Handling Parameters in HTTP Requests in LabVIEW
发布时间: 2024-09-14 21:27:41 阅读量: 26 订阅数: 31 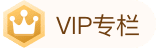
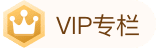
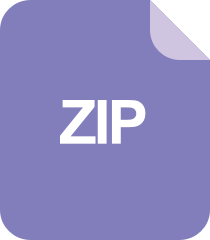
Requests-HTTP库.zip
# 1. Introduction
## 1.1 What is LabVIEW
LabVIEW is a popular graphical programming tool widely used in areas such as engineering control, testing, and measurement. Its intuitive graphical programming interface allows engineers to quickly implement complex functions by dragging and connecting icons.
## 1.2 What Are Parameters in HTTP Requests
In HTTP requests, parameters refer to key-value pairs passed through the URL using the GET method or data passed through the POST method in the request body. These parameters are used to pass information to the server, such as user authentication and data queries.
## 1.3 Why Is It Necessary to Handle Parameters in HTTP Requests
Handling parameters in HTTP requests is one of the common tasks in Web development. By processing parameters, you can achieve data transfer, user authentication, page redirection, and other functions. LabVIEW, as a powerful engineering development tool, can help engineers easily implement parameter processing functionality.
# 2. Building an HTTP Server
Building a simple HTTP server in LabVIEW is very convenient and can be easily accomplished using the Web services module provided by LabVIEW. Below are the detailed steps and code examples for building an HTTP server:
### 2.1 Building a Simple HTTP Server in LabVIEW
First, open the LabVIEW software and create a new VI. Then, drag and drop the Web services module into the VI from the LabVIEW function library, and configure the appropriate port number and route.
```labview
LabVIEW Web services module configuration example:
- Port number: 8080
- Route: /api
```
### 2.2 Receiving HTTP Requests
Next, add an HTTP request event handler to the Web services module to receive and process HTTP requests from clients in real-time.
```labview
HTTP request event handler example:
- Trigger this event when a GET or POST request is received
- Get the request content and process it in the event handler
```
### 2.3 Understanding Parameters in HTTP Requests
Parameters in HTTP requests are usually contained in the URL (GET requests) or the request body (POST requests) and need to be parsed to obtain the data content passed by the client.
By following these steps, you can set up a simple HTTP server in LabVIEW, receive HTTP requests in real-time, and prepare for subsequent parameter processing.
# 3. Parsing HTTP Parameters
Parsing parameters is a crucial part of handling HTTP requests. LabVIEW, as a graphical programming tool, can help engineers easily parse parameters in HTTP requests. This chapter will introduce how to parse HTTP parameters in LabVIEW, including methods for parsing parameters from GET and POST requests, and how to use LabVIEW's built-in functions to handle these parameters. Next, we will discuss the parsing and processing of parameters in detail.
#### 3.1 Parsing Parameters from GET Requests
Parameters in GET requests are passed through the URL, usually in the format of `***`. In LabVIEW, we can use the GET method in the HTTP methods to obtain parameters from the URL and then parse these parameters using string processing functions.
```labview
// LabVIEW code example: parsing parameters from a GET request
URL := "***";
[param1, value1, param2, value2] := ParseGETParameters(URL);
// The ParseGETParameters method is a custom implementation for parsing parameters from a GET request
```
Code Summary: By using the custom ParseGETParameters method, we can obtain the parameters from the GET request in the URL and parse out the corresponding parameter names and values.
Result Explanation: With the above code, we can successfully parse the parameters from the GET request, achieving the extraction and processing of GET request parameters.
#### 3.2 Parsing Parameters from POST Requests
Parameters in POST requests are sent through the request body, usually in the format of form data or raw data. In LabVIEW, we can obtain the parameters in the request body through the POST method in the HTTP methods and then parse and process them.
```labview
// LabVIEW code example: parsing parameters from a POST request
requestBody := GetPOSTBodyData(); // Get the request body data of the POST request
[param1, value1, param2, value2] := ParsePOSTParameters(requestBody)
```
0
0
相关推荐







