
str = 'MATLAB, is, a, programming, language';
delim = ', ';
% Split the string
token = strtok(str, delim);
% Print tokens one by one
while ~isempty(token)
disp(token);
token = strtok(delim); % Continue splitting the remainder of the string
end
```
**Execution Logic Explanation:**
This code uses a comma and space as the delimiter to split the string into comma-separated fields. The strtok function scans the string character by character and splits it whenever it encounters a delimiter. It returns the first token and then continues splitting the remainder of the string until the end of the string is reached.
# Chapter 3: Practical Application of the strtok Function
### 3.1 String Splitting in Text Parsing
The strtok function plays a crucial role in text parsing, breaking down complex textual data into smaller, more manageable chunks. For example, the following code demonstrates how to use the strtok function to split a text string containing comma-separated values into individual elements:
```matlab
text_data = 'John,Doe,123 Main St,Anytown,CA,91234';
delimiter = ',';
tokens = strtok(text_data, delimiter);
while ~isempty(tokens)
disp(tokens);
tokens = strtok(tokens, delimiter);
end
```
**Code Logic Line-by-Line Explanation:**
1. The `text_data` variable stores the text string to be parsed.
2. The `delimiter` variable specifies the character used for splitting (in this case, a comma).
3. The strtok function splits the `text_data` string into tokens separated by the specified delimiter.
4. The `while` loop repeats the splitting process until the strtok function retu
0
0
相关推荐







