Basic Concepts of HTTP Protocol in LabVIEW
发布时间: 2024-09-14 21:23:38 阅读量: 27 订阅数: 20 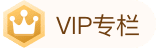
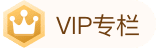
# Chapter 1: An Overview of the HTTP Protocol
In this chapter, we will introduce the fundamental concepts and development history of the HTTP protocol, helping readers to establish a comprehensive understanding of HTTP.
# Chapter 2: HTTP Communication in LabVIEW
Implementing HTTP communication in LabVIEW is crucial as it enables us to easily interact with various network services for data exchange. Below, we will introduce the basic principles of HTTP communication in LabVIEW, the concepts of HTTP Client and HTTP Server, and how to implement HTTP communication in LabVIEW.
### 2.1 The Basic Principles of HTTP Communication in LabVIEW
The fundamental principle of HTTP communication involves the client (Client) sending an HTTP request to the server (Server), which processes the request and returns an HTTP response to the client. In LabVIEW, we can use the HTTP Client VI to construct requests and send them to the server, and the HTTP Server VI to listen for and process client requests.
### 2.2 Concepts of HTTP Client and HTTP Server
- **HTTP Client:** Responsible for sending HTTP requests to the server and receiving the HTTP responses returned by the server. In LabVIEW, the HTTP Client VI can help us construct different types of HTTP requests, such as GET, POST, etc.
- **HTTP Server:** Responsible for receiving HTTP requests from clients and returning HTTP responses after processing. In LabVIEW, the HTTP Server VI can be used to listen on a specific port, receive client requests, and respond accordingly.
### 2.3 How to Implement HTTP Communication in LabVIEW
Implementing HTTP communication in LabVIEW typically involves the following steps:
1. **Create an HTTP Client VI:** Use the HTTP Client VI to create an HTTP request, including setting request method, URL, request headers, request body, and other parameters.
2. **Send an HTTP Request:** Invoke the method of the HTTP Client VI to send the HTTP request to the target server.
3. **Create an HTTP Server VI:** If you need to set up an HTTP server, you can use the HTTP Server VI to create a simple HTTP server that listens on a specified port.
4. **Process HTTP Requests and Responses:** Add processing logic to the HTTP Server VI to handle client requests accordingly and return HTTP responses.
Through these steps, we can implement HTTP communication in LabVIEW, achieving data transmission and interaction. In the following chapters, we will delve into the structure of HTTP requests and responses and how to handle HTTP response data.
# Chapter 3: HTTP Requests and Responses
HTTP requests and responses are the core components of HTTP communication. Through the interaction of requests and responses, clients and servers can exchange data and communicate. In LabVIEW, we can also achieve data exchange with other devices or systems through HTTP requests and responses. Let's delve into the details of HTTP requests and responses.
### 3.1 The Structure and Format of HTTP Requests
When a client sends a request to the server in HTTP communication, it typically includes the following important parts:
- **Request Line (Request Line):** Includes the request method (GET, POST, etc.), the URL path of the requested resource, and the protocol version information.
For example: `GET /index.html HTTP/1.1`
- **Request Headers (Headers):** Contains various additional information about the request, such as Accept, User-Agent, etc., used to describe some attributes and requirements of the client.
For example:
```
Host: ***
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3
```
- **Request Body (Entity Body):** For POST requests, there is usually a request body used to transmit data sent by the client to the server.
For example:
```json
{"key1": "value1", "key2": "value2"}
```
### 3.2 Analyzing Important Parameters in HTTP Requests
- **Request Method (Method):** Common request methods include GET (fetch resource), POST (submit data), PUT (update resource), DELETE (delete resource), etc.
- **URL Path (Uniform Resource Locator):** Indicates the path of the requested resource, which can be an absolute or relative path.
- **Request Header Parameters (Headers):** Contains various request-related information, such as User-Agent (user agent), Content-Type (request content type), etc., which describe and supplement the request.
