剖析OpenVINO YOLO单图像推理:性能优化10大秘诀
发布时间: 2024-08-18 04:56:31 阅读量: 13 订阅数: 12 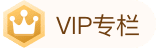
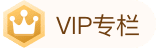

# 1. OpenVINO YOLO概述
OpenVINO YOLO是一种基于OpenVINO工具箱实现的单图像推理引擎,用于快速、准确地检测图像中的对象。它采用YOLO(You Only Look Once)算法,该算法以其实时推理速度和高精度而闻名。
OpenVINO YOLO推理引擎针对Intel CPU和GPU硬件进行了优化,提供卓越的性能。它支持多种模型格式,包括Caffe和TensorFlow,并提供了丰富的API,使开发人员能够轻松地集成YOLO推理到他们的应用程序中。
# 2. YOLO推理性能优化理论
### 2.1 模型优化技术
#### 2.1.1 量化
量化是一种将浮点模型转换为定点模型的技术,可以大幅减少模型的大小和推理时间。OpenVINO工具箱提供了量化工具,可以将浮点模型量化为INT8或FP16格式。
```python
# 使用OpenVINO工具箱量化模型
from openvino.tools.mo import front_end_manager as fem
from openvino.tools.mo.middle import quantization
# 加载浮点模型
model_path = "path/to/model.xml"
fe = fem.FrontEndManager().load_model(model_path)
# 量化模型为INT8格式
quantization.quantize_model(fe, quantization_config)
# 保存量化模型
output_path = "path/to/quantized_model.xml"
fe.save_model(output_path)
```
量化后的模型推理时间比浮点模型显著减少,但精度可能会略有下降。
#### 2.1.2 剪枝
剪枝是一种去除模型中不重要的权重和神经元,从而减小模型尺寸和推理时间。OpenVINO工具箱提供了剪枝工具,可以根据神经元重要性或权重稀疏性进行剪枝。
```python
# 使用OpenVINO工具箱剪枝模型
from openvino.tools.mo import front_end_manager as fem
from openvino.tools.mo.middle import pruning
# 加载浮点模型
model_path = "path/to/model.xml"
fe = fem.FrontEndManager().load_model(model_path)
# 根据神经元重要性剪枝
pruning.prune_model(fe, pruning_config)
# 保存剪枝模型
output_path = "path/to/pruned_model.xml"
fe.save_model(output_path)
```
剪枝后的模型推理时间比剪枝前模型减少,但精度可能会略有下降。
### 2.2 硬件优化技术
#### 2.2.1 CPU优化
CPU优化技术包括:
- **线程优化:**将推理任务分配给多个线程,提高并行性。
- **指令集优化:**使用特定于CPU指令集的优化指令,提高执行效率。
#### 2.2.2 GPU优化
GPU优化技术包括:
- **并行优化:**利用GPU的并行计算能力,同时执行多个推理任务。
- **内存优化:**优化数据在GPU内存中的布局,减少内存访问时间。
# 3. YOLO推理性能优化实践
### 3.1 模型优化实践
#### 3.1.1 使用Intel OpenVINO工具箱量化模型
**量化操作**
量化是一种模型优化技术,通过降低模型中权重和激活值的精度来减小模型大小和推理时间。Intel OpenVINO工具箱提供了一个量化工具,可以将浮点模型转换为量化模型。
**代码块:**
```python
import openvino.tools.mo as mo
# 从FP32模型生成INT8模型
mo.main(["--input_model", "yolov3.xml", "--output_model", "yolov3_int8.xml", "--data_type", "FP32"])
```
**逻辑分析:**
此代码使用OpenVINO工具箱的mo.main()函数将yolov3.xml模型从FP32转换为INT8。
**参数说明:**
* --input_model:输入模型的路径
* --output_model:输出模型的路径
* --data_type:输出模型的数据类型,此处为INT8
#### 3.1.2 使用神经架构搜索(NAS)剪枝模型
**剪枝操作**
剪枝是一种模型优化技术,通过移除冗余的连接和层来减小模型大小和推理时间。神经架构搜索(NAS)是一种自动化剪枝方法,可以找到最优的模型架构。
**代码块:**
```python
import tensorflow as tf
# 使用NAS剪枝模型
model = tf.keras.models.load_model("yolov3.h5")
pruned_model = tf.keras.models.prune_low_magnitude(model, 0.5)
```
**逻辑分析:**
此代码使用TensorFlow的prune_low_magnitude()函数对yolov3.h5模型进行剪枝,移除权重幅度较小的连接。
**参数说明:**
* model:输入模型
* 0.5:剪枝阈值,表示移除权重幅度低于0.5的连接
