Exception and Error Handling in HTTP Requests with LabVIEW
发布时间: 2024-09-14 21:29:56 阅读量: 33 订阅数: 24 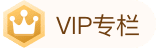
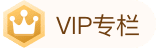
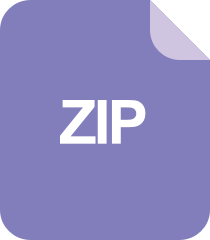
Simple client for making and logging HTTP requests and responses
# 1. Understanding the Basics of HTTP Request Handling
In this chapter, we will introduce the basics of handling HTTP requests, including the fundamental concepts of HTTP requests and responses, an overview of the HTTP request handling process in LabVIEW, and common types of HTTP request handling exceptions. A deep understanding of these basics can help us better manage potential exceptions and errors when handling HTTP requests. Let's explore each one in turn.
# 2. Handling HTTP Requests with LabVIEW
Handling HTTP requests in LabVIEW is a common task and can be achieved by sending HTTP requests and receiving HTTP responses to communicate with remote servers. In this section, we will delve into how to handle HTTP requests in LabVIEW and introduce some key operations and considerations. Let's take a look at the following content:
# ***mon Exceptions in HTTP Request Handling
When handling HTTP requests in LabVIEW, various exceptions are often encountered. Below are some possible common exception types and their handling methods:
#### 3.1 Connection Timeout and Network Issues
```python
import requests
try:
response = requests.get("***", timeout=5)
response.raise_for_status()
except requests.exceptions.Timeout:
print("Connection timed out, please check if the network connection is normal.")
except requests.exceptions.RequestException as e:
print(f"Network issue: {e}")
```
**Code Explanation:** The above Python code uses the requests library to send an HTTP GET request, setting a timeout of 5 seconds. If the connection times out, it will prompt a connection timeout message; if other network issues occur, it will print the exception information.
#### 3.2 Server Error Response Handling
```***
***.HttpURLConnection;
***.URL;
import java.io.InputStream;
import java.io.InputStreamReader;
try {
URL url = new URL("***");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
InputStream response = connection.getInputStream();
// Check HTTP status code
if (connection.getResponseCode() >= 400) {
// Handle server errors
System.out.println("Server error: " + connection.getResponseCode());
}
} catch (Exception e) {
System.err.println("An exception occurred: " + e.getMessage());
}
```
**Code Explanation:** The above Java code uses HttpURLConnection to send an HTTP request and checks the status code returned by the server. If the status code is greater than or equal to 400, it handles the server error; if an exception occurs, it prints the exception information.
#### 3.3 Data Parsing and Processing Exceptions
```go
package main
import (
"fmt"
"net/http"
"io/ioutil"
)
func main() {
response, err := http.Get("***")
if err != nil {
fmt.Println("HTTP request error:", err)
return
}
defer response.Body.Close()
body, err := ioutil.ReadAll(response.Body)
if err != nil {
fmt.Println("Data parsing error:", err)
return
}
fmt.Println("Response data:", string(body))
}
```
**Code Explanation:** The above Go language example demonstrates how to send an HTTP request and parse the response data. If an HTTP request error or data parsing error occurs, the error message will be output accordingly.
With the above example co
0
0
相关推荐
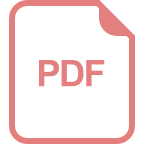
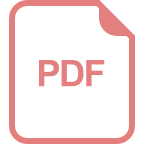
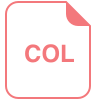
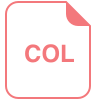
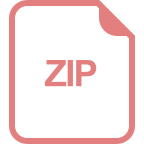
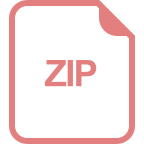
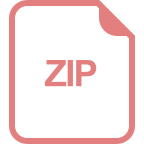
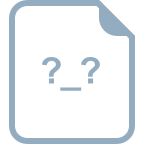