【线性表问题诊断与解决】:Python中的错误管理与日志记录
发布时间: 2024-09-12 09:11:38 阅读量: 38 订阅数: 23 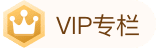
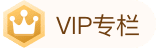
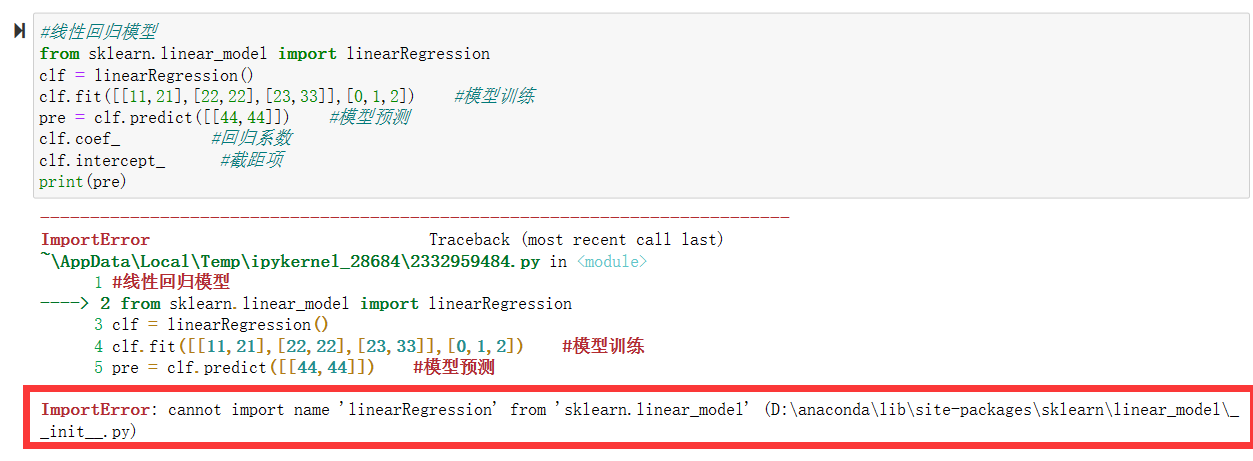
# 1. Python线性表概述与常见问题
## 1.1 Python线性表的基本概念
Python中的线性表是一种常见的数据结构,以顺序的方式存储数据,包括列表(list)、元组(tuple)、字符串(str)和字节序列(bytes)等。线性表的基本操作包括访问、添加、删除和修改元素,其直观的特性使得开发者容易上手。
## 1.2 线性表的使用场景
线性表广泛应用于数据的临时存储、简单的数据查询和处理场景中,如排序、搜索等。线性表的实现虽然简单,但在大数据集的处理中,性能和资源管理成为需要关注的重点。
## 1.3 线性表的常见问题
线性表操作中常见的问题包括索引越界、数据类型不匹配、内存溢出等。避免这些问题通常需要对数据操作的边界条件进行细致的检查,并合理分配内存资源。
通过理解线性表的基础知识和常见问题,开发者可以更加有效地利用Python处理数据,并为错误管理和日志记录打下坚实的基础。
# 2. Python线性表的错误管理策略
### 2.1 理解Python中的错误和异常
#### 2.1.1 错误与异常的基本概念
在Python编程中,错误和异常是程序运行时可能遇到的问题,它们的处理是保证程序稳定运行的关键。一个错误通常指的是在程序执行之前就可以被发现的问题,比如语法错误。而异常则是在程序运行时发生的,通常是无法在编译时期预测的问题。
Python中的异常是对象,它们代表了在程序执行过程中发生的不正常情况。当Python遇到异常时,如果没有适当的处理,它会停止执行程序,并显示异常信息。举个例子:
```python
def divide(x, y):
return x / y
result = divide(10, 0)
print(result)
```
上述代码中,`10 / 0` 将引发一个 `ZeroDivisionError` 异常,因为除数不能为零。
#### 2.1.2 异常处理的重要性
异常处理是让程序能够继续运行的一种机制,即便在遇到了某些错误的情况下。Python中的异常处理机制主要通过`try-except`语句来实现。使用异常处理机制可以带来以下几个好处:
- 防止程序异常退出。
- 允许程序在遇到错误时优雅地进行恢复。
- 提供了一种通过日志记录错误详情的方式,便于后续分析和调试。
正确的异常处理可以提高代码的健壮性,提高用户体验,并确保程序的可靠性和稳定性。
### 2.2 异常处理机制的实现
#### 2.2.1 try-except语句的工作原理
`try-except`是Python处理异常的基石。基本的`try-except`语句结构如下:
```python
try:
# 代码块,可能出现异常的地方
pass
except SomeException as e:
# 异常处理代码
print(f"Caught an exception: {e}")
```
在`try`块中,如果执行代码时发生了异常,Python会暂停`try`块的执行,查找与异常类型匹配的`except`块,并执行该`except`块中的代码。如果没有找到匹配的`except`块,异常会被向上抛出到调用栈中,直到被处理或导致程序退出。
#### 2.2.2 多个异常的捕获和处理
在`try-except`语句中,可以指定多个`except`子句来捕获和处理多种不同的异常:
```python
try:
# 可能抛出不同异常的代码
pass
except ZeroDivisionError as e:
print(f"Cannot divide by zero: {e}")
except ValueError as e:
print(f"Invalid value: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
```
这使得代码可以对不同的错误作出不同的反应,增强了程序的灵活性和可控性。
#### 2.2.3 自定义异常类
Python允许开发人员定义自己的异常类,通过继承内置的`Exception`类来实现。这在需要区分异常类型,或者当内置的异常类型不能明确地描述错误时非常有用:
```python
class MyError(Exception):
"""自定义异常类"""
def perform_action():
raise MyError("Something went wrong")
try:
perform_action()
except MyError as e:
print(f"Caught a custom error: {e}")
```
自定义异常使得异常处理更加具体化和模块化,有助于维护和扩展代码。
### 2.3 优化异常处理的实践技巧
#### 2.3.1 异常日志记录的最佳实践
为了确保异常信息的有效性,记录异常日志是一种常见的实践。Python的`logging`模块可以用来记录异常信息:
```python
import logging
try:
# 可能抛出异常的代码
pass
except Exception as e:
logging.error("Exception occurred", exc_info=True)
```
使用`logging`模块记录异常时,`exc_info=True`参数会自动记录当前异常的信息。这样的记录方式不但详细而且不侵入业务代码逻辑。
#### 2.3.2 避免过度异常处理陷阱
在实际的项目开发中,过度的异常处理可能会导致代码难以阅读和维护。因此,开发者需要避免捕获异常的"黑洞"效应。以下是一些避免过度异常处理的建议:
- 只捕获并处理你预期可以处理的异常。
- 避免使用空的`except`子句,这样做会隐藏一些难以预料的错误。
- 尽可能在合适的层级处理异常,避免在函数中捕获异常后隐藏异常的根源。
通过这些方法,可以在确保程序鲁棒性的同时,也保证了代码的清晰性和可维护性。
通过本章节的介绍,我们了解了错误和异常的基本概念,异常处理的重要性,以及如何使用`try-except`语句实现异常处理机制,并在实践中应用优化技巧。接下来的章节中,我们将探讨Python线性表日志记录方法,以进一步加强错误管理的能力。
# 3. Python线性表日志记录方法
日志记录是软件开发中不可或缺的部分,它帮助开发者追踪程序运行过程中的行为,从而能够更好地进行错误诊断和系统分析。本章节将深入介绍Python中日志模块的基础知识,探讨如何配置和优化高级日志系统,以及分析和利用日志数据的技巧。
## 3.1 日志模块基础
### 3.1.1 logging模块概述
Python的`logging`模块是用于记录日志的标准库,它提供了强大的日志记录功能。开发者可以通过简单的API调用,灵活地配置日志记录的级别、格式以及输出目标等。`logging`模块的灵活性意味着可以满足从简单的脚本到复杂应用系统的日志记录需求。
### 3.1.2 基本的日志记录方法
在`logging`模块中,日志记录方法非常直观。开发者可以使用不同级别的函数,如`logging.debug()`, `***()`, `logging.warning()`, `logging.error()`, 和 `logging.critical()`,来记录不同严重性的日志信息。以下是一个简单的示例:
```python
import logging
logging.b
```
0
0
相关推荐
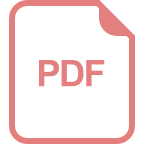