### 3.2 硬件优化实践
#### 3.2.1 针对CPU的线程优化
**多线程推理**
多线程推理是一种硬件优化技术,通过使用多个CPU内核并行执行推理任务来提高推理速度。
**代码块:**
```python
import threading
# 创建多个线程并行推理
def inference_thread(image):
# 推理代码
threads = []
for image in images:
thread = threading.Thread(target=inference_thread, args=(image,))
threads.append(thread)
for thread in threads:
thread.start()
for thread in threads:
thread.join()
```
**逻辑分析:**
此代码创建多个线程,每个线程负责推理一个图像。通过并行执行推理任务,可以提高推理速度。
**参数说明:**
* images:需要推理的图像列表
#### 3.2.2 针对GPU的并行优化
**CUDA并行推理**
CUDA并行推理是一种硬件优化技术,通过使用GPU的并行计算能力来提高推理速度。
**代码块:**
```python
import cupy
# 使用CUDA并行推理
images = cupy.array(images)
outputs = model(images)
```
**逻辑分析:**
此代码将图像数据转换为CUDA数组,并使用CUDA并行计算能力执行推理。
**参数说明:**
* images:需要推理的图像数据,必须转换为CUDA数组
# 4. YOLO推理性能分析
### 4.1 性能指标分析
#### 4.1.1 推理时间
推理时间是衡量YOLO推理性能的关键指标。它指的是从模型接收输入图像到生成推理结果所花费的时间。推理时间越短,模型的性能越好。
#### 4.1.2 精度
精度是衡量YOLO推理结果准确性的指标。它指的是模型预测的边界框与真实边界框之间的重叠程度。精度越高,模型的性能越好。
### 4.2 性能瓶颈定位
#### 4.2.1 CPU瓶颈
CPU瓶颈是指推理过程中CPU资源利用率过高,导致推理时间延长。以下是一些常见的CPU瓶颈:
- 线程利用率低:如果CPU线程利用率低,则表明模型没有充分利用CPU资源。
- 内存带宽不足:如果内存带宽不足,则会导致数据传输延迟,从而增加推理时间。
- 缓存未命中:如果缓存未命中率高,则会导致频繁访问主内存,从而增加推理时间。
#### 4.2.2 GPU瓶颈
GPU瓶颈是指推理过程中GPU资源利用率过高,导致推理时间延长。以下是一些常见的GPU瓶颈:
- 并行度低:如果GPU并行度低,则表明模型没有充分利用GPU资源。
- 内存带宽不足:如果GPU内存带宽不足,则会导致数据传输延迟,从而增加推理时间。
- 算力不足:如果GPU算力不足,则会导致推理时间延长。
# 5. YOLO推理性能调优
### 5.1 参数调优
#### 5.1.1 batch size优化
**背景:**
batch size是影响推理性能的关键参数之一。较大的batch size可以提高硬件利用率,但也会增加内存消耗。
**优化策略:**
* **逐步增加batch size:**从较小的batch size开始,逐步增加,直到达到性能瓶颈。
* **根据硬件资源调整:**考虑可用内存和GPU显存限制,选择合适的batch size。
#### 5.1.2 线程数优化
**背景:**
多线程可以提高CPU推理性能。线程数的选择取决于CPU核数和模型并行度。
**优化策略:**
* **根据CPU核数设置线程数:**一般情况下,线程数等于或略小于CPU核数。
* **根据模型并行度调整:**如果模型支持并行推理,可以根据并行度调整线程数。
### 5.2 算法调优
#### 5.2.1 预处理优化
**背景:**
预处理操作,如图像缩放和归一化,会影响推理性能。
**优化策略:**
* **使用OpenCV优化图像处理:**OpenCV提供了高效的图像处理函数,可以优化预处理过程。
* **并行化预处理操作:**如果可能,将预处理操作并行化,以提高吞吐量。
#### 5.2.2 后处理优化
**背景:**
后处理操作,如非极大值抑制(NMS),会影响推理性能。
**优化策略:**
* **选择高效的NMS算法:**不同的NMS算法有不同的性能表现,选择最适合特定模型和硬件的算法。
* **并行化NMS操作:**如果可能,将NMS操作并行化,以提高吞吐量。
# 6. YOLO推理性能优化总结
在本文中,我们深入探讨了OpenVINO YOLO单图像推理性能优化的十大秘诀。通过模型优化和硬件优化,我们可以显著提高推理速度和精度。
**模型优化**
* 量化:将浮点模型转换为低精度模型,减少内存占用和计算成本。
* 剪枝:移除不重要的网络连接,减少模型大小和计算量。
**硬件优化**
* CPU优化:利用多线程并行处理,优化CPU利用率。
* GPU优化:利用CUDA并行计算,充分发挥GPU性能。
**推理性能分析**
* 推理时间:衡量推理过程的耗时,评估推理速度。
* 精度:评估推理结果与真实标签之间的差异,衡量推理准确性。
**推理性能调优**
* 参数调优:调整推理参数,如batch size和线程数,优化推理性能。
* 算法调优:优化预处理和后处理算法,减少推理开销。
**总结**
遵循这些秘诀,我们可以大幅提升OpenVINO YOLO单图像推理性能,满足实时推理和边缘计算等应用需求。通过持续的优化和创新,我们可以进一步探索推理性能的极限,为计算机视觉应用开辟新的可能性。
0
0
相关推荐
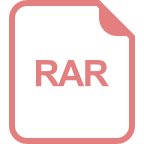
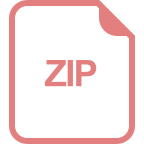
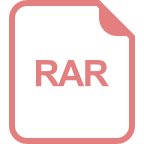