### 3.3 The Structure and Status Codes of HTTP Responses
When the server receives a client's request, it returns an HTTP response that includes the following important contents:
- **Status Line (Status Line):** Includes the protocol version (such as HTTP/1.1), status code, and status message.
For example: `HTTP/1.1 200 OK`
- **Response Headers (Headers):** Contains various additional information about the response, such as Content-Type, Content-Length, etc., describing the information returned by the server.
For example:
```
Content-Type: text/html; charset=utf-8
Content-Length: 1234
```
- **Response Body (Entity Body):** For GET requests, the returned data is usually in the response body and can be in HTML, JSON, XML, etc., formats.
By understanding the structure and content of HTTP requests and responses, we can better handle HTTP communication in LabVIEW and implement data exchange functionality. Next, we will continue to explore methods for making HTTP requests and handling responses in LabVIEW.
# Chapter 4: Using HTTP APIs in LabVIEW
To implement HTTP communication in LabVIEW, we typically use the HTTP Client to send HTTP requests and then receive the response data from the HTTP Server. Below, we will introduce how to use HTTP APIs for GET and POST requests in LabVIEW and how to handle the response data.
### 4.1 Using LabVIEW for HTTP GET Requests
Performing an HTTP GET request in LabVIEW is very simple. First, we need to create an HTTP Client object, set the request URL and other parameters, send the request, and obtain the response data.
```labview
// Create an HTTP Client object
client = HTTP Client Open()
// Set request parameters
url = "***"
method = "GET"
headers = ""
body = ""
// Send an HTTP GET request
response = HTTP Client Get(client, url, method, headers, body)
// Get response data
status_code = HTTP Client Get Status(response)
data = HTTP Client Get Body(response)
// Close the HTTP Client object
HTTP Client Close(client)
```
With the above code, we successfully sent an HTTP GET request and obtained the data returned by the server.
### 4.2 Using LabVIEW for HTTP POST Requests
Like GET requests, using LabVIEW for HTTP POST requests is also easy. We only need to set the request method to POST and add the data to be transmitted in the body.
```labview
// Create an HTTP Client object
client = HTTP Client Open()
// Set request parameters
url = "***"
method = "POST"
headers = "Content-Type: application/json"
body = "{\"key\": \"value\"}"
// Send an HTTP POST request
response = HTTP Client Post(client, url, method, headers, body)
// Get response data
status_code = HTTP Client Post Status(response)
data = HTTP Client Post Body(response)
// Close the HTTP Client object
HTTP Client Close(client)
```
With the above code, we successfully sent an HTTP POST request and obtained the data returned by the server.
### 4.3 Handling HTTP Response Data
After obtaining HTTP response data, we also need to process it. Depending on the actual situation, we can parse JSON or XML data or directly display the data on the Front Panel for users to view.
```labview
// Parse JSON format response data
json_data = Parse JSON Data(data)
key = Get JSON Element(json_data, "key")
value = Get JSON Element(json_data, "value")
// Display data on the Front Panel
Set Control Value(String Indicator, value)
```
With the above code, we have successfully parsed the JSON format response data and displayed its content on the Front Panel.
Through these sample codes, we can use HTTP APIs in LabVIEW to send GET and POST requests and handle the response data returned by the server. This provides a convenient way to interact with remote servers for data exchange in our project development.
# Chapter 5: HTTP Security and Encryption
In actual project development and data transmission processes, ensuring the security of communication data is crucial. In the HTTP protocol, there are some security mechanisms and encryption methods that can help us ensure that the communication content is not tampered with or stolen. This chapter will focus on the content related to HTTP security and encryption.
### 5.1 The Difference and Role of HTTP and HTTPS
**HTTP (HyperText Transfer Protocol)** is an application layer protocol used for transferring hypertext data, usually based on TCP connections. However, HTTP has obvious security vulnerabilities, such as the risk of information eavesdropping and tampering. To ensure the security of communication data, **HTTPS (HyperText Transfer Protocol Secure)** emerged. HTTPS adds SSL/TLS protocols on the basis of HTTP, encrypting the transmitted data to ensure the confidentiality and integrity of communication.
