MATLAB's printf Function: Advanced Formatting Output, Customize Your Output Style
发布时间: 2024-09-13 20:56:15 阅读量: 19 订阅数: 18 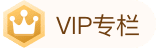
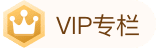
# 1. Introduction to fprintf Function
`fprintf` is a highly versatile function in MATLAB used for formatting and outputting text to the command window or files. Its basic syntax is as follows:
```matlab
fprintf(formatSpec, A, ...)
```
- `formatSpec` is a string that defines the format of the output. It can include text and format specifiers such as `%d` (integer), `%f` (floating-point number), `%s` (string), and so on.
- `A` is the variable to be output, which can be a scalar, vector, or matrix.
### Examples
1. **Outputting simple text**:
```matlab
fprintf('Hello, World!\n');
```
2. **Formatting numerical output**:
```matlab
a = 10;
b = 3.14159;
fprintf('The value of a is %d and the value of b is %.2f\n', a, b);
```
3. **Output to a file**:
```matlab
fileID = fopen('output.txt', 'w');
fprintf(fileID, 'The value of a is %d and the value of b is %.2f\n', a, b);
fclose(fileID);
```
### Common Format Specifiers
- `%d`: Integer
- `%f`: Floating-point number
- `%.nf`: Floating-point number with n decimal places
- `%s`: String
- `%c`: Single character
### Tips
- `\n` is used for new lines in the format string.
- `fopen` and `fclose` can be used to write output to files, not just the command window.
`fprintf` is a powerful tool that enables users to output data in a readable manner, making it particularly useful for debugging and report generation.
# 2. Format Specifiers of fprintf Function
The format specifiers for the printf function are special character sequences that specify the output format. They allow you to control the width, alignment, decimal point, and precision of the output.
The format specifiers for the `fprintf` function are used to control the output format. Here are some common format specifiers and their detailed explanations:
### 2.1 Basic Format Specifiers
- `%d` or `%i`: Output an integer (decimal).
- `%u`: Output an unsigned integer.
- `%f`: Output a floating-point number (default six decimal places).
- `%e` or `%E`: Output a floating-point number in scientific notation (lowercase or uppercase).
- `%g` or `%G`: Automatically choose between `%f` or `%e` format based on the size of the number.
```matlab
a = 42;
b = 3.14159;
str = 'World';
fprintf('Integer: %d\n', a); % Output integer
fprintf('Float: %f\n', b); % Output floating-point number
fprintf('String: %s\n', str); % Output string
```
**Output Result:**
```
Integer: 42
Float: 3.141590
String: World
```
### 2.2 Numeric Format Specifiers
- `%d`: Decimal integer.
- `%o`: Octal integer.
- `%x` or `%X`: Hexadecimal integer (lowercase or uppercase).
- `%f`: Floating-point number, default six decimal places.
- `%e`: Scientific notation (lowercase).
- `%E`: Scientific notation (uppercase).
```matlab
decimal = 255;
octal = 255;
hexadecimal = 255;
fprintf('Decimal: %d\n', decimal); % Decimal
fprintf('Octal: %o\n', octal); % Octal
fprintf('Hexadecimal: %x\n', hexadecimal); % Hexadecimal (lowercase)
fprintf('Hexadecimal: %X\n', hexadecimal); % Hexadecimal (uppercase)
```
**Output Result:**
```
Decimal: 255
Octal: 377
Hexadecimal: ff
Hexadecimal: FF
```
### 2.3 String Format Specifiers
- `%s`: Output a string.
- `%c`: Output a single character.
```matlab
char = 'A';
str = 'Hello, World!';
fprintf('Character: %c\n', char); % Output single character
fprintf('String: %s\n', str); % Output string
```
**Output Result:**
```
Character: A
String: Hello, World!
```
### 2.4 Advanced Format Specifiers
- `%p`: Output the address of a pointer.
- `%n`: Do not output anything, but store the current number of characters into the s
0
0
相关推荐
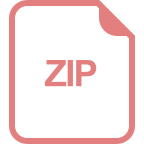
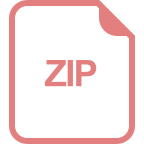
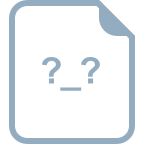
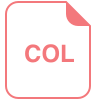
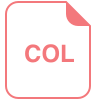
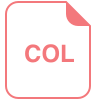
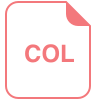
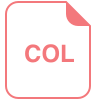
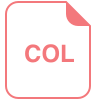