Implementing HTTP Digest Authentication in LabVIEW
发布时间: 2024-09-14 21:33:05 阅读量: 19 订阅数: 24 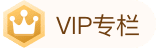
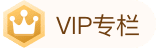
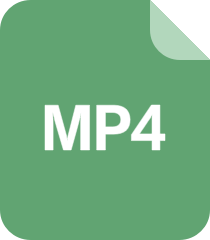
Implementing Counters in LabVIEW FPGA 中英双字幕
# 1. Introduction
In this article, we will discuss how to write a simple text processing program using Python, which will read a text file and perform basic processing and analysis. We will cover file reading, text cleaning, and tokenization, among other operations, to help you understand how to handle and analyze textual data. Let's get started!
# 2. Data Structures and Algorithms
The choice of data structures and algorithms is crucial when writing software. Good data structures and efficient algorithms can enhance the performance of a program and reduce resource consumption. This chapter will introduce some commonly used data structures and algorithms, and demonstrate their applications through practical examples.
First, let's start with arrays (Array).
```python
# Sample code: Creating and accessing arrays
array = [1, 2, 3, 4, 5]
print(array) # Outputs the entire array
print(array[2]) # Outputs the element at index 2, which is the third element
```
**Code Explanation**:
- An array containing five integers is created.
- Specific elements in the array are accessed using indices. In Python, array indexing starts at 0.
**Code Execution Results**:
```
[1, 2, 3, 4, 5]
3
```
Next, we will delve into the concept and applications of linked lists (Linked List).
# 3. Data Structures and Algorithms
In the IT domain, data structures and algorithms are fundamental knowledge. Data structures refer to how data is organized and stored in a computer, while algorithms are a series of steps and rules for solving problems. In programming, the appropriate choice of data structures and algorithms can improve program efficiency and performance.
#### 1. Arrays
Arrays are one of the simplest data structures, composed of an ordered collection of elements of the same type. In most programming languages, array elements are stored contiguously in memory, which is why arrays can be accessed quickly.
**Sample Code:**
```python
# Creating an integer array
arr = [1, 2, 3, 4, 5]
# Accessing array elements
print(arr[0]) # Outputs: 1
# Modifying array elements
arr[2] = 10
print(arr) # Outputs: [1, 2, 10, 4, 5]
# Traversing the array
for num in arr:
print(num)
```
**Code Summary**: The above examples illustrate how to create, access, modify array elements, and traverse an array.
**Results Explanation**: Running the code will output the array elements and the array after modification.
#### 2. Linked Lists
A linked list is a linear data structure where elements do not need to be stored in contiguous memory. Each element (node) contains data and a pointer to the next node.
**Sample Code:**
```python
# Defining a linked list node
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
# Creating a linked list
node1 = Node(1)
node2 = Node(
```
0
0
相关推荐
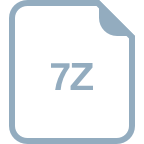
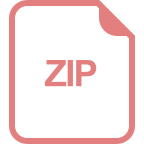
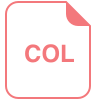
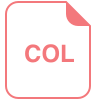
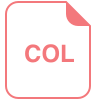
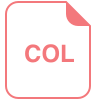
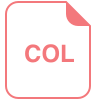
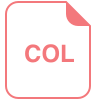