### 5.2 The Application of SSL/TLS Protocols in HTTP
**SSL/TLS (Secure Sockets Layer/Transport Layer Security)** is a public key infrastructure (PKI) encryption protocol used to ensure the security of data transmission. In HTTP, SSL/TLS is mainly used to establish a secure encrypted channel, protecting the data transmission process from eavesdropping or tampering. With the SSL/TLS protocol, communication between clients and servers becomes more secure and reliable.
### 5.3 How to Implement HTTPS Communication in LabVIEW
To implement HTTPS communication in LabVIEW, you typically need to use an HTTP Client library that supports SSL/TLS protocols. By configuring SSL certificates, selecting appropriate encryption algorithms, and security parameters, you can set up a secure HTTPS communication channel in LabVIEW. In practical applications, it is recommended to properly set the security options of HTTPS connections to ensure the security and reliability of data transmission.
Through the content of this chapter, readers can gain a deeper understanding of knowledge related to HTTP security and encryption, providing a more comprehensive reference for actual project development and system integration.
# Chapter 6: The Future Development Trend of the HTTP Protocol
As the fundamental protocol for Web communication, the HTTP protocol is used daily by billions of devices worldwide. With the rapid development of the Internet and technological progress, the HTTP protocol is constantly evolving and improving to adapt to new application scenarios and user needs. In this chapter, we will explore the future development trends of the HTTP protocol, understanding the new features of HTTP/2, HTTP/3, and the application of the HTTP protocol in the Internet of Things and cloud computing.
### 6.1 The New Features of HTTP/2 and HTTP/3
HTTP/2 is the new generation of the HTTP/1.1 protocol, ***pared to HTTP/1.1, HTTP/2 introduces new features such as multiplexing, header compression, and server push, effectively reducing latency and improving transmission performance. HTTP/3, based on the UDP protocol, is the new generation protocol aimed at further improving network transmission efficiency and security. Through the QUIC protocol, HTTP/3 achieves faster connection establishment and reliable transmission mechanisms, suitable for high-latency and high-packet-loss network environments.
### 6.2 The Application of the HTTP Protocol in the Internet of Things and Cloud Computing
With the rapid development of Internet of Things and cloud computing technologies, the application of the HTTP protocol in fields such as intelligent devices, sensors, and cloud services is increasingly widespread. Through the HTTP protocol, devices can communicate in real-time with cloud platforms for data exchange, implementing functions such as data collection, monitoring, and control. At the same time, the HTTP interface based on the RESTful architecture also provides convenience and a standardized approach for the integration of Internet of Things devices and cloud services, promoting the rapid development of the Internet of Things industry.
### 6.3 Prospects and Suggestions for the HTTP Protocol
In the future, with the vigorous development of emerging technologies such as 5G, edge computing, and artificial intelligence, the HTTP protocol will face more challenges and opportunities. To adapt to the needs of massive connections, low latency, and high security, the HTTP protocol needs to be continuously optimized and upgraded, and deeply integrated with new technologies. It is recommended that future HTTP protocols should focus on performance optimization, security reinforcement, and ecological construction, to better meet the needs of users and enterprises, and promote the continuous development of the Internet.
0
0
相关推荐
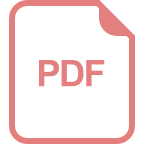
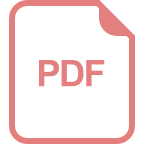
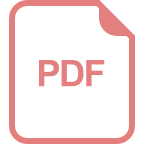
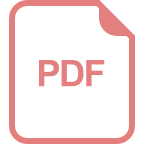
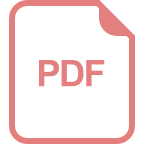
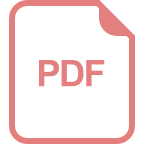