Methods for Processing HTTP Responses in LabVIEW
发布时间: 2024-09-14 21:26:00 阅读量: 21 订阅数: 20 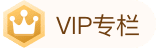
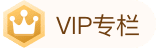
## 1. Understanding the Basics of HTTP Responses
HTTP (Hypertext Transfer Protocol) is an application layer protocol used for transmitting hypertext data, such as HTML, XML, images, videos, etc. It is one of the foundational elements of the internet. Understanding the HTTP protocol is crucial for anyone involved in Web development or network communications.
### 1.1 Overview of the HTTP Protocol
The HTTP protocol is a set of rules for communication between clients and servers. It defines how clients send requests and how servers respond to those requests. Typically, clients send HTTP requests to retrieve resources from the server, and the server responds with an HTTP response to provide the requested resource.
### 1.2 Basic Structure of HTTP Requests and Responses
An HTTP request consists of a request line, request headers, an empty line, and a request body. An HTTP response consists of a status line, response headers, an empty line, and a response body. The request line or status line contains basic information about the request or response, the request headers or response headers contain additional metadata, and the request body or response body contains the actual data.
### 1.3 Meanings and Classifications of HTTP Status Codes
HTTP status codes are numerical codes that represent the server's response status to a client'***mon HTTP status codes include: 2xx for success, 3xx for redirection, 4xx for client errors, and 5xx for server errors. Understanding the meanings of different status codes is essential for handling HTTP responses effectively.
## 2. Sending HTTP Requests with LabVIEW
In this chapter, we will introduce how to send HTTP requests in LabVIEW, including configuring HTTP requests, writing LabVIEW code to send HTTP requests, and handling potential problems encountered. Let's delve into it together.
### 2.1 Configuring HTTP Requests in LabVIEW
To configure an HTTP request in LabVIEW, follow these steps:
1. Open LabVIEW and create a new VI.
2. Right-click on a blank space in the Block Diagram, choose Functions Palette, and then select Internet -> TCP/IP functions.
3. Choose the HTTP Client VI and drag it into the Block Diagram.
4. Configure the input parameters of the HTTP Client VI, including the URL, request method (GET, POST, etc.), and request headers.
5. Connect the output of the HTTP Client VI to an appropriate data processing module.
### 2.2 Writing LabVIEW Code to Send HTTP Requests
Here is a simple LabVIEW code example for sending an HTTP GET request:
```LabVIEW
// Create an instance of the HTTP Client
client = New HTTP Client;
// Configure the request URL
Set URL(client, "***");
// Set the request method to GET
Set Method(client, "GET");
// Send the HTTP request
response = Send Request(client);
```
### 2.3 Handling Potential Problems in HTTP Requests
While processing HTTP requests, various problems may occur, such as network connection failure, request timeouts, or server errors. In LabVIEW, these issues can be captured and handled by using an Error Handler, ensuring program stability and reliability.
With these steps, you can successfully send HTTP requests in LabVIEW and handle any potential issues. In the next chapter, we will continue to explore how to analyze the structure of HTTP responses.
## 3. Analyzing the Structure of HTTP Responses
In this chapter, we will delve into how to analyze the structure of HTTP responses, including parsing response header information, extracting data from the response body, and dealing with different types of HTTP responses. Let's explore these details together.
#### 3.1 Parsing HTTP Response Header Information
The header information of an HTTP response provides various metadata about the response, such as the response status code and header fields. When handling HTTP responses, you need to parse this header information for further processing.
```python
import requests
response = requests.get('***')
print(response.status_code) # Outputs the status code
print(response.headers) # Outputs the header information
```
**Code Explanation:**
- Use the Python requests library to send an HTTP GET request and receive a response.
- The `status_code` attribute is used to get the HTTP response status code.
- The `headers` attribute is used to get the HTTP response header information.
**Code Summary:*
0
0
相关推荐
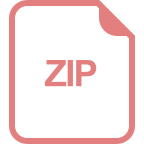
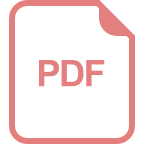
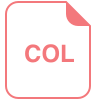
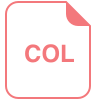
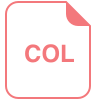
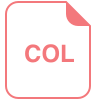
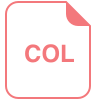
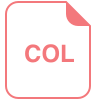